HamSupper.java 个 import java.util.Arrays; Formatting (-1): There's a lack of comments in areas that should be better explained like the specialized method. Part 1 (-2): See marker comment below. Incorrect comparisons are being done for the serial numbers. Testing (-2.5): - Not tested with the given input file. public class HamSupper { public static void main(String[] args) { // Input list of serial numbers List serialNumbers = Arrays.asList("41360114", "48648110", "11036224" "83620684", // Generalized code 10 11 import java.util.HashMap; 12 import java.util.List; 13 import java.util.Map; 14 15 /* MARKER-COMMENT: 16 * Submission Guidelines (-1): 17 * - Missing javadoc comments on methods. 18 * 19 * 20 * 21 * 22 23 * 24 * 25 * 26 27 28 29 30 31 32 33 34 35 36 37 38 // Specialized code 39 40 41 42 43 } 44 45 46 47 48 49 50 51 52 53 54 55 56 Map generalized Result = computeCleaning Sums General (serialNumbers); System.out.println("Generalized Code:"); System.out.println("Sum to be cleaned in dish washer: " + generalized Result.get(true)); System.out.println("Sum to be cleaned in sink: " + generalized Result.get(false)); = computeCleaning Sums Special (serialNumbers); Map specialized Result System.out.println("\nSpecialized Code:"); System.out.println("Sum to be cleaned in dish washer: " + specialized Result.get(true)); System.out.println("Sum to be cleaned in sink: " + specialized Result.get(false)); // Generalized code to compute cleaning sums public static Map computeCleaning Sums General (List serialNumbers) { long dishwasherSum = 0; long sinkSum = 0; for (int i = 0; i < serialNumbers.size(); i++) { for (int j i + 1; j < serialNumbers.size(); j++) { int hammingDistance = "11806064" "22 computeHamming Distance (serialNumbers.get(i), serialNumbers.get(j)); if (hammingDistance serialNumbers.get(i).length()) { ' /* MARKER-COMMENT: The serial number needs to have a hamming distance of 8 when compared * to ALL the other serial numbers. In other words, if the hamming distance between itself, and * any other serial number is less than 8, than it should be cleaned in the dish washer. */ 57 58 59 60 61 64 2262832 65 66 67 68 } sinkSum += Long. parseLong(serialNumbers.get(i)); sinkSum += Long. parseLong(serialNumbers.get(j)); } else { } dishwasherSum += Long. parseLong (serialNumbers.get(i)); dishwasherSum += Long. parseLong (serialNumbers.get(j)); Map result = new HashMap<>(); result.put(true, dishwasherSum); result.put(false, sinkSum); return result; // Helper method to compute Hamming distance between two strings public static int computeHamming Distance (String sl, String s2) { int distance = 0; for (int i = 0; i < s1.length(); i++) { if (s1.charAt(i) != s2.charAt(i)) { distance++; 69 70 } 71 72 73 74 75 76 77 78 } 79 80 return distance; 81 } 82 83 84 85 86 87 88 89 90 91 92 93 } 94 } 95 96 97 98 99 100 101 102 } 103 104 // Specialized code to compute cleaning sums with linear time complexity public static Map computeCleaning Sums Special (List serialNumbers) { long dishwasherSum = 0; long sinkSum = 0; int[] countOfHamming Distance = new int[serialNumbers.get(0).length() + 1]; for (String serial Number : serialNumbers) { for (String otherSerialNumber: serialNumbers) { int hamming Distance computeHamming Distance (serialNumber, otherSerialNumber); countOfHamming Distance [hamming Distance]++; 1) / 2; 1) / 2; for (int i = 0; i < if (i == countOfHamming Distance.length; i++) { serialNumbers.get(0).length()) { sinkSum += countOfHammingDistance [i] ✶ (countOfHamming Distance [i] } else { } dishwasherSum += countOfHammingDistance[i] ✶ (countOfHammingDistance[i] Map result = new HashMap<>();
HamSupper.java 个 import java.util.Arrays; Formatting (-1): There's a lack of comments in areas that should be better explained like the specialized method. Part 1 (-2): See marker comment below. Incorrect comparisons are being done for the serial numbers. Testing (-2.5): - Not tested with the given input file. public class HamSupper { public static void main(String[] args) { // Input list of serial numbers List serialNumbers = Arrays.asList("41360114", "48648110", "11036224" "83620684", // Generalized code 10 11 import java.util.HashMap; 12 import java.util.List; 13 import java.util.Map; 14 15 /* MARKER-COMMENT: 16 * Submission Guidelines (-1): 17 * - Missing javadoc comments on methods. 18 * 19 * 20 * 21 * 22 23 * 24 * 25 * 26 27 28 29 30 31 32 33 34 35 36 37 38 // Specialized code 39 40 41 42 43 } 44 45 46 47 48 49 50 51 52 53 54 55 56 Map generalized Result = computeCleaning Sums General (serialNumbers); System.out.println("Generalized Code:"); System.out.println("Sum to be cleaned in dish washer: " + generalized Result.get(true)); System.out.println("Sum to be cleaned in sink: " + generalized Result.get(false)); = computeCleaning Sums Special (serialNumbers); Map specialized Result System.out.println("\nSpecialized Code:"); System.out.println("Sum to be cleaned in dish washer: " + specialized Result.get(true)); System.out.println("Sum to be cleaned in sink: " + specialized Result.get(false)); // Generalized code to compute cleaning sums public static Map computeCleaning Sums General (List serialNumbers) { long dishwasherSum = 0; long sinkSum = 0; for (int i = 0; i < serialNumbers.size(); i++) { for (int j i + 1; j < serialNumbers.size(); j++) { int hammingDistance = "11806064" "22 computeHamming Distance (serialNumbers.get(i), serialNumbers.get(j)); if (hammingDistance serialNumbers.get(i).length()) { ' /* MARKER-COMMENT: The serial number needs to have a hamming distance of 8 when compared * to ALL the other serial numbers. In other words, if the hamming distance between itself, and * any other serial number is less than 8, than it should be cleaned in the dish washer. */ 57 58 59 60 61 64 2262832 65 66 67 68 } sinkSum += Long. parseLong(serialNumbers.get(i)); sinkSum += Long. parseLong(serialNumbers.get(j)); } else { } dishwasherSum += Long. parseLong (serialNumbers.get(i)); dishwasherSum += Long. parseLong (serialNumbers.get(j)); Map result = new HashMap<>(); result.put(true, dishwasherSum); result.put(false, sinkSum); return result; // Helper method to compute Hamming distance between two strings public static int computeHamming Distance (String sl, String s2) { int distance = 0; for (int i = 0; i < s1.length(); i++) { if (s1.charAt(i) != s2.charAt(i)) { distance++; 69 70 } 71 72 73 74 75 76 77 78 } 79 80 return distance; 81 } 82 83 84 85 86 87 88 89 90 91 92 93 } 94 } 95 96 97 98 99 100 101 102 } 103 104 // Specialized code to compute cleaning sums with linear time complexity public static Map computeCleaning Sums Special (List serialNumbers) { long dishwasherSum = 0; long sinkSum = 0; int[] countOfHamming Distance = new int[serialNumbers.get(0).length() + 1]; for (String serial Number : serialNumbers) { for (String otherSerialNumber: serialNumbers) { int hamming Distance computeHamming Distance (serialNumber, otherSerialNumber); countOfHamming Distance [hamming Distance]++; 1) / 2; 1) / 2; for (int i = 0; i < if (i == countOfHamming Distance.length; i++) { serialNumbers.get(0).length()) { sinkSum += countOfHammingDistance [i] ✶ (countOfHamming Distance [i] } else { } dishwasherSum += countOfHammingDistance[i] ✶ (countOfHammingDistance[i] Map result = new HashMap<>();
Chapter14: Files And Streams
Section: Chapter Questions
Problem 17RQ
Related questions
Question
Could you fix this? My marker has commented, What's missing? The input list is the link below.
https://gmierzwinski.github.io/bishops/cs321/resources/CS321_Assignment_1_Input.txt
result.put(true, dishwasherSum);
result.put(false, sinkSum);
return result;
}
}
![HamSupper.java
个
import java.util.Arrays;
Formatting (-1):
There's a lack of comments in areas that should be better explained like the
specialized method.
Part 1 (-2):
See marker comment below. Incorrect comparisons are being done for the serial numbers.
Testing (-2.5):
- Not tested with the given input file.
public class HamSupper {
public static void main(String[] args) {
// Input list of serial numbers
List<String> serialNumbers = Arrays.asList("41360114", "48648110", "11036224" "83620684",
// Generalized code
10
11
import java.util.HashMap;
12
import java.util.List;
13
import java.util.Map;
14
15
/* MARKER-COMMENT:
16
*
Submission Guidelines (-1):
17
*
- Missing javadoc comments on methods.
18
*
19
*
20
*
21
*
22
23
*
24
*
25
*
26
27
28
29
30
31
32
33
34
35
36
37
38
// Specialized code
39
40
41
42
43
}
44
45
46
47
48
49
50
51
52
53
54
55
56
Map<Boolean, Long> generalized Result = computeCleaning Sums General (serialNumbers);
System.out.println("Generalized Code:");
System.out.println("Sum to be cleaned in dish washer: " + generalized Result.get(true));
System.out.println("Sum to be cleaned in sink: " + generalized Result.get(false));
=
computeCleaning Sums Special (serialNumbers);
Map<Boolean, Long> specialized Result
System.out.println("\nSpecialized Code:");
System.out.println("Sum to be cleaned in dish washer: " + specialized Result.get(true));
System.out.println("Sum to be cleaned in sink: " + specialized Result.get(false));
// Generalized code to compute cleaning sums
public static Map<Boolean, Long> computeCleaning Sums General (List<String> serialNumbers) {
long dishwasherSum = 0;
long sinkSum = 0;
for (int i = 0; i < serialNumbers.size(); i++) {
for (int j i + 1; j < serialNumbers.size(); j++) {
int hammingDistance =
"11806064" "22
computeHamming Distance (serialNumbers.get(i), serialNumbers.get(j));
if (hammingDistance serialNumbers.get(i).length()) {
'
/* MARKER-COMMENT: The serial number needs to have a hamming distance of 8 when compared
* to ALL the other serial numbers. In other words, if the hamming distance between itself, and
* any other serial number is less than 8, than it should be cleaned in the dish washer. */](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdc7a8be5-c84e-4c07-8de6-6565e3c318c8%2Fcbd8aaa1-569d-4021-9dcb-da83d965e249%2Fpkru03_processed.png&w=3840&q=75)
Transcribed Image Text:HamSupper.java
个
import java.util.Arrays;
Formatting (-1):
There's a lack of comments in areas that should be better explained like the
specialized method.
Part 1 (-2):
See marker comment below. Incorrect comparisons are being done for the serial numbers.
Testing (-2.5):
- Not tested with the given input file.
public class HamSupper {
public static void main(String[] args) {
// Input list of serial numbers
List<String> serialNumbers = Arrays.asList("41360114", "48648110", "11036224" "83620684",
// Generalized code
10
11
import java.util.HashMap;
12
import java.util.List;
13
import java.util.Map;
14
15
/* MARKER-COMMENT:
16
*
Submission Guidelines (-1):
17
*
- Missing javadoc comments on methods.
18
*
19
*
20
*
21
*
22
23
*
24
*
25
*
26
27
28
29
30
31
32
33
34
35
36
37
38
// Specialized code
39
40
41
42
43
}
44
45
46
47
48
49
50
51
52
53
54
55
56
Map<Boolean, Long> generalized Result = computeCleaning Sums General (serialNumbers);
System.out.println("Generalized Code:");
System.out.println("Sum to be cleaned in dish washer: " + generalized Result.get(true));
System.out.println("Sum to be cleaned in sink: " + generalized Result.get(false));
=
computeCleaning Sums Special (serialNumbers);
Map<Boolean, Long> specialized Result
System.out.println("\nSpecialized Code:");
System.out.println("Sum to be cleaned in dish washer: " + specialized Result.get(true));
System.out.println("Sum to be cleaned in sink: " + specialized Result.get(false));
// Generalized code to compute cleaning sums
public static Map<Boolean, Long> computeCleaning Sums General (List<String> serialNumbers) {
long dishwasherSum = 0;
long sinkSum = 0;
for (int i = 0; i < serialNumbers.size(); i++) {
for (int j i + 1; j < serialNumbers.size(); j++) {
int hammingDistance =
"11806064" "22
computeHamming Distance (serialNumbers.get(i), serialNumbers.get(j));
if (hammingDistance serialNumbers.get(i).length()) {
'
/* MARKER-COMMENT: The serial number needs to have a hamming distance of 8 when compared
* to ALL the other serial numbers. In other words, if the hamming distance between itself, and
* any other serial number is less than 8, than it should be cleaned in the dish washer. */
![57
58
59
60
61
64
2262832
65
66
67
68
}
sinkSum += Long. parseLong(serialNumbers.get(i));
sinkSum += Long. parseLong(serialNumbers.get(j));
} else {
}
dishwasherSum += Long. parseLong (serialNumbers.get(i));
dishwasherSum += Long. parseLong (serialNumbers.get(j));
Map<Boolean, Long> result = new HashMap<>();
result.put(true, dishwasherSum);
result.put(false, sinkSum);
return result;
// Helper method to compute Hamming distance between two strings
public static int computeHamming Distance (String sl, String s2) {
int distance = 0;
for (int i = 0; i < s1.length(); i++) {
if (s1.charAt(i) != s2.charAt(i)) {
distance++;
69
70
}
71
72
73
74
75
76
77
78
}
79
80
return distance;
81
}
82
83
84
85
86
87
88
89
90
91
92
93
}
94
}
95
96
97
98
99
100
101
102
}
103
104
// Specialized code to compute cleaning sums with linear time complexity
public static Map<Boolean, Long> computeCleaning Sums Special (List<String> serialNumbers) {
long dishwasherSum = 0;
long sinkSum = 0;
int[] countOfHamming Distance = new int[serialNumbers.get(0).length() + 1];
for (String serial Number : serialNumbers) {
for (String otherSerialNumber: serialNumbers) {
int hamming Distance computeHamming Distance (serialNumber, otherSerialNumber);
countOfHamming Distance [hamming Distance]++;
1) / 2;
1) / 2;
for (int i = 0; i <
if (i
==
countOfHamming Distance.length; i++) {
serialNumbers.get(0).length()) {
sinkSum += countOfHammingDistance [i] ✶ (countOfHamming Distance [i]
} else {
}
dishwasherSum += countOfHammingDistance[i] ✶ (countOfHammingDistance[i]
Map<Boolean, Long> result = new HashMap<>();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdc7a8be5-c84e-4c07-8de6-6565e3c318c8%2Fcbd8aaa1-569d-4021-9dcb-da83d965e249%2F5ia5jlm_processed.png&w=3840&q=75)
Transcribed Image Text:57
58
59
60
61
64
2262832
65
66
67
68
}
sinkSum += Long. parseLong(serialNumbers.get(i));
sinkSum += Long. parseLong(serialNumbers.get(j));
} else {
}
dishwasherSum += Long. parseLong (serialNumbers.get(i));
dishwasherSum += Long. parseLong (serialNumbers.get(j));
Map<Boolean, Long> result = new HashMap<>();
result.put(true, dishwasherSum);
result.put(false, sinkSum);
return result;
// Helper method to compute Hamming distance between two strings
public static int computeHamming Distance (String sl, String s2) {
int distance = 0;
for (int i = 0; i < s1.length(); i++) {
if (s1.charAt(i) != s2.charAt(i)) {
distance++;
69
70
}
71
72
73
74
75
76
77
78
}
79
80
return distance;
81
}
82
83
84
85
86
87
88
89
90
91
92
93
}
94
}
95
96
97
98
99
100
101
102
}
103
104
// Specialized code to compute cleaning sums with linear time complexity
public static Map<Boolean, Long> computeCleaning Sums Special (List<String> serialNumbers) {
long dishwasherSum = 0;
long sinkSum = 0;
int[] countOfHamming Distance = new int[serialNumbers.get(0).length() + 1];
for (String serial Number : serialNumbers) {
for (String otherSerialNumber: serialNumbers) {
int hamming Distance computeHamming Distance (serialNumber, otherSerialNumber);
countOfHamming Distance [hamming Distance]++;
1) / 2;
1) / 2;
for (int i = 0; i <
if (i
==
countOfHamming Distance.length; i++) {
serialNumbers.get(0).length()) {
sinkSum += countOfHammingDistance [i] ✶ (countOfHamming Distance [i]
} else {
}
dishwasherSum += countOfHammingDistance[i] ✶ (countOfHammingDistance[i]
Map<Boolean, Long> result = new HashMap<>();
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
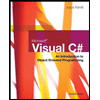
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
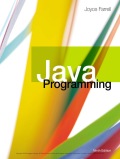
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
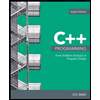
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
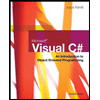
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
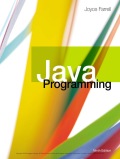
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
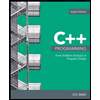
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
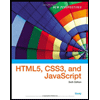
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
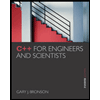
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr