Using comments within the code itself, can you provide an line by line explanation of the below JavaScript file? The file itself deals with WebGl and if that helps you. Please and thank you JavaScript file: function mat2() { var out = new Array(2); out[0] = new Array(2); out[1] = new Array(2); switch ( arguments.length ) { case 0: out[0][0]=out[3]=1.0; out[1]=out[2]=0.0; break; case 1: if(arguments[0].type == 'mat2') { out[0][0] = arguments[0][0][0]; out[0][1] = arguments[0][0][1]; out[1][0] = arguments[0][1][0]; out[1][1] = arguments[0][1][1]; break; } case 4: out[0][0] = arguments[0]; out[0][1] = arguments[1]; out[1][0] = arguments[2]; out[1][1] = arguments[3]; break; default: throw "mat2: wrong arguments"; } out.type = 'mat2'; return out; } //---------------------------------------------------------------------------- function mat3() { // v = _argumentsToArray( arguments ); var out = new Array(3); out[0] = new Array(3); out[1] = new Array(3); out[2] = new Array(3); switch ( arguments.length ) { case 0: out[0][0]=out[1][1]=out[2][2]=1.0; out[0][1]=out[0][2]=out[1][0]=out[1][2]=out[2][0]=out[2][1]=0.0; break; case 1: for(var i=0; i<3; i++) for(var j=0; j<3; j++) { out[i][j]=arguments[0][3*i+j]; } break; case 9: for(var i=0; i<3; i++) for(var j=0; j<3; j++) { out[i][j] = arguments[3*i+j]; } break; default: throw "mat3: wrong arguments"; } out.type = 'mat3'; return out; } //---------------------------------------------------------------------------- function mat4() { //var v = _argumentsToArray( arguments ); var out = new Array(4); out[0] = new Array(4); out[1] = new Array(4); out[2] = new Array(4); out[3] = new Array(4); switch ( arguments.length ) { case 0: out[0][0]=out[1][1]=out[2][2]=out[3][3] = 1.0; out[0][1]=out[0][2]=out[0][3]=out[1][0]=out[1][2]=out[1][3]=out[2][0]=out[2][1] =out[2][3]=out[3][0]=out[3][1]=out[3][2]=0.0; break; case 1: for(var i=0; i<4; i++) for(var i=0; i<4; i++) { out[i][j]=arguments[0][4*i+j]; } break; case 4: if(arguments[0].type == "vec4") { for( var i=0; i<4; i++) for(var j=0; j<4; j++) out[i][j] = arguments[i][j]; break; } case 16: for(var i=0; i<4; i++) for(var j=0; j<4; j++) { out[i][j] = arguments[4*i+j]; } break; } out.type = 'mat4'; return out; } //---------------------------------------------------------------------------- // // Generic Mathematical Operations for Vectors and Matrices // function equal( u, v ) { if(!(isMatrix(u)&&isMatrix(v) || (isVector(u)&&isVector(v)))) throw "equal: at least one input not a vec or mat"; if ( u.type != v.type ) throw "equal: types different"; if(isMatrix(u)) { for ( var i = 0; i < u.length; ++i ) for ( var j = 0; j < u.length; ++j ) if ( u[i][j] !== v[i][j] ) return false; return true; } if(isVector(u)) { for ( var i = 0; i < u.length; ++i ) if ( u[i] !== v[i] ) return false; return true; } }
Using comments within the code itself, can you provide an line by line explanation of the below JavaScript file? The file itself deals with WebGl and if that helps you.
Please and thank you
JavaScript file:
function mat2()
{
var out = new Array(2);
out[0] = new Array(2);
out[1] = new Array(2);
switch ( arguments.length ) {
case 0:
out[0][0]=out[3]=1.0;
out[1]=out[2]=0.0;
break;
case 1:
if(arguments[0].type == 'mat2') {
out[0][0] = arguments[0][0][0];
out[0][1] = arguments[0][0][1];
out[1][0] = arguments[0][1][0];
out[1][1] = arguments[0][1][1];
break;
}
case 4:
out[0][0] = arguments[0];
out[0][1] = arguments[1];
out[1][0] = arguments[2];
out[1][1] = arguments[3];
break;
default:
throw "mat2: wrong arguments";
}
out.type = 'mat2';
return out;
}
//----------------------------------------------------------------------------
function mat3()
{
// v = _argumentsToArray( arguments );
var out = new Array(3);
out[0] = new Array(3);
out[1] = new Array(3);
out[2] = new Array(3);
switch ( arguments.length ) {
case 0:
out[0][0]=out[1][1]=out[2][2]=1.0;
out[0][1]=out[0][2]=out[1][0]=out[1][2]=out[2][0]=out[2][1]=0.0;
break;
case 1:
for(var i=0; i<3; i++) for(var j=0; j<3; j++) {
out[i][j]=arguments[0][3*i+j];
}
break;
case 9:
for(var i=0; i<3; i++) for(var j=0; j<3; j++) {
out[i][j] = arguments[3*i+j];
}
break;
default:
throw "mat3: wrong arguments";
}
out.type = 'mat3';
return out;
}
//----------------------------------------------------------------------------
function mat4()
{
//var v = _argumentsToArray( arguments );
var out = new Array(4);
out[0] = new Array(4);
out[1] = new Array(4);
out[2] = new Array(4);
out[3] = new Array(4);
switch ( arguments.length ) {
case 0:
out[0][0]=out[1][1]=out[2][2]=out[3][3] = 1.0;
out[0][1]=out[0][2]=out[0][3]=out[1][0]=out[1][2]=out[1][3]=out[2][0]=out[2][1]
=out[2][3]=out[3][0]=out[3][1]=out[3][2]=0.0;
break;
case 1:
for(var i=0; i<4; i++) for(var i=0; i<4; i++) {
out[i][j]=arguments[0][4*i+j];
}
break;
case 4:
if(arguments[0].type == "vec4") {
for( var i=0; i<4; i++)
for(var j=0; j<4; j++)
out[i][j] = arguments[i][j];
break;
}
case 16:
for(var i=0; i<4; i++) for(var j=0; j<4; j++) {
out[i][j] = arguments[4*i+j];
}
break;
}
out.type = 'mat4';
return out;
}
//----------------------------------------------------------------------------
//
// Generic Mathematical Operations for
//
function equal( u, v )
{
if(!(isMatrix(u)&&isMatrix(v) || (isVector(u)&&isVector(v))))
throw "equal: at least one input not a vec or mat";
if ( u.type != v.type ) throw "equal: types different";
if(isMatrix(u)) {
for ( var i = 0; i < u.length; ++i ) for ( var j = 0; j < u.length; ++j )
if ( u[i][j] !== v[i][j] ) return false;
return true;
}
if(isVector(u)) {
for ( var i = 0; i < u.length; ++i )
if ( u[i] !== v[i] ) return false;
return true;
}
}

Step by step
Solved in 4 steps with 8 images

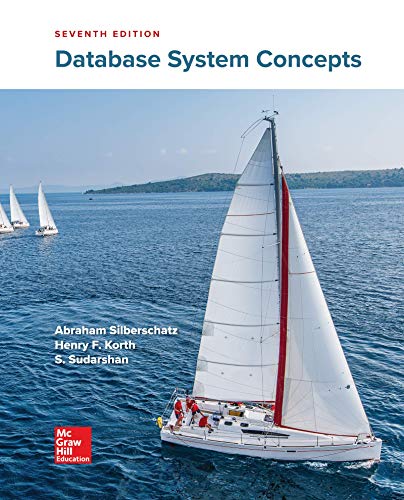
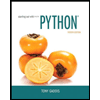
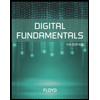
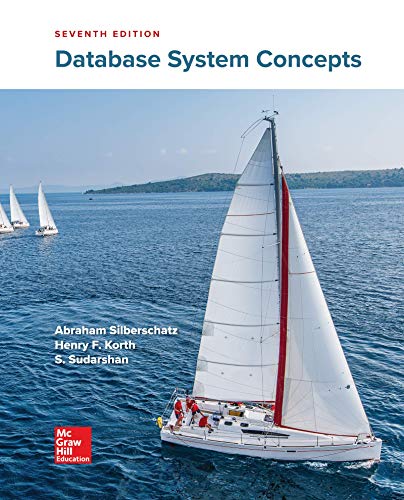
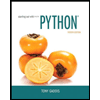
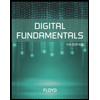
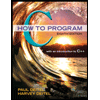
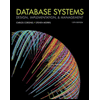
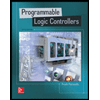