Given the C++ code: // toh.cpp #include #include using namespace std; int main() { vector t[3]; int n; cout << "Enter the number of rings: "; cin >> n; int from = 0; int candidate = 1; int to, move = 0; if ( n % 2 == 0) to = 2; // (from + to)%mod3, counter clockwise else to = 1; // (from + to)%mod3, clockwise for ( int i = n + 1 ; i >= 1; i-- ) t[0].push_back(i); t[1].push_back(n+1); t[2].push_back(n+1); if ( n % 2 == 0 ) { while ( t[1].size() < (n+1) ) { cout << "move " << ++move << ":" << " Move candidate " << candidate; cout << " from tower " << (char) (from + 'A'); cout << " to tower " << (char)(to + 'A') << endl; t[to].push_back(t[from].back()); t[from].pop_back(); if (t[(to+2)%3].back() < t[(to+1)%3].back()) from = (to + 2)%3; else from = (to + 1)%3; if (t[(from)%3].back() < t[(from+2)%3].back()) to = (from + 2)%3; else to = (from + 1)%3; candidate = t[from].back(); } return 0; } else { while ( t[1].size() < ( n+1 ) ) { cout << "move " << ++move << ":" << " Move candidate " << candidate; cout << " from tower " << (char) (from + 'A'); cout << " to tower " << (char)(to + 'A') << endl; t[to].push_back(t[from].back()); t[from].pop_back(); if (t[(to+1)%3].back() < t[(to+2)%3].back()) from = ( to + 1 )%3; else from = ( to + 2 )%3; //cout << "from = " << from << endl; if (t[(from)%3].back() < t[(from+1)%3].back()) to = (from + 1)%3; else to = (from + 2)%3; //cout << "to = " << to <
Given the C++ code:
// toh.cpp
#include <iostream>
#include <
using namespace std;
int main() {
vector<int> t[3];
int n;
cout << "Enter the number of rings: ";
cin >> n;
int from = 0;
int candidate = 1;
int to, move = 0;
if ( n % 2 == 0)
to = 2; // (from + to)%mod3, counter clockwise
else
to = 1; // (from + to)%mod3, clockwise
for ( int i = n + 1 ; i >= 1; i-- )
t[0].push_back(i);
t[1].push_back(n+1);
t[2].push_back(n+1);
if ( n % 2 == 0 )
{
while ( t[1].size() < (n+1) ) {
cout << "move " << ++move << ":" << " Move candidate " << candidate;
cout << " from tower " << (char) (from + 'A');
cout << " to tower " << (char)(to + 'A') << endl;
t[to].push_back(t[from].back());
t[from].pop_back();
if (t[(to+2)%3].back() < t[(to+1)%3].back())
from = (to + 2)%3;
else
from = (to + 1)%3;
if (t[(from)%3].back() < t[(from+2)%3].back())
to = (from + 2)%3;
else
to = (from + 1)%3;
candidate = t[from].back();
}
return 0;
} else {
while ( t[1].size() < ( n+1 ) ) {
cout << "move " << ++move << ":" << " Move candidate " << candidate;
cout << " from tower " << (char) (from + 'A');
cout << " to tower " << (char)(to + 'A') << endl;
t[to].push_back(t[from].back());
t[from].pop_back();
if (t[(to+1)%3].back() < t[(to+2)%3].back())
from = ( to + 1 )%3;
else
from = ( to + 2 )%3;
//cout << "from = " << from << endl;
if (t[(from)%3].back() < t[(from+1)%3].back())
to = (from + 1)%3;
else
to = (from + 2)%3;
//cout << "to = " << to <<endl;
candidate = t[from].back();
}
return 0;
}
return 0;
}
Given the while loop:
while ( t[1].size() < ( n+1 ) ) {
cout << "move " << ++move << ":" << " Move candidate " << candidate;
cout << " from tower " << (char) (from + 'A');
cout << " to tower " << (char)(to + 'A') << endl;
t[to].push_back(t[from].back());
t[from].pop_back();
if (t[(to+1)%3].back() < t[(to+2)%3].back())
from = ( to + 1 )%3;
else
from = ( to + 2 )%3;
//cout << "from = " << from << endl;
if (t[(from)%3].back() < t[(from+1)%3].back())
to = (from + 1)%3;
else
to = (from + 2)%3;
//cout << "to = " << to <<endl;
candidate = t[from].back();
}
return 0;
Question: Explain what each instruction is doing.

Step by step
Solved in 2 steps

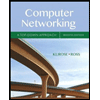
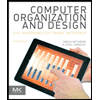
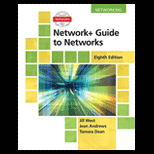
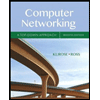
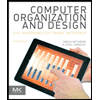
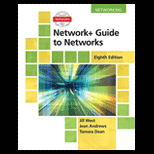
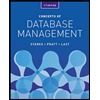
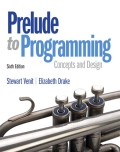
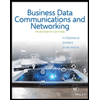