In Java, I need help completing these two classes. // ************************************************************ // Student.java // // Define a student class that stores name, score on test 1, and // score on test 2. Methods prompt for and read in grades, // compute the average, and return a string containing student's info. // ************************************************************ import java.util.Scanner; public class Student { //declare instance data // --------------------------------------------- //constructor // --------------------------------------------- public Student(String studentName) { //add body of constructor } // --------------------------------------------- //inputGrades: prompt for and read in student's grades for test1 and test2. //Use name in prompts, e.g., "Enter's Joe's score for test1". // --------------------------------------------- public void inputGrades() { //add body of inputGrades } // --------------------------------------------- //getAverage: compute and return the student's test average // --------------------------------------------- //add header for getAverage { //add body of getAverage } // --------------------------------------------- //getName: get the student's name // --------------------------------------------- //add header for getName { //add body of getName } } // ************************************************************ // Grades.java // // Use Student class to get test grades for two students // and compute averages // // ************************************************************ public class Grades { public static void main(String[] args) { Student student1 = new Student("Mary"); //create student2, "Mike" //input grades for Mary //print average for Mary System.out.println(); //input grades for Mike //print average for Mike } }
In Java, I need help completing these two classes.
// ************************************************************
// Student.java
//
// Define a student class that stores name, score on test 1, and
// score on test 2. Methods prompt for and read in grades,
// compute the average, and return a string containing student's info.
// ************************************************************
import java.util.Scanner;
public class Student
{
//declare instance data
// ---------------------------------------------
//constructor
// ---------------------------------------------
public Student(String studentName)
{
//add body of constructor
}
// ---------------------------------------------
//inputGrades: prompt for and read in student's grades for test1 and test2.
//Use name in prompts, e.g., "Enter's Joe's score for test1".
// ---------------------------------------------
public void inputGrades()
{
//add body of inputGrades
}
// ---------------------------------------------
//getAverage: compute and return the student's test average
// ---------------------------------------------
//add header for getAverage
{
//add body of getAverage
}
// ---------------------------------------------
//getName: get the student's name
// ---------------------------------------------
//add header for getName
{
//add body of getName
}
}
// ************************************************************
// Grades.java
//
// Use Student class to get test grades for two students
// and compute averages
//
// ************************************************************
public class Grades
{
public static void main(String[] args)
{
Student student1 = new Student("Mary");
//create student2, "Mike"
//input grades for Mary
//print average for Mary
System.out.println();
//input grades for Mike
//print average for Mike
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

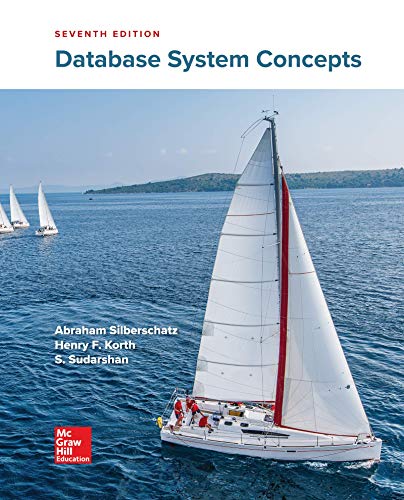
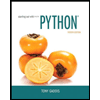
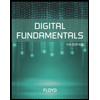
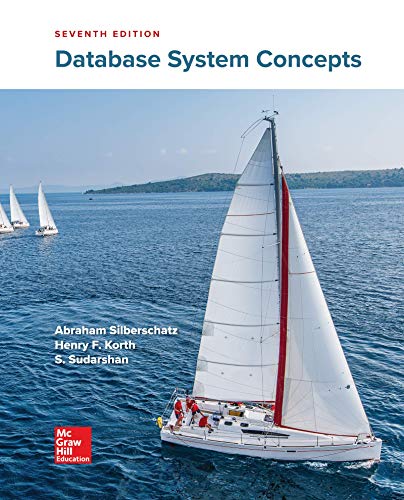
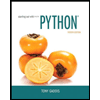
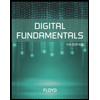
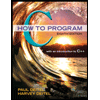
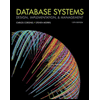
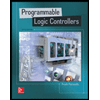