I have a program that asks for queries. It is based on a CSV file with three columns for a person's name, city, and height. The print statement for City_list gives it a blank. The problem I'm running into is that if I insert a city that isn't in the CSV file, it ends and doesn't transition to other cities. When I do put in the city that is in the list, it prints out the indexes from the file. How can this be revised to ignore off-list cities and work with in-list cities? Example Output: C:\Users\lucas\PycharmProjects\pythonProject3\venv\Scripts\python.exe C:\Users\lucas\Documents\cs1151\ch8\querydata.py Welcome to my query program! What would you like to do? 1) Count how many people in a city: 2) Find the tallest person/people in a city. 0) Quit Option: 1 Which city?Ely The population of Ely is 10 What would you like to do? 1) Count how many people in a city: 2) Find the tallest person/people in a city. 0) Quit Option: 2 Which city?Ely [1, 12, 13, 14, 20, 25, 40, 65, 84, 90]
I have a program that asks for queries. It is based on a CSV file with three columns for a person's name, city, and height. The print statement for City_list gives it a blank. The problem I'm running into is that if I insert a city that isn't in the CSV file, it ends and doesn't transition to other cities. When I do put in the city that is in the list, it prints out the indexes from the file. How can this be revised to ignore off-list cities and work with in-list cities?
Example Output:
C:\Users\lucas\PycharmProjects\pythonProject3\venv\Scripts\python.exe C:\Users\lucas\Documents\cs1151\ch8\querydata.py
Welcome to my query program!
What would you like to do?
1) Count how many people in a city:
2) Find the tallest person/people in a city.
0) Quit
Option: 1
Which city?Ely
The population of Ely is 10
What would you like to do?
1) Count how many people in a city:
2) Find the tallest person/people in a city.
0) Quit
Option: 2
Which city?Ely
[1, 12, 13, 14, 20, 25, 40, 65, 84, 90]
# Lucas Conklin
# 5772707
import csv
def readCSVIntoDictionary(f_name):
dictionary = dict()
with open(f_name) as f:
f_reader = csv.reader(f)
headers = next(f_reader)
id = 1
for row in f_reader:
item_dictionary = dict()
for i in range(0, len(row)):
item_dictionary[headers[i]] = row[i]
dictionary[id] = item_dictionary
id += 1
f.close()
return dictionary
def printItem(item):
for k, v in item.items():
print(f' {k:20}: {v}')
def lookupIDs(mydict, key, value):
matching_keys = []
for i in mydict.keys():
if mydict[i][key] == value:
matching_keys.append(i)
return matching_keys
def people_counter(dict, city):
city_pop = lookupIDs(dict, "city", city)
return len(city_pop)
def find_tallest(dict, city):
city_dict = lookupIDs(dict, "city", city)
print(city_dict)
tall = 0
for i in city_dict:
height = int(dict[i]["height"])
if height > tall:
tall = height
tallest = []
for i in city_dict:
height = int(dict[i]["height"])
if tall == height:
name = dict[i]["name"]
tallest.append(name)
while True:
if city_dict == []:
continue
return tallest
cityDictionary = readCSVIntoDictionary("C:\\Users\\lucas\\Downloads\\sample-data.csv")
print("Welcome to my query program!")
while True:
print("What would you like to do?")
print()
print("1) Count how many people in a city: ")
print("2) Find the tallest person/people in a city.")
print("0) Quit")
option = int(input("Option: "))
if option == 0:
print("Goodbye!")
break
elif option == 1:
city = input("Which city?")
pop = people_counter(cityDictionary, city)
if pop == 0:
print(f'There are no people in {city}.')
else:
print(f'The population of {city} is {pop}')
elif option == 2:
city = input("Which city?")
people_list = find_tallest(cityDictionary, city)
if len(people_list) <= 1:
print(f'The tallest people/person in {city} is {people_list[0]}')
else:
print(f'The tallest people/person in {city} are ')
for people in people_list:
print(f' {people} ')

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

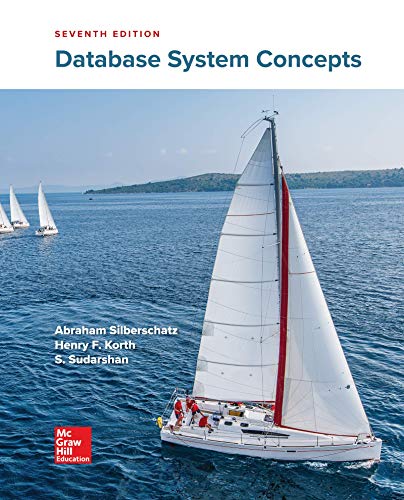
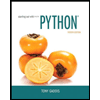
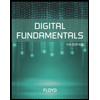
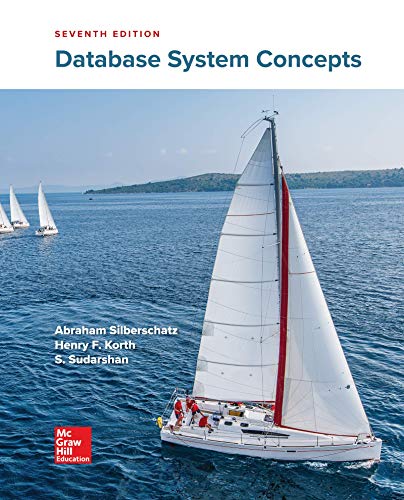
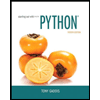
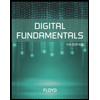
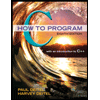
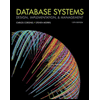
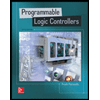