3 Functions with Value Parameters Use program BoxesWithFunction for Exercises 3-4. Program Boxes WithFunction is the shell of a program that prompts the user to enter an integer number. When completed, this number is read and passed to function Print as parameter numSigns. The function prints a box of dollar signs on the screen that is numSigns by (numSigns / 2) in dimension. For example, if numSigns is 10, the following box is printed on the screen: ssssssssss SSSsssssss Note the interior dimensions are (numSigns - 2) by (numSigns / 2- 2). // Program BoxesWithFunction prompts for the number of dollar signs // for the top of the box. // printed with dollar signs on the sides. That number/ 2-2 1ines are #include using namespace std; void Print (int numSigns); int main () int number; cout <« "Enter the number of dollar signs for the top; " « "press return."; cout <« "Enter a negative number to quit."<> number; while (number>=0) /* FILL IN call to Print */ cout << "Enter the number of dollar signs for the top; " « "press return."; cout « "Enter a negative number to quit."<> number; return 0; void Print (int numsigns) // FILL IN documentation. /* FILL IN code to print numSigns S's */ /* FILL IN code to print (numsigns / 2)-2 lines with /* S's lining up under the left-most and right-most /* $ ones on the top line. /* FILL IN code to print numsigns $'s Exercise 3. Fill in the missing code in function Print and the invoking statement in function main. Compile and run your program using 4, 10, and 7 as input data. Don't forget to fill in documentation for the function. Exercise 4: Rewrite your solution to Exercise 3 so that the symbol used as the border is also read as data and passed to function Print as a parameter. To make the symbol be a parameter requires the following changes: • Prompt for and read the symbol. • Add the symbol and its type (or just the type) to the parameter list of the function prototype. • Add the symbol and its type to the parameter list of the function definition. Use the parameter instead of 'S' in the body of the function definition. • Add the symbol to the argument list. Run the program three times using &, %, and A as symbols and 4, 10, and 7 as the number of symbols to use.
3 Functions with Value Parameters Use program BoxesWithFunction for Exercises 3-4. Program Boxes WithFunction is the shell of a program that prompts the user to enter an integer number. When completed, this number is read and passed to function Print as parameter numSigns. The function prints a box of dollar signs on the screen that is numSigns by (numSigns / 2) in dimension. For example, if numSigns is 10, the following box is printed on the screen: ssssssssss SSSsssssss Note the interior dimensions are (numSigns - 2) by (numSigns / 2- 2). // Program BoxesWithFunction prompts for the number of dollar signs // for the top of the box. // printed with dollar signs on the sides. That number/ 2-2 1ines are #include using namespace std; void Print (int numSigns); int main () int number; cout <« "Enter the number of dollar signs for the top; " « "press return."; cout <« "Enter a negative number to quit."<> number; while (number>=0) /* FILL IN call to Print */ cout << "Enter the number of dollar signs for the top; " « "press return."; cout « "Enter a negative number to quit."<> number; return 0; void Print (int numsigns) // FILL IN documentation. /* FILL IN code to print numSigns S's */ /* FILL IN code to print (numsigns / 2)-2 lines with /* S's lining up under the left-most and right-most /* $ ones on the top line. /* FILL IN code to print numsigns $'s Exercise 3. Fill in the missing code in function Print and the invoking statement in function main. Compile and run your program using 4, 10, and 7 as input data. Don't forget to fill in documentation for the function. Exercise 4: Rewrite your solution to Exercise 3 so that the symbol used as the border is also read as data and passed to function Print as a parameter. To make the symbol be a parameter requires the following changes: • Prompt for and read the symbol. • Add the symbol and its type (or just the type) to the parameter list of the function prototype. • Add the symbol and its type to the parameter list of the function definition. Use the parameter instead of 'S' in the body of the function definition. • Add the symbol to the argument list. Run the program three times using &, %, and A as symbols and 4, 10, and 7 as the number of symbols to use.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can anyone help me with these coding questions please

Transcribed Image Text:# Functions with Value Parameters
Use program **BoxesWithFunction** for Exercises 3-4. Program **BoxesWithFunction** is the shell of a program that prompts the user to enter an integer number. When completed, this number is read and passed to function Print as parameter `numSigns`. The function prints a box of dollar signs on the screen that is `numSigns` by `(numSigns / 2)` in dimension. For example, if `numSigns` is 10, the following box is printed on the screen:
```
$$$$$$$$$$
$ $
$ $
$$$$$$$$$$
```
Note the interior dimensions are `(numSigns – 2)` by `(numSigns / 2 – 2)`.
```cpp
// Program BoxesWithFunction prompts for the number of dollar signs
// for the top of the box. That number / 2 - 2 lines are
// printed with dollar signs on the sides.
#include <iostream>
using namespace std;
void Print(int numSigns);
int main ()
{
int number;
cout << "Enter the number of dollar signs for the top; "
<< "press return.";
cout << "Enter a negative number to quit."<endl;
cin >> number;
while (number>=0)
{
/* FILL IN call to Print */
cout << "Enter the number of dollar signs for the top; "
<< "press return.";
cout << "Enter a negative number to quit."<endl;
cin >> number;
}
return 0;
}
//*****************************************************
void Print(int numSigns)
// FILL IN documentation.
{
/* FILL IN code to print numSigns $'s */
/* FILL IN code to print (numSigns / 2) -2 lines with */
/* $'s lining up under the left-most and right-most */
/* $ ones on the top line. */
/* FILL IN code to print numSigns $'s */
}
```
## Exercise 3
Fill in the missing code in function `Print` and the invoking statement in function `main`. Compile and run your program using 4, 10, and 7 as input data. Don’t forget to fill in documentation for the function.
## Exercise 4
Rewrite your solution to Exercise 3 so that the symbol used as the border is also read as data and passed to function `Print` as
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
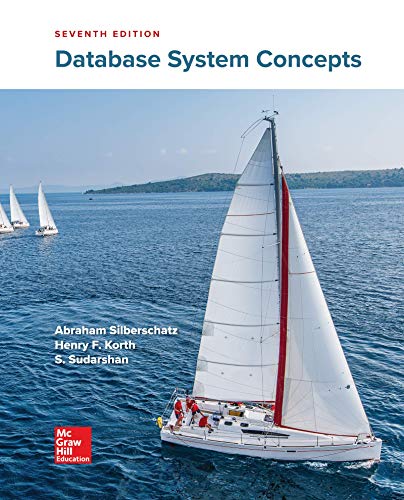
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
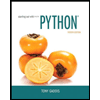
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
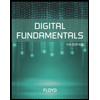
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
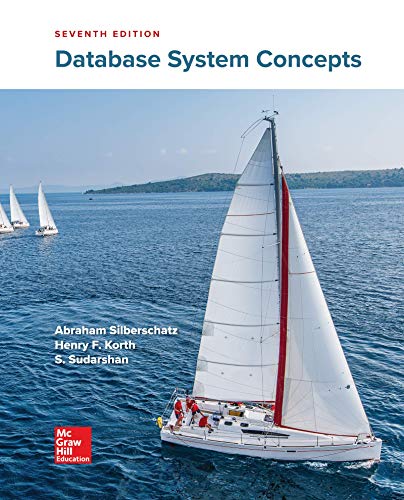
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
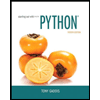
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
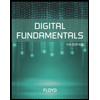
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
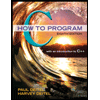
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
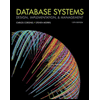
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
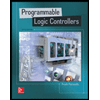
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education