I am making a program with a CSV file with three columns: names, cities, and heights. In each city, I found the average height. I need to find the two people closest to the average. Most of the time, it works, but there is an error. A person second closest to the average doesn't even appear on the list. Also, the value for their height is way too high. What changes do I need to make? import csv data = [] with open("C:\\Users\\lucas\\Downloads\\sample-data.csv") as f: reader = csv.reader(f) for row in csv.reader(f): data.append(list(row)) f.close() data.pop(0) city_list = [] for i in range(len(data)): city_list.append(data[i][1]) city_list.sort() unique_cities = [] people_count = [] for city in city_list: # this if statement analyzes which cities are in unique_cities. If they are not in the list, # then they will be appended into the list if city not in unique_cities: unique_cities.append(city) for city in unique_cities: count = city_list.count(city) people_count.append(count) for k in range(len(unique_cities)): print(f'City: {unique_cities[k]}, Population: {people_count[k]}') # because unique cities lists one of each city, # and because we want to count the frequency of the cities, we use city_list for city in unique_cities: name_list = [] height_list = [] for d in data: if city == d[1]: height_list.append(float(d[2])) name_list.append(d[0]) avg = sum(height_list) / len(height_list) difference_list = [] for height in height_list: diff = abs(height - avg) difference_list.append(diff) closest_to_average_height = 1000000000 closest_to_average_name = " " second_closest_height = 1000000000 second_closest_name = " " difference_list_two = difference_list.copy() closest_to_average_diff = difference_list_two[0] # second_closest_to_average_diff = closest_to_average_diff for index in range(len(height_list)): current_diff = difference_list_two[index] if current_diff <= closest_to_average_diff: second_closest_to_average_diff = closest_to_average_diff second_closest_to_average_height = closest_to_average_height second_closest_to_average_name = closest_to_average_name closest_to_average_diff = current_diff closest_to_average_height = height_list[index] closest_to_average_name = name_list[index] print(f"City: {city} Most height: {closest_to_average_name}, Height: {closest_to_average_height}, " f"Second most height: {second_closest_to_average_name}, Height: {second_closest_to_average_height} ")
I am making a program with a CSV file with three columns: names, cities, and heights. In each city, I found the average height. I need to find the two people closest to the average. Most of the time, it works, but there is an error. A person second closest to the average doesn't even appear on the list. Also, the value for their height is way too high. What changes do I need to make?
import csv
data = []
with open("C:\\Users\\lucas\\Downloads\\sample-data.csv") as f:
reader = csv.reader(f)
for row in csv.reader(f):
data.append(list(row))
f.close()
data.pop(0)
city_list = []
for i in range(len(data)):
city_list.append(data[i][1])
city_list.sort()
unique_cities = []
people_count = []
for city in city_list:
# this if statement analyzes which cities are in unique_cities. If they are not in the list,
# then they will be appended into the list
if city not in unique_cities:
unique_cities.append(city)
for city in unique_cities:
count = city_list.count(city)
people_count.append(count)
for k in range(len(unique_cities)):
print(f'City: {unique_cities[k]}, Population: {people_count[k]}')
# because unique cities lists one of each city,
# and because we want to count the frequency of the cities, we use city_list
for city in unique_cities:
name_list = []
height_list = []
for d in data:
if city == d[1]:
height_list.append(float(d[2]))
name_list.append(d[0])
avg = sum(height_list) / len(height_list)
difference_list = []
for height in height_list:
diff = abs(height - avg)
difference_list.append(diff)
closest_to_average_height = 1000000000
closest_to_average_name = " "
second_closest_height = 1000000000
second_closest_name = " "
difference_list_two = difference_list.copy()
closest_to_average_diff = difference_list_two[0]
# second_closest_to_average_diff = closest_to_average_diff
for index in range(len(height_list)):
current_diff = difference_list_two[index]
if current_diff <= closest_to_average_diff:
second_closest_to_average_diff = closest_to_average_diff
second_closest_to_average_height = closest_to_average_height
second_closest_to_average_name = closest_to_average_name
closest_to_average_diff = current_diff
closest_to_average_height = height_list[index]
closest_to_average_name = name_list[index]
print(f"City: {city} Most height: {closest_to_average_name}, Height: {closest_to_average_height}, "
f"Second most height: {second_closest_to_average_name}, Height: {second_closest_to_average_height} ")

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

How could this program work...
with lists instead of dictionaries
without the functions lambda or key=
example:
sorted_order=dict(sorted(person_diff.items(), key=lambda item: item[1]))
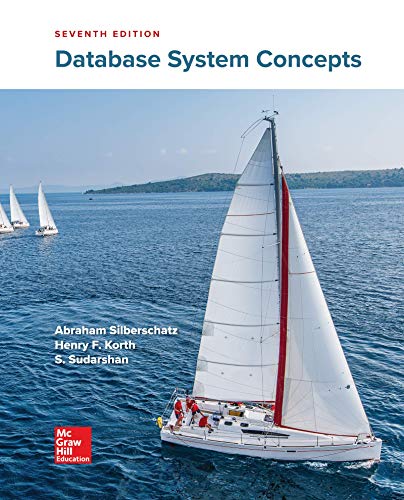
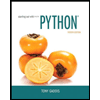
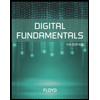
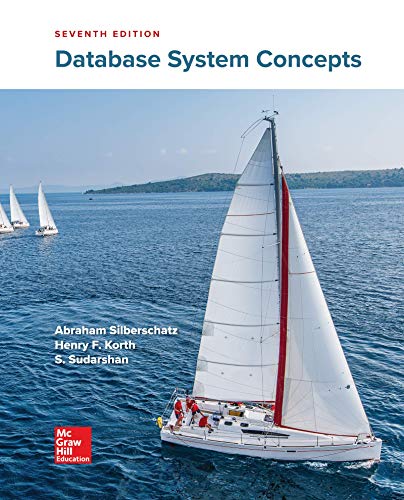
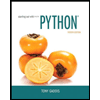
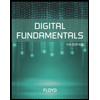
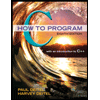
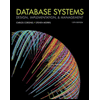
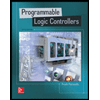