Is this a Phone Number? Learning Objectives In this lab, you will Create a function according to the specifications Use nested if/else statements Instructions Write a function, isPhoneNumber(digits), that checks to see if the input is a real phone number. This function should: Return True if 'digits' is type int and is 10 digits long Otherwise, returns False You can use the assert statements to test that your function behaves as expected. Hint: You can confirm 'digits' is an int with type(digits) == int Hint: To check the length of the number, you can convert it to a string first, then check the length. Alternatively, you can use integer division. # Define the function isPhoneNumber() below. The function should: # - Check that the input value has type 'int' and is 10 digits long. # - If this is true, return True # - If this is false, return False def isPhoneNumber(digits): pass # No print statement is needed for submission, but you can use a print statement to # confirm the output of isPhoneNumber(digits) is as expected with various inputs . if __name__ == "__main__": assert isPhoneNumber("1234567890") == False assert isPhoneNumber(1234567890) == True
Is this a Phone Number?
Learning Objectives
In this lab, you will
- Create a function according to the specifications
- Use nested if/else statements
Instructions
Write a function, isPhoneNumber(digits), that checks to see if the input is a real phone number. This function should:
- Return True if 'digits' is type int and is 10 digits long
- Otherwise, returns False
You can use the assert statements to test that your function behaves as expected.
Hint: You can confirm 'digits' is an int with type(digits) == int
Hint: To check the length of the number, you can convert it to a string first, then check the length. Alternatively, you can use integer division.
# Define the function isPhoneNumber() below. The function should:
# - Check that the input value has type 'int' and is 10 digits long.
# - If this is true, return True
# - If this is false, return False
def isPhoneNumber(digits):
pass
# No print statement is needed for submission, but you can use a print statement to
# confirm the output of isPhoneNumber(digits) is as expected with various inputs .
if __name__ == "__main__":
assert isPhoneNumber("1234567890") == False
assert isPhoneNumber(1234567890) == True


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

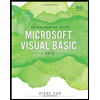
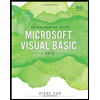