I am making a program that works with a CSV file with three columns: names, cities, and heights. I'm trying to find the person with the most average height in their city. I found the average, but I need to create a difference table to find the person whose height is closest to the average. import csv data = [] with open("C:\\Users\\lucas\\Downloads\\sample-data.csv") as f: reader = csv.reader(f) for row in csv.reader(f): data.append(list(row)) f.close() data.pop(0) # print(data) city_list = [] for i in range(len(data)): city_list.append(data[i][1]) city_list.sort() print(city_list) unique_cities = [] people_count = [] for city in city_list: # this if statement analyzes which cities are in unique_cities. If they are not in the list, # then they will be appended into the list if city not in unique_cities: unique_cities.append(city) for city in unique_cities: # because unique cities lists one of each city, # and because we want to count the frequency of the cities, we use city_list count = city_list.count(city) people_count.append(count) print(unique_cities) print(people_count) height = [] for d in data: if city == d[1]: height.append(float(d[2])) avg = sum(height)/len(height)
I am making a program that works with a CSV file with three columns: names, cities, and heights. I'm trying to find the person with the most average height in their city. I found the average, but I need to create a difference table to find the person whose height is closest to the average.
import csv
data = []
with open("C:\\Users\\lucas\\Downloads\\sample-data.csv") as f:
reader = csv.reader(f)
for row in csv.reader(f):
data.append(list(row))
f.close()
data.pop(0)
# print(data)
city_list = []
for i in range(len(data)):
city_list.append(data[i][1])
city_list.sort()
print(city_list)
unique_cities = []
people_count = []
for city in city_list:
# this if statement analyzes which cities are in unique_cities. If they are not in the list,
# then they will be appended into the list
if city not in unique_cities:
unique_cities.append(city)
for city in unique_cities:
# because unique cities lists one of each city,
# and because we want to count the frequency of the cities, we use city_list
count = city_list.count(city)
people_count.append(count)
print(unique_cities)
print(people_count)
height = []
for d in data:
if city == d[1]:
height.append(float(d[2]))
avg = sum(height)/len(height)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

How could this program work without using the lambda function?
import csv
data = []
with open("sample-data.csv") as f:
reader = csv.reader(f)
for row in csv.reader(f):
data.append(list(row))
f.close()
data.pop(0)
# Create a list to store the average height for each city
city_avg_heights = []
for row in data:
city = row[1]
height = float(row[2])
# Check if city already exists in list
for city_data in city_avg_heights:
if city_data[0] == city:
city_data[1].append(height)
break
else:
city_avg_heights.append([city, [height]])
# Calculate the average height for each city
for city_data in city_avg_heights:
heights = city_data[1]
avg_height = sum(heights) / len(heights)
city_data.append(avg_height)
# Create a list to store the difference between each person's height and their city's average height
person_diff = []
for row in data:
name = row[0]
city = row[1]
height = float(row[2])
for city_data in city_avg_heights:
if city_data[0] == city:
avg_height = city_data[2]
diff = abs(height - avg_height)
person_diff.append([name, city, diff])
break
# Sort the list of differences by city and then by difference
person_diff.sort(key=lambda x: (x[1], x[2]))
# Find the two people with the smallest absolute difference in each city
closest_people = []
for city_data in city_avg_heights:
city = city_data[0]
city_diffs = [person for person in person_diff if person[1] == city]
city_diffs.sort(key=lambda x: x[2])
closest_people.extend(city_diffs[:2])
# Print the two people in each city with the least difference
for person in closest_people:
print(f"The person with the closest height to the average in {person[1]} is {person[0]}")
What if you wanted to write this
What if I wanted to write this program without dictionaries but use a list to find differences from the average?
How do I make output for each person in their city?
How do I make output for each person in their city?
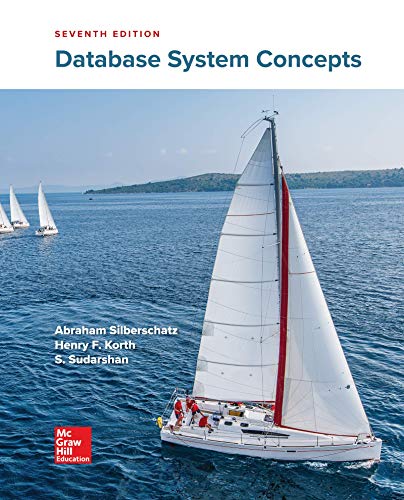
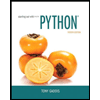
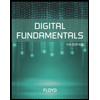
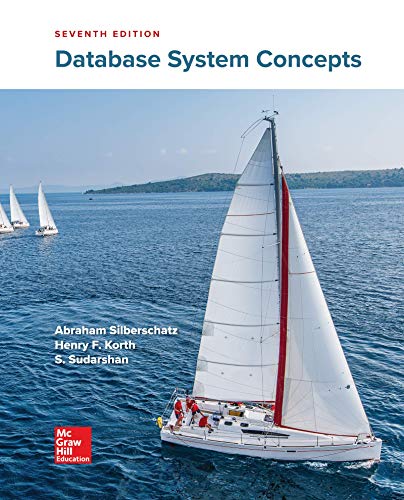
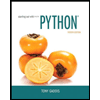
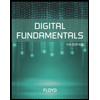
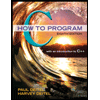
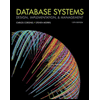
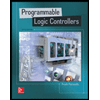