write an extension method called toRoman to convert and int to its roman r
Your job is to write an extension method called toRoman to convert and int to its roman representation so that a client code such as below would work.
static void Main(string[] args)
{
int i = 99;
string s = i.toRoman();
Console.WriteLine(s);
}
- Hint: Review Module 2-Chapter 3 slides and Extension Methods - C#
Programming Guide from Microsoft to understand how to implement an extension method is. There are example code there too - Hint: You can refer to this link for the
algorithm to convert a decimal to a roman number. - Hint: If you have issues compiling your code, make sure to include the extended method in its own static class with its own namespace.

//Program
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ConsoleApplication1
{
class Program
{
static String intToRoman(int num)
{
// storing roman values of digits from 0-9
// when placed at different places
String[] m = { "", "M", "MM", "MMM" };
String[] c = { "", "C", "CC", "CCC", "CD",
"D", "DC", "DCC", "DCCC", "CM" };
String[] x = { "", "X", "XX", "XXX", "XL",
"L", "LX", "LXX", "LXXX", "XC" };
String[] i = { "", "I", "II", "III", "IV",
"V", "VI", "VII", "VIII", "IX" };
// Converting to roman
String thousands = m[num / 1000];
String hundreds = c[(num % 1000) / 100];
String tens = x[(num % 100) / 10];
String ones = i[num % 10];
String ans = thousands + hundreds + tens + ones;
return ans;
}
static void Main(string[] args)
{
int number = 99;
Console.WriteLine(intToRoman(number));
Console.ReadLine();
}
}
}
Output:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

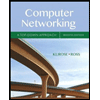
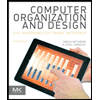
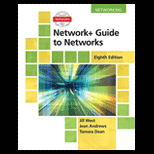
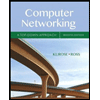
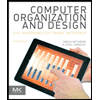
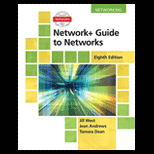
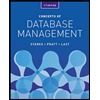
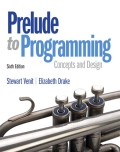
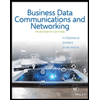