For beginning Java, two things 1) I got this error from my code and how and where can I fix it: Main.java:133: error: reached end of file while parsing } ^ 1 error 2) I was told my code is too long and needs to be separate files. How can I fix this into separate Java files? 3) I need to Draw the UML diagram using MS Word or PowerPoint for the classes and implement them. My original assignment is attached. Here's my code: import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.time.LocalDate; class Person { private String name; private String address; private String phoneNumber; private String emailAddress; public Person(String name, String address, String phoneNumber, String emailAddress) { this.name = name; this.address = address; this.phoneNumber = phoneNumber; this.emailAddress = emailAddress; } @Override public String toString() { return "Person: " + name + "\nAddress: " + address + "\nPhone Number: " + phoneNumber + "\nEmail Address: " + emailAddress; } } class Student extends Person { private String classStatus; public Student(String name, String address, String phoneNumber, String emailAddress, String classStatus) { super(name, address, phoneNumber, emailAddress); this.classStatus = classStatus; } @Override public String toString() { return super.toString() + "\nClass: Student" + "\nClass Status: " + classStatus; } } class Employee extends Person { private double salary; private LocalDate dateHired; public Employee(String name, String address, String phoneNumber, String emailAddress, double salary, LocalDate dateHired) { super(name, address, phoneNumber, emailAddress); this.salary = salary; this.dateHired = dateHired; } @Override public String toString() { return super.toString() + "\nClass: Employee" + "\nSalary: " + salary + "\nDate Hired: " + dateHired; } } class Faculty extends Employee { private String officeHours; private String discipline; public Faculty(String name, String address, String phoneNumber, String emailAddress, double salary, LocalDate dateHired, String officeHours, String discipline) { super(name, address, phoneNumber, emailAddress, salary, dateHired); this.officeHours = officeHours; this.discipline = discipline; } @Override public String toString() { return super.toString() + "\nClass: Faculty" + "\nOffice Hours: " + officeHours + "\nDiscipline: " + discipline; } } class Staff extends Employee { private String title; public Staff(String name, String address, String phoneNumber, String emailAddress, double salary, LocalDate dateHired, String title) { super(name, address, phoneNumber, emailAddress, salary, dateHired); this.title = title; } @Override public String toString() { return super.toString() + "\nClass: Satff" + "\nTitle: " + title; } } // test program public class Main { public static void main(String[] args) { // Read data from a text file try (BufferedReader br = new BufferedReader(new FileReader("data.txt"))) { String line; while ((line = br.readLine()) != null) { String[] data = line.split(","); switch (data[0]) { case "Person": if (data.length >= 5) { Person person = new Person(data[1], data[2], data[3], data[4]); System.out.println(person); } break; case "Student": if (data.length >= 6) { Student student = new Student(data[1], data[2], data[3], data[4], data[5]); System.out.println(student); } break; case "Employee": if (data.length >= 6) { LocalDate dateHired = LocalDate.parse(data[5]); Employee employee = new Employee(data[1], data[2], data[3], data[4], Double.parseDouble(data[5]), dateHired); System.out.println(employee); } break; case "Faculty": if (data.length >= 8) { LocalDate facultyDateHired = LocalDate.parse(data[6]); Faculty faculty = new Faculty(data[1], data[2], data[3], data[4], Double.parseDouble(data[5]), facultyDateHired, data[6], data[7]); System.out.println(faculty); } break; case "Staff": if (data.length >= 7) { LocalDate staffDateHired = LocalDate.parse(data[5]); Staff staff = new Staff(data[1], data[2], data[3], data[4], Double.parseDouble(data[5]), staffDateHired, data[6]); System.out.println(staff); } break; default: System.out.println("Invalid entry"); break; }
For beginning Java, two things
1) I got this error from my code and how and where can I fix it:
Main.java:133: error: reached end of file while parsing } ^ 1 error
2) I was told my code is too long and needs to be separate files. How can I fix this into separate Java files?
3) I need to Draw the UML diagram using MS Word or PowerPoint for the classes and implement them.
My original assignment is attached.
Here's my code:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.time.LocalDate;
class Person {
private String name;
private String address;
private String phoneNumber;
private String emailAddress;
public Person(String name, String address, String phoneNumber, String emailAddress) {
this.name = name;
this.address = address;
this.phoneNumber = phoneNumber;
this.emailAddress = emailAddress;
}
@Override
public String toString() {
return "Person: " + name + "\nAddress: " + address + "\nPhone Number: " + phoneNumber +
"\nEmail Address: " + emailAddress;
}
}
class Student extends Person {
private String classStatus;
public Student(String name, String address, String phoneNumber, String emailAddress, String classStatus) {
super(name, address, phoneNumber, emailAddress);
this.classStatus = classStatus;
}
@Override
public String toString() {
return super.toString() + "\nClass: Student" + "\nClass Status: " + classStatus;
}
}
class Employee extends Person {
private double salary;
private LocalDate dateHired;
public Employee(String name, String address, String phoneNumber, String emailAddress, double salary, LocalDate dateHired) {
super(name, address, phoneNumber, emailAddress);
this.salary = salary;
this.dateHired = dateHired;
}
@Override
public String toString() {
return super.toString() + "\nClass: Employee" + "\nSalary: " + salary + "\nDate Hired: " + dateHired;
}
}
class Faculty extends Employee {
private String officeHours;
private String discipline;
public Faculty(String name, String address, String phoneNumber, String emailAddress, double salary, LocalDate dateHired,
String officeHours, String discipline) {
super(name, address, phoneNumber, emailAddress, salary, dateHired);
this.officeHours = officeHours;
this.discipline = discipline;
}
@Override
public String toString() {
return super.toString() + "\nClass: Faculty" + "\nOffice Hours: " + officeHours + "\nDiscipline: " + discipline;
}
}
class Staff extends Employee {
private String title;
public Staff(String name, String address, String phoneNumber, String emailAddress, double salary, LocalDate dateHired,
String title) {
super(name, address, phoneNumber, emailAddress, salary, dateHired);
this.title = title;
}
@Override
public String toString() {
return super.toString() + "\nClass: Satff" + "\nTitle: " + title;
}
}
// test
public class Main {
public static void main(String[] args) {
// Read data from a text file
try (BufferedReader br = new BufferedReader(new FileReader("data.txt"))) {
String line;
while ((line = br.readLine()) != null) {
String[] data = line.split(",");
switch (data[0]) {
case "Person":
if (data.length >= 5) {
Person person = new Person(data[1], data[2], data[3], data[4]);
System.out.println(person);
}
break;
case "Student":
if (data.length >= 6) {
Student student = new Student(data[1], data[2], data[3], data[4], data[5]);
System.out.println(student);
}
break;
case "Employee":
if (data.length >= 6) {
LocalDate dateHired = LocalDate.parse(data[5]);
Employee employee = new Employee(data[1], data[2], data[3], data[4], Double.parseDouble(data[5]), dateHired);
System.out.println(employee);
}
break;
case "Faculty":
if (data.length >= 8) {
LocalDate facultyDateHired = LocalDate.parse(data[6]);
Faculty faculty = new Faculty(data[1], data[2], data[3], data[4], Double.parseDouble(data[5]), facultyDateHired, data[6], data[7]);
System.out.println(faculty);
}
break;
case "Staff":
if (data.length >= 7) {
LocalDate staffDateHired = LocalDate.parse(data[5]);
Staff staff = new Staff(data[1], data[2], data[3], data[4], Double.parseDouble(data[5]), staffDateHired, data[6]);
System.out.println(staff);
}
break;
default:
System.out.println("Invalid entry");
break;
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

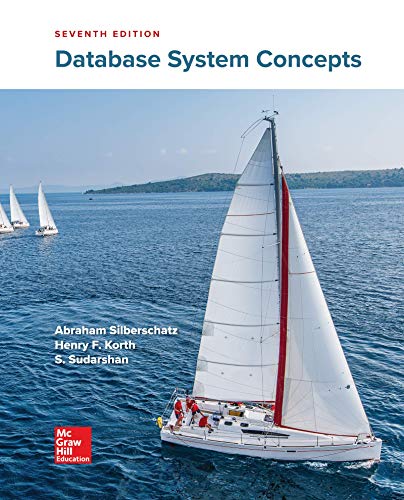
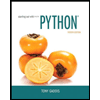
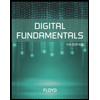
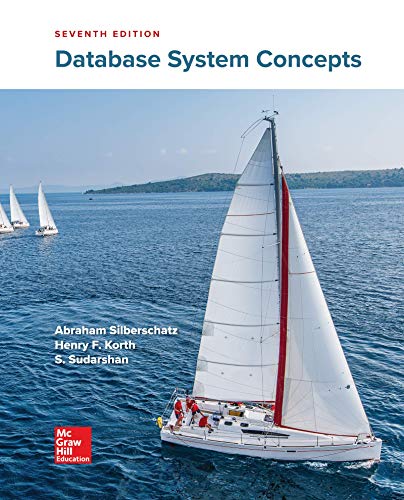
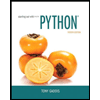
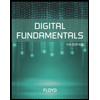
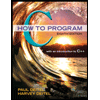
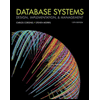
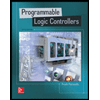