Hello, I am experimenting with Python. There is a lot of # comments in the code to help me understand it better, but there is an error at line 52 with the input_file PdfFileReader and I do not know how to fix the code. Can you help me? # Import easygui and pypdf2 import easygui as gui # imports easygui from PyPDF2 import PdfFileReader, PdfFileWriter # imports PyPDF2 # 1. Display file selection dialog for opening a PDF file. This allows the user to select a PDF that they can rotate! input_path = gui.fileopenbox( # sets the input path and opens a gui box title="Select a PDF to rotate...", # sets title default="*.pdf" # sets the default file type ) # 2. If user cancels the dialog, exit the program. The program will automatically do this if the user cancels! if input_path is None: # if statement for input path being 'none' exit() # exits the file # 3. Ask user to select one of 90, 180, or 270 degrees. This is how much they will rotate their selected PDF by! choices = ("90", "180", "270") # degree choices for rotating a PDF degrees = gui.buttonbox( # creates buttonbox gui for degrees msg="Rotate the PDF clockwise by how many degrees?", # sets the message for the box title="Choose rotation...", # sets the title for the box choices=choices, # sets the choices ) # closes the function # 4. Display a file selection dialog for saving the rotated PDF. This allows the user to select a file to save the PDF they chose to rotate! save_title = "Save the rotated PDF as..." # has the user choose a save title file_type = "*.pdf" # sets the file type to be saved output_path = gui.filesavebox(title=save_title, default=file_type) # sets the output path # 5. If the user tries to save with the same name as the input file: while input_path == output_path: # while statement for input path not being equal to output path # - Alert the user with a message box that this is not allowed. gui.msgbox(msg="Cannot overwrite original file!") # creates message box in gui # - Return to step 4. output_path = gui.filesavebox(title=save_title, default=file_type) # reverts to step 4, sets the output path # 6. If the user cancels the file save dialog, then exit the program. if output_path is None: # if statement for output path being 'none' exit() # exits the file # 7. Perform the page rotation: # - Open the selected PDF. input_file = PdfFileReader(input_path) # sets input file and has it read output_pdf = PdfFileWriter() # sets output pdf and has it written # - Rotate all the pages. for page in input_file.pages: # has the file input and pages accounted for page = page.rotateClockwise(degrees) # rotates the pages clockwise output_pdf.addPage(page) # sets the pdf to the output with rotated pages # - Save the rotated PDF to the selected file. with open(output_path, "wb") as output_file: # opens the output path and file output_pdf.write(output_file) # writes to the output file and saves rotated
Hello, I am experimenting with Python. There is a lot of # comments in the code to help me understand it better, but there is an error at line 52 with the input_file PdfFileReader and I do not know how to fix the code. Can you help me?
# Import easygui and pypdf2
import easygui as gui # imports easygui
from PyPDF2 import PdfFileReader, PdfFileWriter # imports PyPDF2
# 1. Display file selection dialog for opening a PDF file. This allows the user to select a PDF that they can rotate!
input_path = gui.fileopenbox( # sets the input path and opens a gui box
title="Select a PDF to rotate...", # sets title
default="*.pdf" # sets the default file type
)
# 2. If user cancels the dialog, exit the program. The program will automatically do this if the user cancels!
if input_path is None: # if statement for input path being 'none'
exit() # exits the file
# 3. Ask user to select one of 90, 180, or 270 degrees. This is how much they will rotate their selected PDF by!
choices = ("90", "180", "270") # degree choices for rotating a PDF
degrees = gui.buttonbox( # creates buttonbox gui for degrees
msg="Rotate the PDF clockwise by how many degrees?", # sets the message for the box
title="Choose rotation...", # sets the title for the box
choices=choices, # sets the choices
) # closes the function
# 4. Display a file selection dialog for saving the rotated PDF. This allows the user to select a file to save the PDF they chose to rotate!
save_title = "Save the rotated PDF as..." # has the user choose a save title
file_type = "*.pdf" # sets the file type to be saved
output_path = gui.filesavebox(title=save_title, default=file_type) # sets the output path
# 5. If the user tries to save with the same name as the input file:
while input_path == output_path: # while statement for input path not being equal to output path
# - Alert the user with a message box that this is not allowed.
gui.msgbox(msg="Cannot overwrite original file!") # creates message box in gui
# - Return to step 4.
output_path = gui.filesavebox(title=save_title, default=file_type) # reverts to step 4, sets the output path
# 6. If the user cancels the file save dialog, then exit the program.
if output_path is None: # if statement for output path being 'none'
exit() # exits the file
# 7. Perform the page rotation:
# - Open the selected PDF.
input_file = PdfFileReader(input_path) # sets input file and has it read
output_pdf = PdfFileWriter() # sets output pdf and has it written
# - Rotate all the pages.
for page in input_file.pages: # has the file input and pages accounted for
page = page.rotateClockwise(degrees) # rotates the pages clockwise
output_pdf.addPage(page) # sets the pdf to the output with rotated pages
# - Save the rotated PDF to the selected file.
with open(output_path, "wb") as output_file: # opens the output path and file
output_pdf.write(output_file) # writes to the output file and saves rotated

Step by step
Solved in 3 steps

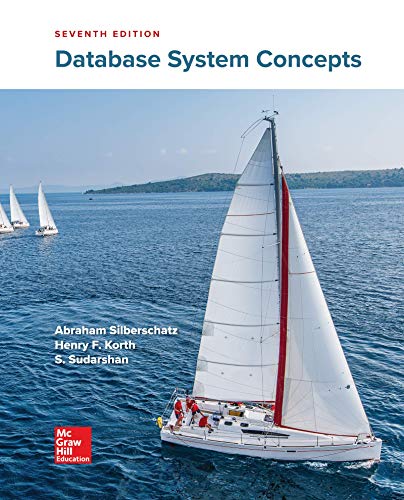
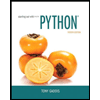
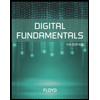
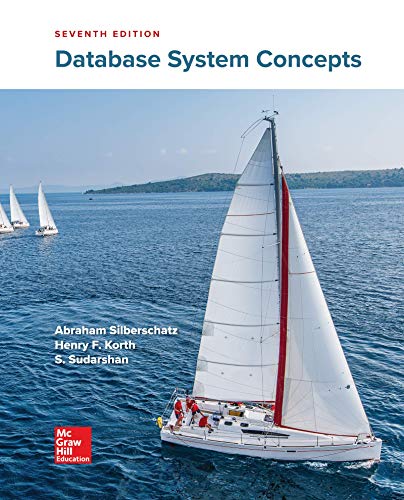
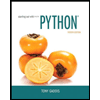
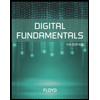
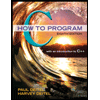
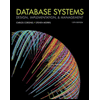
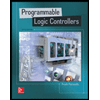