Please fill in the blanks. /* This function asks for 2 strings from the user and asks the user to choose what they want to do with them. Inputs: 2 strings, and a character for choice. Output: execute that choice. Choices can be: 'E'/'e': call checkEqual() function 'C'/'c': call concatStrings() function Everything else: invalid and exit */ #include<__1__> //library to use printf and scanf #include<__2__> //library to use boolean #include<__3__> //library to use exit(0) #define len 1000 /*This function just concatenates 2 strings using a */ __4__ concatStrings(__5__ w1[], __6__ w2[], __7__ w3[]) { int w3_idx = 0; //index for w3 //Copy word1 w1 over to the combined string for(int i = 0; __8__ != __9__; i++) //loop runs as long as string is not done { __10__[__11__] = __12__[__13__]; //copy a character from w1 to w3 w3_idx++; //update index for w3 } //Add in a space between w1 and next word for our w3 string __14__[__15__] = ' '; __16__; //update the index for w3, type everything without spaces //Copy over word2 w2 for(int i = 0; __17__ != __18__; i++) //loop runs as long as string is not done { __19__[__20__] = __21__[__22__]; //copy a character from w2 to w3 w3_idx++;//update index for w3 } //Reach here means the copy process is done. w3 is done. // Need to do something here to let the program know it's done for later use __23__[__24__] = __25__; } /*This function returns the length of a string*/ __26__ getLen(__27__ w[]) { int size = 0; for(int i = 0; __28__ != __29__; i++) //no spaces, loop runs as long as string is not done __30__++; //update the length __31__ __32__; //return the length } /*This function checks for equality, and return a boolean for true/false*/ __33__ checkEqual(__34__ word1[], __35__ word2[]) { //Call the function to get the length for each word int len1 = __36__(__37__); //word1's len int len2 = __38__(__39__); //word2's len //stop right away if the two words have different length if(len1 __40__ __41__) __42__ __43__; //Reach here means they have same length //Loop until the first (or second since len is the same) reaches the end //Check if each character is the same. //If they are not, return false right away for(int i = 0; word1[i] __44__ __45__; i++) //if in any case the characters aren't the same, return right away //no spaces in the blank if(__46__ __47__ word2[i]) __48__ __49__; //Reach here means the words are done, and they are the same. Return return __50__; } /*executeChoice is a function that uses switch case to perform user's choice 3 cases: 'E'/'e': call checkEqual() function 'C'/'c': call concatStrings() function Everything else: invalid and exit note: you have to write checkEqual() and concatStrings() functions*/ void executeChoice(char choice, char w1[], char w2[], char combined[]) { bool isSame; switch(choice) { case 'E': case 'e': isSame = checkEqual(w1, w2); (isSame == 1) ? printf("They are the same.\n") : printf("They are different.\n"); break; case 'C': case 'c': concatStrings(w1,w2, combined); printf("Result of the concatenation is: \n%s\n", combined); break; default: printf("Invalid. Bye.\n"); exit(0); } } int main() { //Create 3 strings (2 for 2 inputted words and 1 for the concat) // and 1 character for user's choice //len is defined on top for maximum length char word1[len], word2[len], combined[len], choice; //Get 2 words printf("Enter word1: "); scanf("%s", word1); printf("Enter word2: "); scanf("%s", word2); //Get user's choice printf("What do you want to do with these 2 strings?\n"); printf("Enter 'E' to check for equality or 'C' to combine into 1 string:"); scanf(" %c",&choice); //Call the function to execute the choices executeChoice(choice, word1, word2, combined); return 0; }
Please fill in the blanks.
/* This function asks for 2 strings from the user and asks the user to choose what they want to do with them.
Inputs: 2 strings, and a character for choice.
Output: execute that choice.
Choices can be: 'E'/'e': call checkEqual() function
'C'/'c': call concatStrings() function
Everything else: invalid and exit */
#include<__1__> //library to use printf and scanf
#include<__2__> //library to use boolean
#include<__3__> //library to use exit(0)
#define len 1000
/*This function just concatenates 2 strings using a */
__4__ concatStrings(__5__ w1[], __6__ w2[], __7__ w3[])
{
int w3_idx = 0; //index for w3
//Copy word1 w1 over to the combined string
for(int i = 0; __8__ != __9__; i++) //loop runs as long as string is not done
{
__10__[__11__] = __12__[__13__]; //copy a character from w1 to w3
w3_idx++; //update index for w3
}
//Add in a space between w1 and next word for our w3 string
__14__[__15__] = ' ';
__16__; //update the index for w3, type everything without spaces
//Copy over word2 w2
for(int i = 0; __17__ != __18__; i++) //loop runs as long as string is not done
{
__19__[__20__] = __21__[__22__]; //copy a character from w2 to w3
w3_idx++;//update index for w3
}
//Reach here means the copy process is done. w3 is done.
// Need to do something here to let the program know it's done for later use
__23__[__24__] = __25__;
}
/*This function returns the length of a string*/
__26__ getLen(__27__ w[])
{
int size = 0;
for(int i = 0; __28__ != __29__; i++) //no spaces, loop runs as long as string is not done
__30__++; //update the length
__31__ __32__; //return the length
}
/*This function checks for equality, and return a boolean for true/false*/
__33__ checkEqual(__34__ word1[], __35__ word2[])
{
//Call the function to get the length for each word
int len1 = __36__(__37__); //word1's len
int len2 = __38__(__39__); //word2's len
//stop right away if the two words have different length
if(len1 __40__ __41__) __42__ __43__;
//Reach here means they have same length
//Loop until the first (or second since len is the same) reaches the end
//Check if each character is the same.
//If they are not, return false right away
for(int i = 0; word1[i] __44__ __45__; i++)
//if in any case the characters aren't the same, return right away
//no spaces in the blank
if(__46__ __47__ word2[i]) __48__ __49__;
//Reach here means the words are done, and they are the same. Return
return __50__;
}
/*executeChoice is a function that uses switch case
to perform user's choice
3 cases: 'E'/'e': call checkEqual() function
'C'/'c': call concatStrings() function
Everything else: invalid and exit
note: you have to write checkEqual() and concatStrings() functions*/
void executeChoice(char choice, char w1[], char w2[], char combined[])
{
bool isSame;
switch(choice)
{
case 'E':
case 'e':
isSame = checkEqual(w1, w2);
(isSame == 1) ? printf("They are the same.\n") : printf("They are different.\n");
break;
case 'C':
case 'c':
concatStrings(w1,w2, combined);
printf("Result of the concatenation is: \n%s\n", combined);
break;
default:
printf("Invalid. Bye.\n");
exit(0);
}
}
int main()
{
//Create 3 strings (2 for 2 inputted words and 1 for the concat)
// and 1 character for user's choice
//len is defined on top for maximum length
char word1[len], word2[len], combined[len], choice;
//Get 2 words
printf("Enter word1: ");
scanf("%s", word1);
printf("Enter word2: ");
scanf("%s", word2);
//Get user's choice
printf("What do you want to do with these 2 strings?\n");
printf("Enter 'E' to check for equality or 'C' to combine into 1 string:");
scanf(" %c",&choice);
//Call the function to execute the choices
executeChoice(choice, word1, word2, combined);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

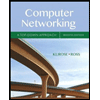
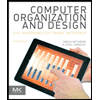
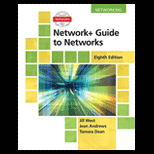
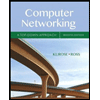
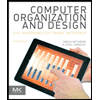
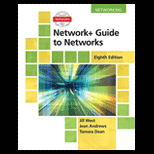
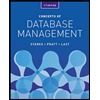
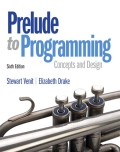
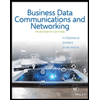