My final python turtle code doesnt want to run, there is no error appearing, and there's no GUI appearing, requesting inputs for amount of lakes, mountains, and rivers, let alone opening python turtle. Help making it run?My code: import turtleimport randomfrom tkinter import Tk, Label, Entry, Button, Scale def get_user_input(): """ Creates a GUI using Tkinter to obtain user input for the number of lakes, mountains, and rivers. Returns: A tuple containing the number of lakes, mountains, and rivers (int, int, int). """ window = Tk() window.title("Island Generator Settings") lake_label = Label(window, text="Number of Lakes:") lake_label.grid(row=0, column=0) lake_entry = Entry(window) lake_entry.grid(row=0, column=1) mountain_label = Label(window, text="Number of Mountains:") mountain_label.grid(row=1, column=0) mountain_entry = Entry(window) mountain_entry.grid(row=1, column=1) river_label = Label(window, text="Number of Rivers:") river_label.grid(row=2, column=0) river_entry = Entry(window) river_entry.grid(row=2, column=1) size_scale_label = Label(window, text="Island Size (Scale)") size_scale_label.grid(row=3, column=0) size_scale = Scale(window, from_=1, to_=3, orient='horizontal', resolution=0.1) size_scale.set(1) # Default size multiplier of 1 size_scale.grid(row=3, column=1, columnspan=2) def handle_close(): window.destroy() button = Button(window, text="Generate Island", command=handle_close) button.grid(row=4, column=0, columnspan=3) window.mainloop() num_lakes = int(lake_entry.get()) if lake_entry.get() else 0 num_mountains = int(mountain_entry.get()) if mountain_entry.get() else 0 num_rivers = int(river_entry.get()) if river_entry.get() else 0 size_multiplier = size_scale.get() return num_lakes, num_mountains, num_rivers, size_multiplier # Define colorsocean = "#000066"sand = "#ffff66"grass = "#00cc00"lake = "#0066ff"mountain_color = "#808080" # Gray color for mountains def draw_circle(radius, line_color, fill_color): """ Draws a circle with the specified radius, line color, and fill color. Args: radius (int): The radius of the circle. line_color (str): The color of the circle's outline. fill_color (str): The color to fill the circle. """ my_turtle.color(line_color) my_turtle.fillcolor(fill_color) my_turtle.begin_fill() my_turtle.circle(radius) my_turtle.end_fill() def move_turtle(x, y): """ Moves the turtle to the specified coordinates without drawing a line. Args: x (int): The x-coordinate of the destination. y (int): The y-coordinate of the destination. """ my_turtle.penup() my_turtle.goto(x, y) my_turtle.pendown() def draw_lake(): """ Draws a random lake circle within the boundaries of the landmass. Increases the size of the lake based on the size multiplier. """ x_limit = int(150 * size_multiplier) // 2 - 30 * size_multiplier # Adjusted for lake size y_limit = int(120 * size_multiplier) // 2 - 30 * size_multiplier # Adjusted for lake size x = random.randint(-x_limit, x_limit) y = random.randint(-y_limit, y_limit) move_turtle(x, y)
My final python turtle code doesnt want to run, there is no error appearing, and there's no GUI appearing, requesting inputs for amount of lakes, mountains, and rivers, let alone opening python turtle. Help making it run?
My code:
import turtle
import random
from tkinter import Tk, Label, Entry, Button, Scale
def get_user_input():
"""
Creates a GUI using Tkinter to obtain user input for the number of lakes, mountains, and rivers.
Returns:
A tuple containing the number of lakes, mountains, and rivers (int, int, int).
"""
window = Tk()
window.title("Island Generator Settings")
lake_label = Label(window, text="Number of Lakes:")
lake_label.grid(row=0, column=0)
lake_entry = Entry(window)
lake_entry.grid(row=0, column=1)
mountain_label = Label(window, text="Number of Mountains:")
mountain_label.grid(row=1, column=0)
mountain_entry = Entry(window)
mountain_entry.grid(row=1, column=1)
river_label = Label(window, text="Number of Rivers:")
river_label.grid(row=2, column=0)
river_entry = Entry(window)
river_entry.grid(row=2, column=1)
size_scale_label = Label(window, text="Island Size (Scale)")
size_scale_label.grid(row=3, column=0)
size_scale = Scale(window, from_=1, to_=3, orient='horizontal', resolution=0.1)
size_scale.set(1) # Default size multiplier of 1
size_scale.grid(row=3, column=1, columnspan=2)
def handle_close():
window.destroy()
button = Button(window, text="Generate Island", command=handle_close)
button.grid(row=4, column=0, columnspan=3)
window.mainloop()
num_lakes = int(lake_entry.get()) if lake_entry.get() else 0
num_mountains = int(mountain_entry.get()) if mountain_entry.get() else 0
num_rivers = int(river_entry.get()) if river_entry.get() else 0
size_multiplier = size_scale.get()
return num_lakes, num_mountains, num_rivers, size_multiplier
# Define colors
ocean = "#000066"
sand = "#ffff66"
grass = "#00cc00"
lake = "#0066ff"
mountain_color = "#808080" # Gray color for mountains
def draw_circle(radius, line_color, fill_color):
"""
Draws a circle with the specified radius, line color, and fill color.
Args:
radius (int): The radius of the circle.
line_color (str): The color of the circle's outline.
fill_color (str): The color to fill the circle.
"""
my_turtle.color(line_color)
my_turtle.fillcolor(fill_color)
my_turtle.begin_fill()
my_turtle.circle(radius)
my_turtle.end_fill()
def move_turtle(x, y):
"""
Moves the turtle to the specified coordinates without drawing a line.
Args:
x (int): The x-coordinate of the destination.
y (int): The y-coordinate of the destination.
"""
my_turtle.penup()
my_turtle.goto(x, y)
my_turtle.pendown()
def draw_lake():
"""
Draws a random lake circle within the boundaries of the landmass.
Increases the size of the lake based on the size multiplier.
"""
x_limit = int(150 * size_multiplier) // 2 - 30 * size_multiplier # Adjusted for lake size
y_limit = int(120 * size_multiplier) // 2 - 30 * size_multiplier # Adjusted for lake size
x = random.randint(-x_limit, x_limit)
y = random.randint(-y_limit, y_limit)
move_turtle(x, y)

Step by step
Solved in 2 steps

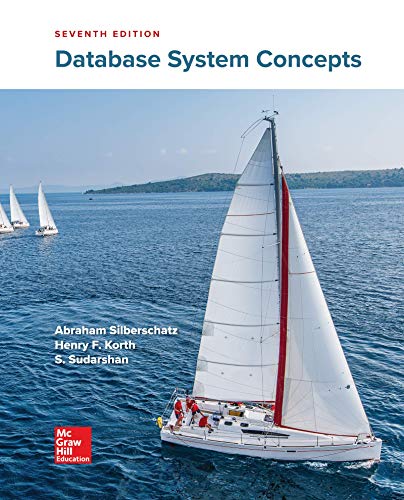
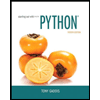
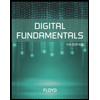
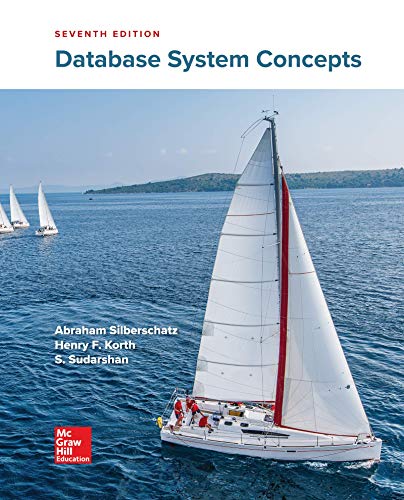
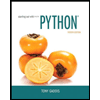
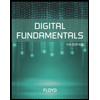
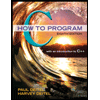
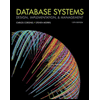
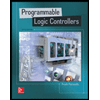