Write a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator (|) in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program is: movies.csv and the contents of movies.csv are: 16:40,Wonders of the World,G 20:00,Wonders of the World,G 19:00,Journey to Space ,PG-13 12:45,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 15:00,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 19:30,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 10:00,Adventures of Lewis and Clark,PG-13 14:30,Adventures of Lewis and Clark,PG-13 19:00,Halloween,R the output of the program is: Wonders of the World | G | 16:40 20:00 Journey to Space | PG-13 | 19:00 Buffalo Bill And The Indians or Sitting Bull | PG | 12:45 15:00 19:30 Adventures of Lewis and Clark | PG-13 | 10:00 14:30 Halloween | R | 19:00 import java.util.Scanner; import java.io.FileInputStream; import java.io.IOException; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); System.out.print(); String filename = scnr.nextLine(); try { FileInputStream fileStream = new FileInputStream(filename); Scanner fileScanner = new Scanner(fileStream); String currentTitle = ""; String currentRating = ""; String currentShowtimes = ""; while (fileScanner.hasNextLine()) { String line = fileScanner.nextLine(); String[] parts = line.split(","); String showtime = parts[0].trim(); String title = parts[1].trim(); String rating = parts[2].trim(); // Truncate or pad the title as needed title = (title.length() > 44) ? title.substring(0, 44) : title; if (title.equals(currentTitle)) { // Same movie, add showtime currentShowtimes += " " + showtime; } else { // New movie, print previous movie data if (!currentTitle.isEmpty()) { System.out.printf("%-44s | %-5s | %s\n", currentTitle, currentRating, currentShowtimes); } // Reset current movie data currentTitle = title; currentRating = rating; currentShowtimes = showtime; } } // Print last movie data if (!currentTitle.isEmpty()) { System.out.printf("%-44s | %-5s | %s\n", currentTitle, currentRating, currentShowtimes); } fileScanner.close(); fileStream.close(); } catch (IOException e) { System.out.println("File not found or could not be read: " + filename); } } }
Write a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements:
- Each row contains the title, rating, and all showtimes of a unique movie.
- A space is placed before and after each vertical separator (|) in each row.
- Column 1 displays the movie titles and is left justified with a minimum of 44 characters.
- If the movie title has more than 44 characters, output the first 44 characters only.
- Column 2 displays the movie ratings and is right justified with a minimum of 5 characters.
- Column 3 displays all the showtimes of the same movie, separated by a space.
Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows.
Ex: If the input of the program is:
movies.csv
and the contents of movies.csv are:
16:40,Wonders of the World,G 20:00,Wonders of the World,G 19:00,Journey to Space ,PG-13 12:45,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 15:00,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 19:30,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 10:00,Adventures of Lewis and Clark,PG-13 14:30,Adventures of Lewis and Clark,PG-13 19:00,Halloween,R
the output of the program is:
Wonders of the World | G | 16:40 20:00 Journey to Space | PG-13 | 19:00 Buffalo Bill And The Indians or Sitting Bull | PG | 12:45 15:00 19:30 Adventures of Lewis and Clark | PG-13 | 10:00 14:30 Halloween | R | 19:00
import java.util.Scanner;
import java.io.FileInputStream;
import java.io.IOException;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
System.out.print();
String filename = scnr.nextLine();
try {
FileInputStream fileStream = new FileInputStream(filename);
Scanner fileScanner = new Scanner(fileStream);
String currentTitle = "";
String currentRating = "";
String currentShowtimes = "";
while (fileScanner.hasNextLine()) {
String line = fileScanner.nextLine();
String[] parts = line.split(",");
String showtime = parts[0].trim();
String title = parts[1].trim();
String rating = parts[2].trim();
// Truncate or pad the title as needed
title = (title.length() > 44) ? title.substring(0, 44) : title;
if (title.equals(currentTitle)) {
// Same movie, add showtime
currentShowtimes += " " + showtime;
} else {
// New movie, print previous movie data
if (!currentTitle.isEmpty()) {
System.out.printf("%-44s | %-5s | %s\n", currentTitle, currentRating, currentShowtimes);
}
// Reset current movie data
currentTitle = title;
currentRating = rating;
currentShowtimes = showtime;
}
}
// Print last movie data
if (!currentTitle.isEmpty()) {
System.out.printf("%-44s | %-5s | %s\n", currentTitle, currentRating, currentShowtimes);
}
fileScanner.close();
fileStream.close();
} catch (IOException e) {
System.out.println("File not found or could not be read: " + filename);
}
}
}
![File
Paste
Clipboard
X
A1
1
2
3
4
5
5
7
3
9
Home Insert
Calibri
B
I U
Page Layout
A
B
16:40 Wonders G
20:00 Wonders G
Font
11
19:00 Journey to PG-13
12:45 Buffalo Bi PG
15:00 Buffalo Bi PG
19:30 Buffalo Bi PG
10:00 Adventure PG-13
14:30 Adventure PG-13
19:00 Hallowee R
Formulas
D
A A
S
4:40:00 PM
Data
E
Review
LL
View
Help
ab Wrap Text
Alignment
G
Merge & Center
H
movies [Read-Only] - Excel
Tell me what you want to do
i POSSIBLE DATA LOSS Some features might be lost if you save this workbook in the comma-delimited (.csv) format. To preserve these features, save it in an Excel
x ✓ fix
Custom
$
% ⁹ .00
Number
.00
K
S
F#
|||||
Conditional Format as
Formatting Table
Styles
M
N](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2Fa523bf9d-ff54-4d36-9e66-9210d1390c62%2F3y13bje_processed.png&w=3840&q=75)


- Start
- Import necessary Java libraries: Import the java.util.Scanner class for user input and file reading, and import java.io.FileInputStream and java.io.IOException for file handling.
- Create a Scanner object to read user input from the console.
- Prompt the user to enter the name of the CSV file and store the input as filename.
- Set up a try-catch block to handle potential exceptions that may occur when opening and reading the file.
- Inside the try block, create a FileInputStream to open the CSV file specified by filename, and create a Scanner (fileScanner) to read from the file.
- Initialize variables currentTitle, currentRating, and currentShowtimes to store information about the currently processed movie.
- Use a while loop to iterate through each line in the CSV file as long as fileScanner has more lines to read.
- For each line, split it into parts using a comma (,) as the delimiter, resulting in an array of strings.
- Extract the showtime, title, and rating from the parts array and trim any leading or trailing whitespace.
- Truncate or pad the title to a maximum length of 44 characters, as specified.
- Check if the title matches the currentTitle. If it does, it's the same movie, so append the showtime to the currentShowtimes.
- If the title is different (indicating a new movie), print the data for the previous movie (if it exists) in the specified format, using printf to format the output with the correct alignment and width.
- Update currentTitle, currentRating, and currentShowtimes with the information for the current movie.
- After the loop, print the data for the last movie (if it exists), using the same format as in step 12.
- Close the fileScanner and fileStream to release resources.
- In the catch block, handle any IOException exceptions that may occur, such as file not found or errors during file reading, and display an error message.
- Stop
Step by step
Solved in 4 steps with 1 images

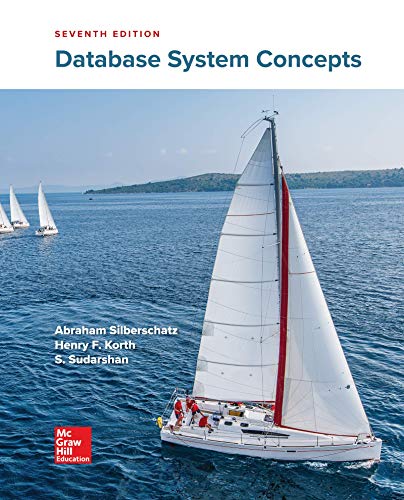
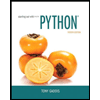
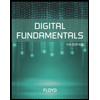
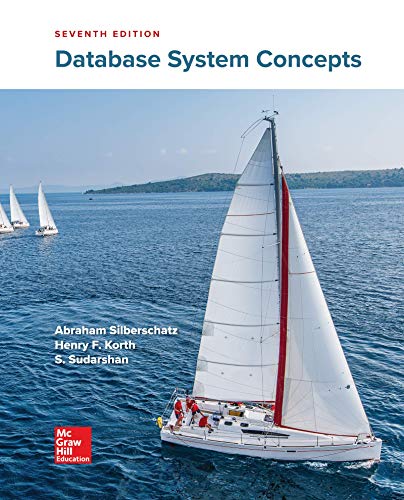
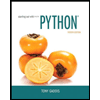
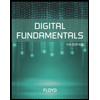
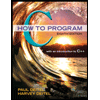
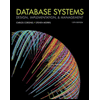
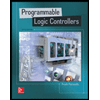