How do you implement the following template into the code shown below it? // -- brief statement as to the file’s purpose //XXXX-XXX- ADD YOUR SECTION NUMBER //Include statements #include #include using namespace std; //Global declarations: Constants and type definitions only -- no variables //Function prototypes int main() { //In cout statement below SUBSTITUTE your name and lab number cout << "Your name -- Lab Number" << endl << endl; //Variable declarations //Program logic //Closing program statements system("pause"); return 0; } //Function definitions Code: #include #include #include using namespace std; // function prototypes void Fire(char board[][25]); void FleetSunk(char board[][25], int&); int main() { // declaring board char board[25][25]; ifstream infile; // opening the input file infile.open("game.txt");// input file location should change depending on where it is if (!infile) { cout << "Can not open file." << endl; } // taking input from file for (int i = 0; i < 25; i++) { for (int j = 0; j < 25; j++) { char bS; infile >> bS; board[i][j] = bS; } } int fS = 0; // calling functions do { Fire(board); FleetSunk(board, fS); } while (fS == 0); system("PAUSE"); } void Fire(char board[25][25]) { int row = 0, row1 = 0; int col = 0, col1 = 0; // taking values from user for row and column cout << "Enter the Row and Column that you would like to try and hit :"; cin >> row1; cin >> col1; row = row1 - 1; col = col1 - 1; // printing msg switch (board[row][col]) { case '#': if (board[row - 1][col] == 'H') { cout << "HIT AGAIN" << endl; board[row][col] = 'H'; } else if (board[row + 1][col] == 'H') { cout << "HIT AGAIN" << endl; board[row][col] = 'H'; } else if (board[row][col - 1] == 'H') { cout << "HIT AGAIN" << endl; board[row][col] = 'H'; } else if (board[row][col + 1] == 'H') { cout << "HIT AGAIN" << endl; board[row][col] = 'H'; } else { cout << "HIT" << endl; board[row][col] = 'H'; } break; case '~': cout << "MISS" << endl; break; case 'H': cout << "You already destroyed these coordinates." << endl; break; } } void FleetSunk(char board[25][25], int& fS) { // checking if all ships have sunk for (int i = 0; i < 25; i++) { for (int j = 0; j < 25; j++) { if (board[i][j] == '#') { fS = 0; return; } } } cout << "The Fleet has been destroyed!" << endl; fS = 1; }
How do you implement the following template into the code shown below it?
// -- brief statement as to the file’s purpose
//XXXX-XXX- ADD YOUR SECTION NUMBER
//Include statements
#include <iostream>
#include <string>
using namespace std;
//Global declarations: Constants and type definitions only -- no variables
//Function prototypes
int main()
{
//In cout statement below SUBSTITUTE your name and lab number
cout << "Your name -- Lab Number" << endl << endl;
//Variable declarations
//Program logic
//Closing program statements
system("pause");
return 0;
}
//Function definitions
Code:
#include<iostream>
#include<fstream>
#include<string.h>
using namespace std;
// function prototypes
void Fire(char board[][25]);
void FleetSunk(char board[][25], int&);
int main()
{
// declaring board
char board[25][25];
ifstream infile;
// opening the input file
infile.open("game.txt");// input file location should change depending on where it is
if (!infile) {
cout << "Can not open file." << endl;
}
// taking input from file
for (int i = 0; i < 25; i++) {
for (int j = 0; j < 25; j++) {
char bS;
infile >> bS;
board[i][j] = bS;
}
}
int fS = 0;
// calling functions
do {
Fire(board);
FleetSunk(board, fS);
}
while (fS == 0);
system("PAUSE");
}
void Fire(char board[25][25])
{
int row = 0, row1 = 0;
int col = 0, col1 = 0;
// taking values from user for row and column
cout << "Enter the Row and Column that you would like to try and hit :";
cin >> row1;
cin >> col1;
row = row1 - 1;
col = col1 - 1;
// printing msg
switch (board[row][col]) {
case '#':
if (board[row - 1][col] == 'H') {
cout << "HIT AGAIN" << endl;
board[row][col] = 'H';
}
else if (board[row + 1][col] == 'H') {
cout << "HIT AGAIN" << endl;
board[row][col] = 'H';
}
else if (board[row][col - 1] == 'H') {
cout << "HIT AGAIN" << endl;
board[row][col] = 'H';
}
else if (board[row][col + 1] == 'H') {
cout << "HIT AGAIN" << endl;
board[row][col] = 'H';
}
else {
cout << "HIT" << endl;
board[row][col] = 'H';
}
break;
case '~':
cout << "MISS" << endl;
break;
case 'H':
cout << "You already destroyed these coordinates." << endl;
break;
}
}
void FleetSunk(char board[25][25], int& fS) {
// checking if all ships have sunk
for (int i = 0; i < 25; i++) {
for (int j = 0; j < 25; j++) {
if (board[i][j] == '#') {
fS = 0;
return;
}
}
}
cout << "The Fleet has been destroyed!" << endl;
fS = 1;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

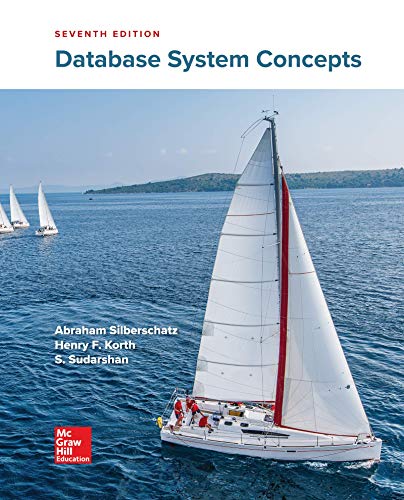
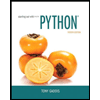
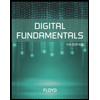
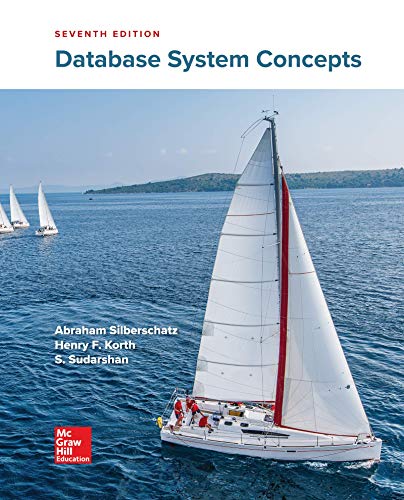
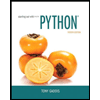
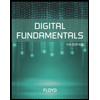
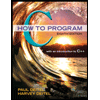
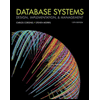
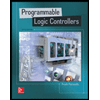