How would you write main() as specified in the img below? This is what I have written(and it works) but it does not meet the requirments asked to have in main(), and likewise of determine_prime() as the actual text string should be produced in main(); def get_intro(): print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~") print("In Math, prime numbers are whole numbers greater than 1, that have only two factors the number 1 and the number itself.") print("This program will calculate if a number you enter is prime.") print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~") def validate_input(): while True: try: newNum = int(input("Enter a postive whole number to check if it is prime: ")) if newNum <= 0: print("Value must be a whole number greater than 0!") continue except ValueError: print("Value must be a whole number!") continue else: return newNum def determine_prime(isWhole): if isWhole < 2: # catch 1 and anything less than zero print("The definition of a prime number is a positive interger that has exactly two positive divisors.") print("The number 1 only has one positive divisor, so the number 1 is not prime.") else: prime = True for i in range(2, isWhole): if isWhole%i == 0: print("This number", isWhole,"is not prime") prime = False break if prime: print("This number", isWhole,"is prime") return isWhole def main(): get_intro() isWhole = validate_input() determine_prime(isWhole) do_again() def do_again(): print("Do you want to run the program again? ('Y' for yes, anything else to quit.)") cont = input() # Start cont = "Y" while cont == "Y": main()
How would you write main() as specified in the img below?
This is what I have written(and it works) but it does not meet the requirments asked to have in main(), and likewise of determine_prime() as the actual text string should be produced in main();
def get_intro():
print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
print("In Math, prime numbers are whole numbers greater than 1, that have only two factors the number 1 and the number itself.")
print("This
print("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~")
def validate_input():
while True:
try:
newNum = int(input("Enter a postive whole number to check if it is prime: "))
if newNum <= 0:
print("Value must be a whole number greater than 0!")
continue
except ValueError:
print("Value must be a whole number!")
continue
else:
return newNum
def determine_prime(isWhole):
if isWhole < 2: # catch 1 and anything less than zero
print("The definition of a prime number is a positive interger that has exactly two positive divisors.")
print("The number 1 only has one positive divisor, so the number 1 is not prime.")
else:
prime = True
for i in range(2, isWhole):
if isWhole%i == 0:
print("This number", isWhole,"is not prime")
prime = False
break
if prime:
print("This number", isWhole,"is prime")
return isWhole
def main():
get_intro()
isWhole = validate_input()
determine_prime(isWhole)
do_again()
def do_again():
print("Do you want to run the program again? ('Y' for yes, anything else to quit.)")
cont = input()
# Start
cont = "Y"
while cont == "Y":
main()



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

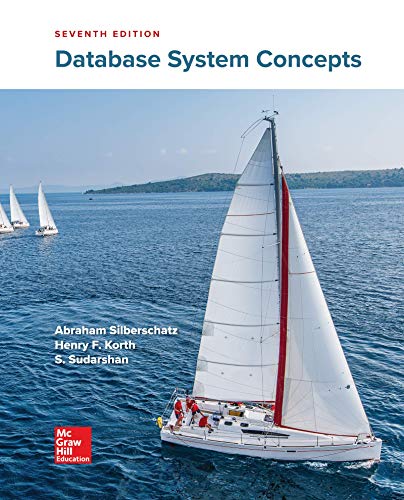
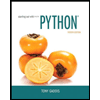
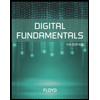
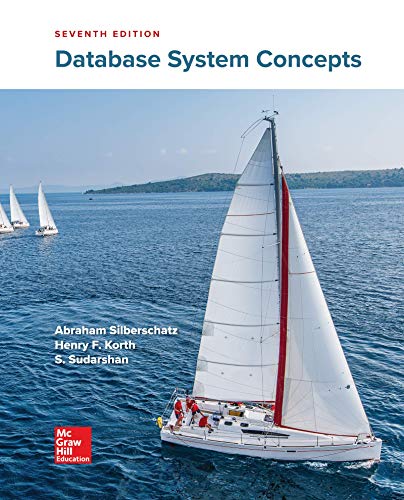
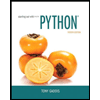
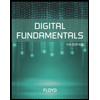
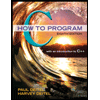
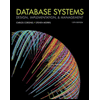
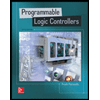