I can't instantiate this template class in C++. The C++ classes are below. What am I doing wrong? Error LNK2019 unresolved external symbol "public: __cdecl ArrayList::ArrayList(int)" (??0?$ArrayList@H@@QEAA@H@Z) referenced in function main Error LNK2019 unresolved external symbol "public: __cdecl ArrayList::~ArrayList(void)" (??1?$ArrayList@H@@QEAA@XZ) referenced in function main Main.cpp #include "ArrayList.h" int main() { ArrayList myList(10); } ArrayList.cpp #include "ArrayList.h" template bool ArrayList::isEmpty() const { return (length == 0); } template bool ArrayList::isFull() const { return (length == maxSize); } template int ArrayList::listSize() const { return length; } template int ArrayList::maxListSize() const { return maxSize; } template void ArrayList::insertEnd(const Type &insertItem) { if (length >= maxSize) // the list is fullcout cout << "Cannot insert in a full list" << endl; else { list[length] = insertItem; // insert the item at the end length++; // increment the length } } template void ArrayList::clearList() { length = 0; } template ArrayList::ArrayList(int size) { if (size < 0) { cout << "the array size must be positive. Creating " << "an array of size 100. " << endl; maxSize = 100; } else maxSize = size; length = 0; list = new Type[maxSize]; assert(list != NULL); } template ArrayList::ArrayList(const ArrayList &otherList) { maxSize = otherList.maxSize; length = otherList.length; list = new Type[maxSize]; // create the arrayassert(list != NULL); // terminate if unable to allocate//memory space for (int j = 0; j < length; j++) // copy otherList list[j] = otherList.list[j]; } template ArrayList::~ArrayList() { } ArrayList.h #include using namespace std; template class ArrayList { public: bool isEmpty() const; bool isFull() const; int listSize() const; int maxListSize() const; void insertEnd(const Type& insertItem); ArrayList(int size = 100); // constructor ArrayList(const ArrayList& otherList); // copy constructor ~ArrayList(); protected: Type* list; int length; int maxSize; };
I can't instantiate this template class in C++. The C++ classes are below. What am I doing wrong? Error LNK2019 unresolved external symbol "public: __cdecl ArrayList::ArrayList(int)" (??0?$ArrayList@H@@QEAA@H@Z) referenced in function main Error LNK2019 unresolved external symbol "public: __cdecl ArrayList::~ArrayList(void)" (??1?$ArrayList@H@@QEAA@XZ) referenced in function main Main.cpp #include "ArrayList.h" int main() { ArrayList myList(10); } ArrayList.cpp #include "ArrayList.h" template bool ArrayList::isEmpty() const { return (length == 0); } template bool ArrayList::isFull() const { return (length == maxSize); } template int ArrayList::listSize() const { return length; } template int ArrayList::maxListSize() const { return maxSize; } template void ArrayList::insertEnd(const Type &insertItem) { if (length >= maxSize) // the list is fullcout cout << "Cannot insert in a full list" << endl; else { list[length] = insertItem; // insert the item at the end length++; // increment the length } } template void ArrayList::clearList() { length = 0; } template ArrayList::ArrayList(int size) { if (size < 0) { cout << "the array size must be positive. Creating " << "an array of size 100. " << endl; maxSize = 100; } else maxSize = size; length = 0; list = new Type[maxSize]; assert(list != NULL); } template ArrayList::ArrayList(const ArrayList &otherList) { maxSize = otherList.maxSize; length = otherList.length; list = new Type[maxSize]; // create the arrayassert(list != NULL); // terminate if unable to allocate//memory space for (int j = 0; j < length; j++) // copy otherList list[j] = otherList.list[j]; } template ArrayList::~ArrayList() { } ArrayList.h #include using namespace std; template class ArrayList { public: bool isEmpty() const; bool isFull() const; int listSize() const; int maxListSize() const; void insertEnd(const Type& insertItem); ArrayList(int size = 100); // constructor ArrayList(const ArrayList& otherList); // copy constructor ~ArrayList(); protected: Type* list; int length; int maxSize; };
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
I can't instantiate this template class in C++. The C++ classes are below. What am I doing wrong?
Error LNK2019 unresolved external symbol "public: __cdecl ArrayList<int>::ArrayList<int>(int)" (??0?$ArrayList@H@@QEAA@H@Z) referenced in function main
Error LNK2019 unresolved external symbol "public: __cdecl ArrayList<int>::~ArrayList<int>(void)" (??1?$ArrayList@H@@QEAA@XZ) referenced in function main
Main.cpp
#include "ArrayList.h"
int main()
{
ArrayList<int> myList(10);
}
ArrayList.cpp
#include "ArrayList.h"
template <class Type>
bool ArrayList<Type>::isEmpty() const
{
return (length == 0);
}
template <class Type>
bool ArrayList<Type>::isFull() const
{
return (length == maxSize);
}
template <class Type>
int ArrayList<Type>::listSize() const
{
return length;
}
template <class Type>
int ArrayList<Type>::maxListSize() const
{
return maxSize;
}
template <class Type>
void ArrayList<Type>::insertEnd(const Type &insertItem)
{
if (length >= maxSize) // the list is fullcout
cout << "Cannot insert in a full list" << endl;
else
{
list[length] = insertItem; // insert the item at the end
length++; // increment the length
}
}
template <class Type>
void ArrayList<Type>::clearList()
{
length = 0;
}
template <class Type>
ArrayList<Type>::ArrayList(int size)
{
if (size < 0)
{
cout << "the array size must be positive. Creating "
<< "an array of size 100. " << endl;
maxSize = 100;
}
else
maxSize = size;
length = 0;
list = new Type[maxSize];
assert(list != NULL);
}
template <class Type>
ArrayList<Type>::ArrayList(const ArrayList<Type> &otherList)
{
maxSize = otherList.maxSize;
length = otherList.length;
list = new Type[maxSize]; // create the
arrayassert(list != NULL); // terminate if unable to allocate//memory space
for (int j = 0; j < length; j++) // copy otherList
list[j] = otherList.list[j];
}
template<class Type>
ArrayList<Type>::~ArrayList()
{
}
ArrayList.h
#include <iostream>
using namespace std;
template <class Type>
class ArrayList
{
public:
bool isEmpty() const;
bool isFull() const;
int listSize() const;
int maxListSize() const;
void insertEnd(const Type& insertItem);
ArrayList(int size = 100); // constructor
ArrayList(const ArrayList<Type>& otherList); // copy constructor
~ArrayList();
protected:
Type* list;
int length;
int maxSize;
};
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Recommended textbooks for you
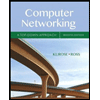
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
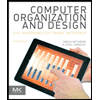
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
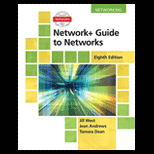
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
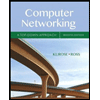
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
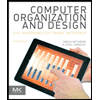
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
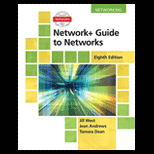
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
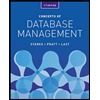
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
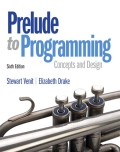
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
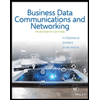
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY