I have inlcluded my code and cannot get the y or no portion to add at end of the loop and trying to understand it. Thank you ! # Define a function to perform safe division def safe_division(num1, num2): try: result = num1 / num2 return result except ValueError: return "Invalid input. Please enter valid numbers." except ZeroDivisionError: return "Division by zero is not allowed." # Create a while loop to allow the user to continue trying while True: try: # Prompt the user for the first number input_num1 = input("Enter the first number: ") # Convert the user input to a floating-point number num1 = float(input_num1) # Prompt the user for the second number input_num2 = input("Enter the second number: ") # Convert the user input to a floating-point number num2 = float(input_num2) # Call the safe_division function and print the result result = safe_division(num1, num2) print(f"Result: {result}") except ValueError: print("Error - Both inputs must be numbers.") except ZeroDivisionError: print("Error - Cannot divide by zero.") # Add to try again print("Thank you for using Safe Division Program!")
I have inlcluded my code and cannot get the y or no portion to add at end of the loop and trying to understand it.
Thank you !
# Define a function to perform safe division
def safe_division(num1, num2):
try:
result = num1 / num2
return result
except ValueError:
return "Invalid input. Please enter valid numbers."
except ZeroDivisionError:
return "Division by zero is not allowed."
# Create a while loop to allow the user to continue trying
while True:
try:
# Prompt the user for the first number
input_num1 = input("Enter the first number: ")
# Convert the user input to a floating-point number
num1 = float(input_num1)
# Prompt the user for the second number
input_num2 = input("Enter the second number: ")
# Convert the user input to a floating-point number
num2 = float(input_num2)
# Call the safe_division function and print the result
result = safe_division(num1, num2)
print(f"Result: {result}")
except ValueError:
print("Error - Both inputs must be numbers.")
except ZeroDivisionError:
print("Error - Cannot divide by zero.")
# Add to try again
print("Thank you for using Safe Division Program!")


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

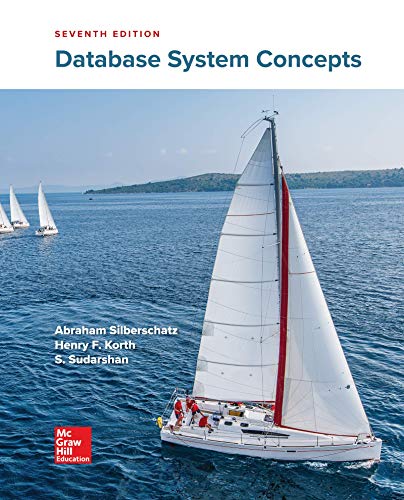
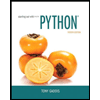
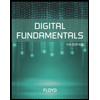
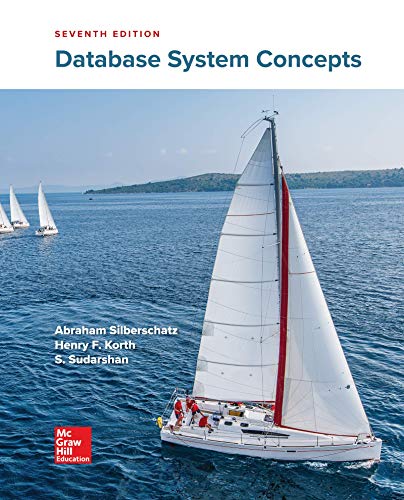
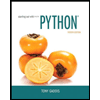
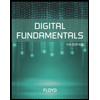
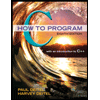
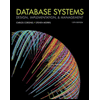
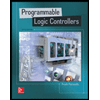