Hello! I am trying to debug this assigment. I already find some bugs and fixed them, however; I am stuck and struggling with the rest. I would appreciate a hint and explanation to continue. Thank you. import java.util.*; // for scanner public class Calc { public static void main(String[] args) { boolean done = false; Scanner console = new Scanner (System.in); while (!done){ displayMenu(); selection = getUserSelection(console); done = processSelection(selection, console); } System.out.println("Thank you for using this program. Goodbye."); } private static boolean processSelection(String selection, Scanner console) { boolean done = false; if (!selection.equalsIgnoreCase("E")){ if (selection.equalsIgnoreCase("U")){ calculateResults(console); } else if (selection.equalsIgnoreCase("H")){ //No need to write a Help Menu at this time, but logic and program execution // should still operate normally and display original menu after message prints System.out.print("Help Menu is under construction, " + "hence not available at this time."); } else { System.out.println("Incorrect entry...please try again!"); } } else { done = true; } return done; } private static void calculateResults(Scanner console) { //displayCalculatorInstructions(); double operand1 = console.nextDouble(); char operator = console.next().charAt(0); double operand2 = console.nextDouble(); double result = 0.0; boolean isOperatorValid = true; if (operator == '+'){ result = operand1 + operand2; } else if (operator == '-'){ result = operand1 - operand2; } else if (operator == '*'){ result = operand1 + operand2; } else if (operator == '/'){ if (operand2 != 0.0){ result = operand1/operand2; } else { result = Double.NaN; //Returns Not a Number } } else if (operator == '^'){ result = Math.pow(operand2, operand1); } else { isOperatorValid = false; } if (isOperatorValid){ System.out.println(operand1 +" "+operator+""+operand2+" = " + result); //One could also use printf to control the precision of result, but not necessary now. } } private static void displayCalculatorInstructions() { System.out.println("Enter a mathematical expression to evaluate."); System.out.println("Valid operations are: +, -, /, *, ^ (for exponents)."); System.out.println("Input expression using spaces between the operands (numbers) " + "and the operator, followed by Enter."); System.out.println("Here is the valid format:"); System.out.println("\t "); System.out.print("Your expression: "); } //The method header for getUserSelection has no errors. private static String getUserSelection(Scanner console) { String selection = console.nextInt(); return selection; } private static void displayMenu() { System.out.println("Enter one these options:"); System.out.println("\tH for Help"); System.out.println("\tC for using calculator"); System.out.println("\tE for exiting this program"); System.out.print("Your selection: "); } }
Hello! I am trying to debug this assigment. I already find some bugs and fixed them, however; I am stuck and struggling with the rest. I would appreciate a hint and explanation to continue. Thank you.
import java.util.*; // for scanner
public class Calc {
public static void main(String[] args) {
boolean done = false;
Scanner console = new Scanner (System.in);
while (!done){
displayMenu();
selection = getUserSelection(console);
done = processSelection(selection, console);
}
System.out.println("Thank you for using this
}
private static boolean processSelection(String selection, Scanner console) {
boolean done = false;
if (!selection.equalsIgnoreCase("E")){
if (selection.equalsIgnoreCase("U")){
calculateResults(console);
}
else if (selection.equalsIgnoreCase("H")){
//No need to write a Help Menu at this time, but logic and program execution
// should still operate normally and display original menu after message prints
System.out.print("Help Menu is under construction, "
+ "hence not available at this time.");
}
else {
System.out.println("Incorrect entry...please try again!");
}
}
else {
done = true;
}
return done;
}
private static void calculateResults(Scanner console) {
//displayCalculatorInstructions();
double operand1 = console.nextDouble();
char operator = console.next().charAt(0);
double operand2 = console.nextDouble();
double result = 0.0;
boolean isOperatorValid = true;
if (operator == '+'){
result = operand1 + operand2;
}
else if (operator == '-'){
result = operand1 - operand2;
}
else if (operator == '*'){
result = operand1 + operand2;
}
else if (operator == '/'){
if (operand2 != 0.0){
result = operand1/operand2;
}
else {
result = Double.NaN; //Returns Not a Number
}
}
else if (operator == '^'){
result = Math.pow(operand2, operand1);
}
else {
isOperatorValid = false;
}
if (isOperatorValid){
System.out.println(operand1 +" "+operator+""+operand2+" = " + result);
//One could also use printf to control the precision of result, but not necessary now.
}
}
private static void displayCalculatorInstructions() {
System.out.println("Enter a mathematical expression to evaluate.");
System.out.println("Valid operations are: +, -, /, *, ^ (for exponents).");
System.out.println("Input expression using spaces between the operands (numbers) "
+ "and the operator, followed by Enter.");
System.out.println("Here is the valid format:");
System.out.println("\t<operand1> <space> <operator> <space> <operand2> <Enter>");
System.out.print("Your expression: ");
}
//The method header for getUserSelection has no errors.
private static String getUserSelection(Scanner console) {
String selection = console.nextInt();
return selection;
}
private static void displayMenu() {
System.out.println("Enter one these options:");
System.out.println("\tH for Help");
System.out.println("\tC for using calculator");
System.out.println("\tE for exiting this program");
System.out.print("Your selection: ");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

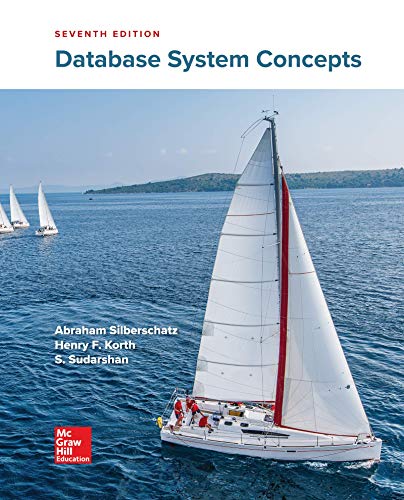
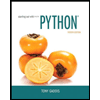
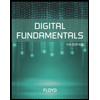
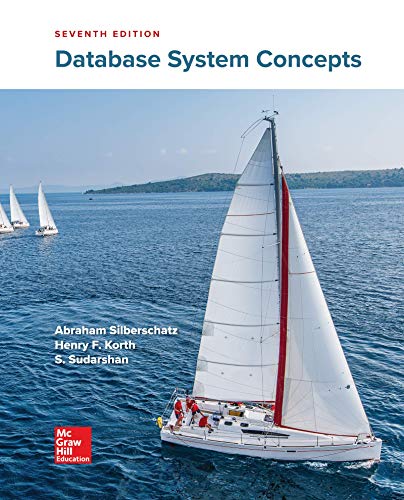
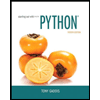
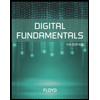
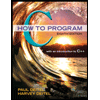
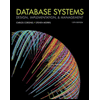
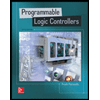