PLease briefly explain me about this code. How does it works? import React from 'react'; class ContactForm extends React.Component { constructor(props) { super(props); this.state = { name: '', storageType: '', maxItem: '', resourceName: '', maxResource: '', currentResource: '' }; this.handleNameChange = this.handleNameChange.bind(this); this.handleStorageTypeChange = this.handleStorageTypeChange.bind(this); this.handleMaxItemChange = this.handleMaxItemChange.bind(this); this.handleResourceNameChange = this.handleResourceNameChange.bind(this); this.handleMaxResourceChange = this.handleMaxResourceChange.bind(this); this.handleCurrentResourceChange = this.handleCurrentResourceChange.bind(this); this.handleSubmit = this.handleSubmit.bind(this); } handleNameChange(event) { const target = event.target; const value = target.type === 'checkbox' ? target.checked : target.value; const name = target.name; this.setState({ name: value }) console.log('Change detected. State updated' + name + ' = ' + value); } handleStorageTypeChange(event) { const target = event.target; const value = target.type === 'checkbox' ? target.checked : target.value; this.setState({ storageType: value }) } handleMaxItemChange(event) { const target = event.target; const value = target.type === 'checkbox' ? target.checked : target.value; this.setState({ maxItem: value }) } handleResourceNameChange(event) { this.setState({ resourceName: event.target.value }) } handleMaxResourceChange(event) { this.setState({ maxResource: event.target.value }) } handleCurrentResourceChange(event) { this.setState({ currentResource: event.target.value }) } handleSubmit(event) { alert('A form was submitted: ' + this.state.name + ' // ' + this.state.email); event.preventDefault(); } render() { return ( Inventory Name Storage Type Max Item Capacity Resources Resource Name Max Number of Resources Current Number of Resources ) } } class MainTitle extends React.Component { render() { return ( Add Inventory ) } } export default class App extends React.Component { render() { return ( ) } }
PLease briefly explain me about this code. How does it works?
import React from 'react';
class ContactForm extends React.Component {
constructor(props) {
super(props);
this.state = {
name: '',
storageType: '',
maxItem: '',
resourceName: '',
maxResource: '',
currentResource: ''
};
this.handleNameChange = this.handleNameChange.bind(this);
this.handleStorageTypeChange = this.handleStorageTypeChange.bind(this);
this.handleMaxItemChange = this.handleMaxItemChange.bind(this);
this.handleResourceNameChange = this.handleResourceNameChange.bind(this);
this.handleMaxResourceChange = this.handleMaxResourceChange.bind(this);
this.handleCurrentResourceChange = this.handleCurrentResourceChange.bind(this);
this.handleSubmit = this.handleSubmit.bind(this);
}
handleNameChange(event) {
const target = event.target;
const value = target.type === 'checkbox' ? target.checked : target.value;
const name = target.name;
this.setState({
name: value
})
console.log('Change detected. State updated' + name + ' = ' + value);
}
handleStorageTypeChange(event) {
const target = event.target;
const value = target.type === 'checkbox' ? target.checked : target.value;
this.setState({
storageType: value
})
}
handleMaxItemChange(event) {
const target = event.target;
const value = target.type === 'checkbox' ? target.checked : target.value;
this.setState({
maxItem: value
})
}
handleResourceNameChange(event) {
this.setState({
resourceName: event.target.value
})
}
handleMaxResourceChange(event) {
this.setState({
maxResource: event.target.value
})
}
handleCurrentResourceChange(event) {
this.setState({
currentResource: event.target.value
})
}
handleSubmit(event) {
alert('A form was submitted: ' + this.state.name + ' // ' + this.state.email);
event.preventDefault();
}
render() {
return (
<div>
<form onSubmit={this.handleSubmit}>
<div className="form-group">
<h1> Inventory </h1>
<label for="nameInput">Name</label>
<input type="text" name="name" value={this.state.name} onChange={this.handleNameChange}
className="form-control" id="nameInput" placeholder="Name" />
</div>
<div className="form-group">
<label for="storageType">Storage Type</label>
<input name="storage" type="text" value={this.state.storageType} onChange={this.handleStorageTypeChange}
className="form-control" id="storageType" placeholder="Storage Type" />
</div>
<div className="form-group">
<label for="nameInput">Max Item Capacity</label>
<input type="text" name="maxItem" value={this.state.maxItem} onChange={this.handleMaxItemChange}
className="form-control" id="nameInput" placeholder="Max Item Capacity" />
</div>
<div className="form-group">
<h1> Resources </h1>
<label for="nameInput">Resource Name</label>
<input type="text" name="resourceName" value={this.state.resourceName} onChange={this.handleResourceNameChange}
className="form-control" id="nameInput" placeholder="Resource Name" />
</div>
<div className="form-group">
<label for="nameInput">Max Number of Resources</label>
<input name="maxResource" value={this.state.maxResource} onChange={this.handleMaxResourceChange}
className="form-control" id="nameInput" placeholder="Max Number of Resources" />
</div>
<div className="form-group">
<label for="nameInput">Current Number of Resources</label>
<input type="text" name="currentResource" value={this.state.currentResource} onChange={this.handleCurrentResourceChange}
className="form-control" id="nameInput" placeholder="Current number of Resources" />
</div>
</form>
</div>
)
}
}
class MainTitle extends React.Component {
render() {
return (
<h1> Add Inventory </h1>
)
}
}
export default class App extends React.Component {
render() {
return (
<div>
<MainTitle />
<ContactForm />
</div>
)
}
}

Step by step
Solved in 4 steps with 2 images

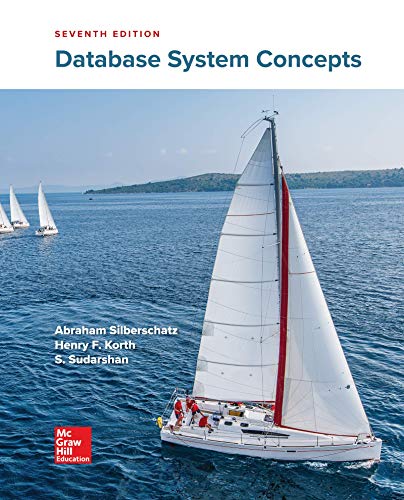
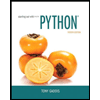
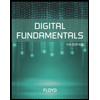
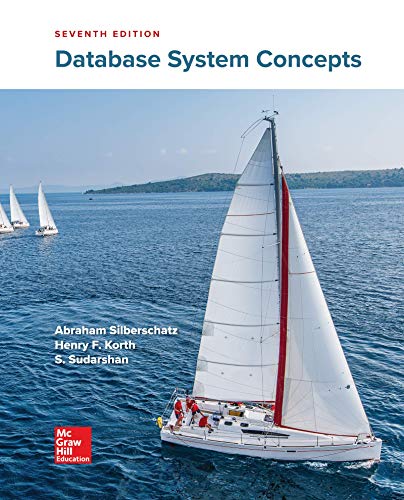
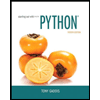
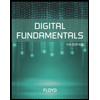
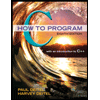
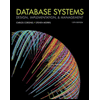
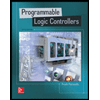