I have created a C program that calculates the union of sets. Using the same parameter and logic I need help in creating intersection, difference and complement functions respectively. Following is the code below- #include #include typedef char String[21]; //if string is found, return 0. Otherwise, return -1. int Search(String key, String A[], int nElem) { int ctr; for(ctr = 0; ctr < nElem; ctr++) { if (strcmp(key, A[ctr])== 0) return 0; } return -1; } int setUnion(String *A, String *B, String *C, int nElemA, int nElemB) { //copy elements from A to C //copy elements from B to C //check for duplicate elements //take the 1st element of B and compare it in C //if the word is not found in C, add the element //otherwise, skip the word and proceed to the next element in B. /* A = { 1, 2, 3, 4, 5} B = { 2, 4, 6, 8, 10} A U B = { 1, 2, 3, 4, 5, 6, 8, 10} */ int ctr, cCount, found; //copy elements from A to C for(ctr = 0; ctr < nElemA; ctr++){ strcpy(C[ctr], A[ctr]); cCount = ctr + 1; } //copy elements from B to C for(ctr = 0; ctr < nElemB; ctr++){ found = Search(B[ctr], C, nElemB); if (found == -1){ strcpy(C[cCount], B[ctr]); cCount++; } } for(ctr = 0; ctr < cCount; ctr++) printf("%s ", C[ctr]); return cCount; } int main() { String A[10] = {"The", "quick", "brown", "fox"}; String B[10] = {"I", "will", "quick", "pass", "CCPROG2"}; String C[10]; String sKey; int totalWords; printf("Enter word to search : "); scanf("\n %s", sKey); int index = Search(sKey, A, 10); printf("\n %d \n", index); totalWords = setUnion(A, B, C, 4, 4); printf("\n %d \n", totalWords); //return 8 return 0; }
I have created a C program that calculates the union of sets. Using the same parameter and logic I need help in creating intersection, difference and complement functions respectively.
Following is the code below-
#include <stdio.h>
#include <string.h>
typedef char String[21];
//if string is found, return 0. Otherwise, return -1.
int Search(String key, String A[], int nElem)
{
int ctr;
for(ctr = 0; ctr < nElem; ctr++)
{
if (strcmp(key, A[ctr])== 0)
return 0;
}
return -1;
}
int setUnion(String *A, String *B, String *C, int nElemA, int nElemB)
{
//copy elements from A to C
//copy elements from B to C
//check for duplicate elements
//take the 1st element of B and compare it in C
//if the word is not found in C, add the element
//otherwise, skip the word and proceed to the next element in B.
/*
A = { 1, 2, 3, 4, 5}
B = { 2, 4, 6, 8, 10}
A U B = { 1, 2, 3, 4, 5, 6, 8, 10}
*/
int ctr, cCount, found;
//copy elements from A to C
for(ctr = 0; ctr < nElemA; ctr++){
strcpy(C[ctr], A[ctr]);
cCount = ctr + 1;
}
//copy elements from B to C
for(ctr = 0; ctr < nElemB; ctr++){
found = Search(B[ctr], C, nElemB);
if (found == -1){
strcpy(C[cCount], B[ctr]);
cCount++;
}
}
for(ctr = 0; ctr < cCount; ctr++)
printf("%s ", C[ctr]);
return cCount;
}
int main()
{
String A[10] = {"The", "quick", "brown", "fox"};
String B[10] = {"I", "will", "quick", "pass", "CCPROG2"};
String C[10];
String sKey;
int totalWords;
printf("Enter word to search : ");
scanf("\n %s", sKey);
int index = Search(sKey, A, 10);
printf("\n %d \n", index);
totalWords = setUnion(A, B, C, 4, 4);
printf("\n %d \n", totalWords); //return 8
return 0;
}

Step by step
Solved in 2 steps with 7 images

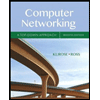
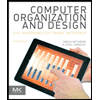
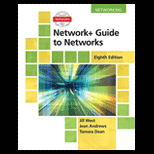
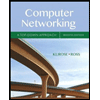
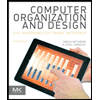
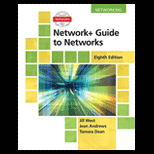
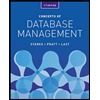
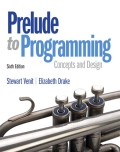
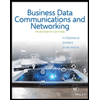