How do I fix the result? import java.lang.*; import java.util.Scanner; // import the Scanner class public class Main { public static Scanner keyboard = new Scanner(System.in); public static void main(String[] args) { boolean doItAgain = false; do { doItAgain = false; calcType(); System.out.println("Do you want to start over? (Y/N)"); //Scanner keyboard = new Scanner(System.in); String decision = keyboard.next(); if(decision.equals("Y")) { doItAgain = true; } } while(doItAgain); System.out.println("Goodbye"); } public static void calcType() { String mode; System.out.println("Enter the calculator mode: Standard/Scientific?"); //Scanner keyboard = new Scanner(System.in); mode = keyboard.next(); if(mode.equals("Standard")) { standard(); } else if (mode.equals("Scientific")) { scientific(); } else System.out.println("Unknown calculator type: " + mode); } public static void standard() { System.out.println("The calculator will operate in standard mode."); boolean doItAgain; do { doItAgain = false; System.out.println("Enter '+' for addition, '-' for subtractions, '*' for multiplication, '/' for division"); String operation; //Scanner keyboard = new Scanner(System.in); operation = keyboard.next(); //System.out.println("DB: operation = " + operation); if(operation.equals("+") ) standardCalcHelper('+'); else if(operation.equals("-")) standardCalcHelper('-'); else if(operation.equals("*")) standardCalcHelper('*'); else if(operation.equals("/")) standardCalcHelper('/'); else { System.out.println("Invalid operator " + operation); doItAgain = true; } } while(doItAgain); } public static void scientific() { System.out.println("The calculator will operate in scientific mode."); boolean doItAgain; do { doItAgain = false; System.out.println("Enter '+' for addition, '-' for subtractions, '*' for multiplication, '/' for division, 'sin' for sin x, 'cos' for cos x, 'tan' for tan x:"); String operation; // Scanner keyboard = new Scanner(System.in); operation = keyboard.next(); if(operation.equals("+")) standardCalcHelper('+'); else if(operation.equals("-")) standardCalcHelper('-'); else if(operation.equals("*")) standardCalcHelper('*'); else if(operation.equals("/")) standardCalcHelper('/'); else if(operation.equals("sin")) scientificCalcHelper('s'); else if(operation.equals("cos")) scientificCalcHelper('c'); else if(operation.equals("tan"))scientificCalcHelper('t'); else { System.out.println("Invalid operator " + operation); doItAgain = true; } } while(doItAgain); } static String getOperationName(char operation) { if(operation == '+') return "add"; else if(operation == '-') return "subtract"; else if(operation == '*') return "multiply"; else if(operation == '/') return "divide"; else if(operation == 's') return "sine"; else if(operation == 'c') return "cosine"; else if(operation == 't') return "tangent"; return ""; } static void standardCalcHelper(char operation) { //Scanner sc = new Scanner(System.in); System.out.println("How many numbers do you want to " + getOperationName(operation) + "?"); int times = keyboard.nextInt(); // if(times > 0) System.out.println("Enter " + times + " numbers"); double result = keyboard.nextDouble(); for (int i = 0; i < times-1; i++){ if(operation == '+') result += keyboard.nextDouble(); else if(operation == '-') result -= keyboard.nextDouble(); else if(operation == '*') result *= keyboard.nextDouble(); else if(operation == '/') result /= keyboard.nextDouble(); } System.out.println("Result: " + result); } static void scientificCalcHelper(char operation) { System.out.println("Enter a number in radians to find the " + getOperationName(operation)); double number; Scanner scanner =new Scanner(System.in); number = scanner.nextInt(); // double radian = keyboard.nextDouble(); // double result = Math.sin(radian); // System.out.println( "Result: " + result); if(operation == 's') System.out.println("Result: " + Math.sin(number)); else if(operation=='c') System.out.println("Result: " + Math.cos(number)); else if(operation == 't') System.out.println("Result: " + Math.tan(number)); } }
How do I fix the result?
import java.lang.*;
import java.util.Scanner; // import the Scanner class
public class Main {
public static Scanner keyboard = new Scanner(System.in);
public static void main(String[] args) {
boolean doItAgain = false;
do
{
doItAgain = false;
calcType();
System.out.println("Do you want to start over? (Y/N)");
//Scanner keyboard = new Scanner(System.in);
String decision = keyboard.next();
if(decision.equals("Y")) {
doItAgain = true;
}
}
while(doItAgain);
System.out.println("Goodbye");
}
public static void calcType() {
String mode;
System.out.println("Enter the calculator mode: Standard/Scientific?");
//Scanner keyboard = new Scanner(System.in);
mode = keyboard.next();
if(mode.equals("Standard")) {
standard();
}
else if (mode.equals("Scientific")) {
scientific();
}
else
System.out.println("Unknown calculator type: " + mode);
}
public static void standard() {
System.out.println("The calculator will operate in standard mode.");
boolean doItAgain;
do
{
doItAgain = false;
System.out.println("Enter '+' for addition, '-' for subtractions, '*' for multiplication, '/' for division");
String operation;
//Scanner keyboard = new Scanner(System.in);
operation = keyboard.next();
//System.out.println("DB: operation = " + operation);
if(operation.equals("+") ) standardCalcHelper('+');
else if(operation.equals("-")) standardCalcHelper('-');
else if(operation.equals("*")) standardCalcHelper('*');
else if(operation.equals("/")) standardCalcHelper('/');
else {
System.out.println("Invalid operator " + operation);
doItAgain = true;
}
}
while(doItAgain);
}
public static void scientific() {
System.out.println("The calculator will operate in scientific mode.");
boolean doItAgain;
do
{
doItAgain = false;
System.out.println("Enter '+' for addition, '-' for subtractions, '*' for multiplication, '/' for division, 'sin' for sin x, 'cos' for cos x, 'tan' for tan x:");
String operation;
// Scanner keyboard = new Scanner(System.in);
operation = keyboard.next();
if(operation.equals("+")) standardCalcHelper('+');
else if(operation.equals("-")) standardCalcHelper('-');
else if(operation.equals("*")) standardCalcHelper('*');
else if(operation.equals("/")) standardCalcHelper('/');
else if(operation.equals("sin")) scientificCalcHelper('s');
else if(operation.equals("cos")) scientificCalcHelper('c');
else if(operation.equals("tan"))scientificCalcHelper('t');
else {
System.out.println("Invalid operator " + operation);
doItAgain = true;
}
}
while(doItAgain);
}
static String getOperationName(char operation) {
if(operation == '+') return "add";
else if(operation == '-') return "subtract";
else if(operation == '*') return "multiply";
else if(operation == '/') return "divide";
else if(operation == 's') return "sine";
else if(operation == 'c') return "cosine";
else if(operation == 't') return "tangent";
return "";
}
static void standardCalcHelper(char operation) {
//Scanner sc = new Scanner(System.in);
System.out.println("How many numbers do you want to " + getOperationName(operation) + "?");
int times = keyboard.nextInt();
// if(times > 0)
System.out.println("Enter " + times + " numbers");
double result = keyboard.nextDouble();
for (int i = 0; i < times-1; i++){
if(operation == '+') result += keyboard.nextDouble();
else if(operation == '-') result -= keyboard.nextDouble();
else if(operation == '*') result *= keyboard.nextDouble();
else if(operation == '/') result /= keyboard.nextDouble();
}
System.out.println("Result: " + result);
}
static void scientificCalcHelper(char operation) {
System.out.println("Enter a number in radians to find the " + getOperationName(operation));
double number;
Scanner scanner =new Scanner(System.in);
number = scanner.nextInt();
// double radian = keyboard.nextDouble();
// double result = Math.sin(radian);
// System.out.println( "Result: " + result);
if(operation == 's')
System.out.println("Result: " + Math.sin(number));
else if(operation=='c')
System.out.println("Result: " + Math.cos(number));
else if(operation == 't')
System.out.println("Result: " + Math.tan(number));
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

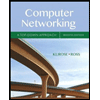
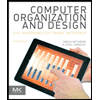
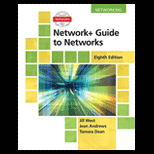
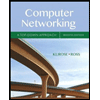
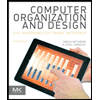
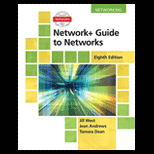
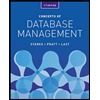
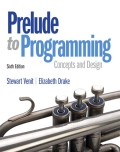
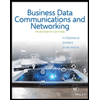