Hello, can you please check and debug this code? For some reason I can't get it to compile. #include #include #include #include #include #include #include #include #define BUFSIZE 1024 typedef struct { char word[42]; unsigned count; } WORD_T; WORD_T *words = NULL; size_t total_words = 0; void print_and_free(WORD_T*, size_t, char*); int comparator(const void *a, const void *b) { constchar *a_ptr = (constchar*) a; WORD_T *b_ptr = (WORD_T) b; //set a_ptr (a pointer) and b_ptr returnstrcmp(a_ptr, b_ptr->word); } int main(int argc, char **argv) { if (argc != 2) return -1; char *infile = argv[1]; int fd = open(infile, O_RDONLY); //open as read only if (fd == -1) return -2; char buf[BUFSIZE]; size_t buf_len = 0; char *stringp = NULL; size_t xread; //times read while ((xread = read(fd, buf +buf_len, BUFSIZE - buf_len)) > 0) { buf_len += xread; stringp = buf; if (buf[buf_len - 1] != '\n' && buf[buf_len -1] != '') // if it's not a space or newline, i.e. partway through a word { size_t i; for (i = buf_len -1; i > 0; --i) { if (buf[i] == '\n' || buf[i] == '') //couldn't figure out why 'or' didn't work, C makes you use '||' { break; } } size_t almost = bif_len -i -1; //this should use memmove to move the last partial word to the beggining of the buffer memmove(buf, buf +i + 1, almost); buf_len = almost; stringp = buf; } while ((stringp = strsep(&stringp, "\n")) != NULL) { if (stringp[0] == '\0') continue; WORD_T *result = (WORD_T *) lfind(&stringp, words, &total_words, sizeof(WORD_T*), comparator); //searches for the word in an array using comparator from earlier if (result != NULL) { result -> count ++; //increment count if word isfound } else//otherwise add a new word to the array { words = realloc(words, sizeof(WORD_T) * (total_words + 1)); strcopy(words[total_words].word, stringp, 42); //42 because thats the size of the array, this will prevent overflows word[total_words].count = 1; total_words ++ ; //because now we have one more word total } } } print_and_free(words, total_words, infile); // TODO: close the file fclose(infile); return0; } void print_and_free(WORD_T *words, size_t total_words, char *infile) { int sum = 0; for (int i = 0; i < total_words; ++i) { if (words[i].count > 1) printf("%s: %u\n", words[i].word, words[i].count); sum += words[i].count; } printf("\n%d%s\n", sum, infile); printf("\n"); free(words); }
Hello, can you please check and debug this code? For some reason I can't get it to compile. #include #include #include #include #include #include #include #include #define BUFSIZE 1024 typedef struct { char word[42]; unsigned count; } WORD_T; WORD_T *words = NULL; size_t total_words = 0; void print_and_free(WORD_T*, size_t, char*); int comparator(const void *a, const void *b) { constchar *a_ptr = (constchar*) a; WORD_T *b_ptr = (WORD_T) b; //set a_ptr (a pointer) and b_ptr returnstrcmp(a_ptr, b_ptr->word); } int main(int argc, char **argv) { if (argc != 2) return -1; char *infile = argv[1]; int fd = open(infile, O_RDONLY); //open as read only if (fd == -1) return -2; char buf[BUFSIZE]; size_t buf_len = 0; char *stringp = NULL; size_t xread; //times read while ((xread = read(fd, buf +buf_len, BUFSIZE - buf_len)) > 0) { buf_len += xread; stringp = buf; if (buf[buf_len - 1] != '\n' && buf[buf_len -1] != '') // if it's not a space or newline, i.e. partway through a word { size_t i; for (i = buf_len -1; i > 0; --i) { if (buf[i] == '\n' || buf[i] == '') //couldn't figure out why 'or' didn't work, C makes you use '||' { break; } } size_t almost = bif_len -i -1; //this should use memmove to move the last partial word to the beggining of the buffer memmove(buf, buf +i + 1, almost); buf_len = almost; stringp = buf; } while ((stringp = strsep(&stringp, "\n")) != NULL) { if (stringp[0] == '\0') continue; WORD_T *result = (WORD_T *) lfind(&stringp, words, &total_words, sizeof(WORD_T*), comparator); //searches for the word in an array using comparator from earlier if (result != NULL) { result -> count ++; //increment count if word isfound } else//otherwise add a new word to the array { words = realloc(words, sizeof(WORD_T) * (total_words + 1)); strcopy(words[total_words].word, stringp, 42); //42 because thats the size of the array, this will prevent overflows word[total_words].count = 1; total_words ++ ; //because now we have one more word total } } } print_and_free(words, total_words, infile); // TODO: close the file fclose(infile); return0; } void print_and_free(WORD_T *words, size_t total_words, char *infile) { int sum = 0; for (int i = 0; i < total_words; ++i) { if (words[i].count > 1) printf("%s: %u\n", words[i].word, words[i].count); sum += words[i].count; } printf("\n%d%s\n", sum, infile); printf("\n"); free(words); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hello, can you please check and debug this code? For some reason I can't get it to compile.
#include <fcntl.h> | |
#include <search.h> | |
#include <stdio.h> | |
#include <stdlib.h> | |
#include <string.h> | |
#include <sys/types.h> | |
#include <sys/stat.h> | |
#include <unistd.h> | |
#define BUFSIZE 1024 | |
typedef struct { | |
char word[42]; | |
unsigned count; | |
} WORD_T; | |
WORD_T *words = NULL; | |
size_t total_words = 0; | |
void print_and_free(WORD_T*, size_t, char*); | |
int comparator(const void *a, const void *b) { | |
constchar *a_ptr = (constchar*) a; | |
WORD_T *b_ptr = (WORD_T) b; //set a_ptr (a pointer) and b_ptr | |
returnstrcmp(a_ptr, b_ptr->word); | |
} | |
int main(int argc, char **argv) { | |
if (argc != 2) return -1; | |
char *infile = argv[1]; | |
int fd = open(infile, O_RDONLY); //open as read only | |
if (fd == -1) return -2; | |
char buf[BUFSIZE]; | |
size_t buf_len = 0; | |
char *stringp = NULL; | |
size_t xread; //times read | |
while ((xread = read(fd, buf +buf_len, BUFSIZE - buf_len)) > 0) | |
{ | |
buf_len += xread; | |
stringp = buf; | |
if (buf[buf_len - 1] != '\n' && buf[buf_len -1] != '') // if it's not a space or newline, i.e. partway through a word | |
{ | |
size_t i; | |
for (i = buf_len -1; | |
i > 0; | |
--i) | |
{ | |
if (buf[i] == '\n' || buf[i] == '') //couldn't figure out why 'or' didn't work, C makes you use '||' | |
{ | |
break; | |
} | |
} | |
size_t almost = bif_len -i -1; //this should use memmove to move the last partial word to the beggining of the buffer | |
memmove(buf, buf +i + 1, almost); | |
buf_len = almost; | |
stringp = buf; | |
} | |
while ((stringp = strsep(&stringp, "\n")) != NULL) | |
{ | |
if (stringp[0] == '\0') continue; | |
WORD_T *result = (WORD_T *) lfind(&stringp, words, &total_words, sizeof(WORD_T*), comparator); | |
//searches for the word in an array using comparator from earlier | |
if (result != NULL) | |
{ | |
result -> count ++; //increment count if word isfound | |
} | |
else//otherwise add a new word to the array | |
{ | |
words = realloc(words, sizeof(WORD_T) * (total_words + 1)); | |
strcopy(words[total_words].word, stringp, 42); //42 because thats the size of the array, this will prevent overflows | |
word[total_words].count = 1; | |
total_words ++ ; //because now we have one more word total | |
} | |
} | |
} | |
print_and_free(words, total_words, infile); | |
// TODO: close the file | |
fclose(infile); | |
return0; | |
} | |
void print_and_free(WORD_T *words, size_t total_words, char *infile) { | |
int sum = 0; | |
for (int i = 0; i < total_words; ++i) { | |
if (words[i].count > 1) | |
printf("%s: %u\n", words[i].word, words[i].count); | |
sum += words[i].count; | |
} | |
printf("\n%d%s\n", sum, infile); | |
printf("\n"); | |
free(words); | |
} |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
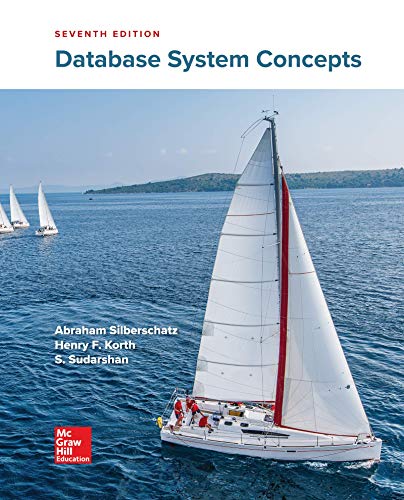
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
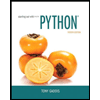
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
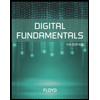
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
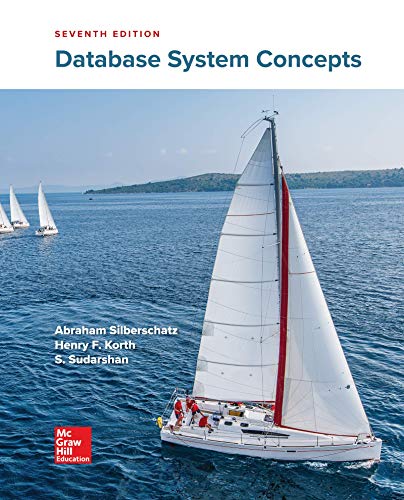
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
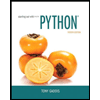
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
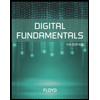
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
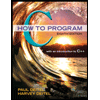
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
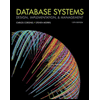
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
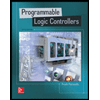
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education