Hello, I've attached the prompt for this assignment. I need help creating code to delete and update in the contactService.java according to the prompt. This is my partial code. package Contact; import java.util.ArrayList; public class ContactService { //Start with an ArrayList of contacts to hold the list of contacts ArrayList contactList = new ArrayList(); //Display the full list of contacts to the console for error checking. public void displayContactList() { for(int counter = 0; counter < contactList.size(); counter++) {
Hello, I've attached the prompt for this assignment. I need help creating code to delete and update in the contactService.java according to the prompt. This is my partial code.
package Contact;
import java.util.ArrayList;
public class ContactService {
//Start with an ArrayList of contacts to hold the list of contacts
ArrayList<Contact> contactList = new ArrayList<Contact>();
//Display the full list of contacts to the console for error checking.
public void displayContactList() {
for(int counter = 0; counter < contactList.size(); counter++) {
System.out.println("\t Contact ID: " + contactList.get(counter).getContactID());
System.out.println("\t First Name: " + contactList.get(counter).getFirstName());
System.out.println("\t Last Name: " + contactList.get(counter).getLastName());
System.out.println("\t Phone Number: " + contactList.get(counter).getNumber());
System.out.println("\t Address: " + contactList.get(counter).getAddress() + "\n");
}
}
//Adds a new contact using the Contact constructor, then assign the new contact to the list.
public void addContact(String firstName, String lastName, String number, String address) {
// Create the new contact
Contact contact = new Contact(firstName, lastName, number, address);
contactList.add(contact);
}
This is my completed Contact.java


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

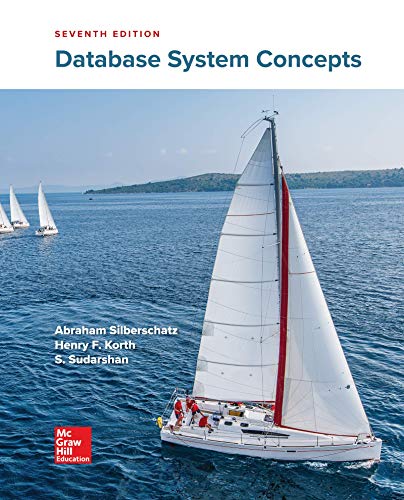
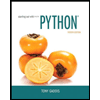
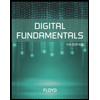
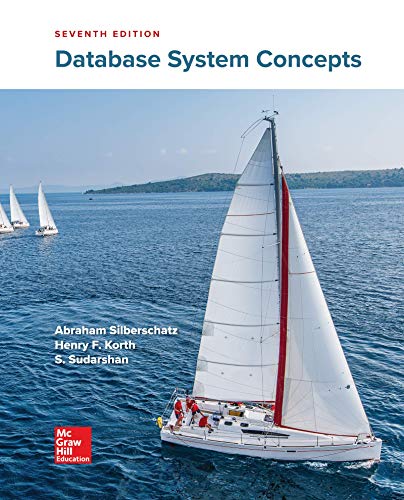
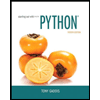
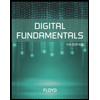
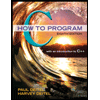
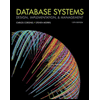
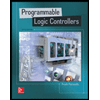