Firstly int is_cycle(*DON'T FORGET TO EDIT THIS LINE*); Implement heap_sort0 function for using Kruskal's MST algorithm. This function also needs heapify0 function. You can add your utility functions as many as you want.Implement Kruskals_MST) function. This function needs another function for detecting the cycles on the graph which name is is_cycle0. You can add your utility functions as many as you want.Write a report that answers the following questions: • What is the complexity of Kruskal's MST algorithm? Show your calculations. • What is the complexity of Heap Sort algorithm? Show your calculations. • What are the differences between Kruskal's and Prim's MST algorithms? • What are the data structures that you used in this assignment and which purposes?
URGENT!!! PLS HELP!! IT HAVE TO BE IN C CODE
{
int source, // DON'T EDIT THIS LINE
destination, // DON'T EDIT THIS LINE
weight; // DON'T EDIT THIS LINE
}EDGE; // DON'T EDIT THIS LINE
typedef struct{
int num_vertices, // DON'T EDIT THIS LINE
num_edges; // DON'T EDIT THIS LINE
EDGE *edges; // DON'T EDIT THIS LINE
}GRAPH; // DON'T EDIT THIS LINE
GRAPH *init_graph(int vertices, int edges); // DON'T EDIT THIS LINE
void fill_graph(GRAPH *graph); // DON'T EDIT THIS LINE
int is_cycle(/*DON'T FORGET TO EDIT THIS LINE*/);
void Kruskals_MST(GRAPH *graph); // DON'T EDIT THIS LINE
int main() {
GRAPH *graph = init_graph(10, 14); // DON'T EDIT THIS LINE
fill_graph(graph); // DON'T EDIT THIS LINE
Kruskals_MST(graph); // DON'T EDIT THIS LINE
return 0;
}
GRAPH *init_graph(int vertices, int edges) {
GRAPH *graph = calloc(1, sizeof(*graph)); // DON'T EDIT THIS LINE
if(graph == NULL) { // DON'T EDIT THIS LINE
printf("Error:"); // DON'T EDIT THIS LINE
exit(EXIT_FAILURE); // DON'T EDIT THIS LINE
}
graph->num_vertices = vertices; // DON'T EDIT THIS LINE
graph->num_edges = edges; // DON'T EDIT THIS LINE
graph->edges = calloc(edges, sizeof(EDGE));// DON'T EDIT THIS LINE
if(graph->edges == NULL){ // DON'T EDIT THIS LINE
printf("Error:"); // DON'T EDIT THIS LINE
exit(EXIT_FAILURE); // DON'T EDIT THIS LINE
}
return graph; // DON'T EDIT THIS LINE
}
void fill_graph(GRAPH *graph){
graph->edges[0].source = 1; // DON'T EDIT THIS LINE
graph->edges[0].destination = 3; // DON'T EDIT THIS LINE
graph->edges[0].weight = 12; // DON'T EDIT THIS LINE
graph->edges[1].source = 1; // DON'T EDIT THIS LINE
graph->edges[1].destination = 2; // DON'T EDIT THIS LINE
graph->edges[1].weight = 4; // DON'T EDIT THIS LINE
graph->edges[2].source = 2; // DON'T EDIT THIS LINE
graph->edges[2].destination = 5; // DON'T EDIT THIS LINE
graph->edges[2].weight = 10; // DON'T EDIT THIS LINE
graph->edges[3].source = 3; // DON'T EDIT THIS LINE
graph->edges[3].destination = 5; // DON'T EDIT THIS LINE
graph->edges[3].weight = 2; // DON'T EDIT THIS LINE
graph->edges[4].source = 3; // DON'T EDIT THIS LINE
graph->edges[4].destination = 4; // DON'T EDIT THIS LINE
graph->edges[4].weight = 7; // DON'T EDIT THIS LINE
graph->edges[5].source = 4; // DON'T EDIT THIS LINE
graph->edges[5].destination = 5; // DON'T EDIT THIS LINE
graph->edges[5].weight = 3; // DON'T EDIT THIS LINE
graph->edges[6].source = 4; // DON'T EDIT THIS LINE
graph->edges[6].destination = 6; // DON'T EDIT THIS LINE
graph->edges[6].weight = 8; // DON'T EDIT THIS LINE
graph->edges[7].source = 5; // DON'T EDIT THIS LINE
graph->edges[7].destination = 6; // DON'T EDIT THIS LINE
graph->edges[7].weight = 9; // DON'T EDIT THIS LINE
graph->edges[8].source = 6; // DON'T EDIT THIS LINE
graph->edges[8].destination = 7; // DON'T EDIT THIS LINE
graph->edges[8].weight = 11; // DON'T EDIT THIS LINE
graph->edges[9].source = 6; // DON'T EDIT THIS LINE
graph->edges[9].destination = 8; // DON'T EDIT THIS LINE
graph->edges[9].weight = 1; // DON'T EDIT THIS LINE
graph->edges[10].source = 7; // DON'T EDIT THIS LINE
graph->edges[10].destination = 8; // DON'T EDIT THIS LINE
graph->edges[10].weight = 13; // DON'T EDIT THIS LINE
graph->edges[11].source = 7; // DON'T EDIT THIS LINE
graph->edges[11].destination = 10; // DON'T EDIT THIS LINE
graph->edges[11].weight = 6; // DON'T EDIT THIS LINE
graph->edges[12].source = 8; // DON'T EDIT THIS LINE
graph->edges[12].destination = 9; // DON'T EDIT THIS LINE
graph->edges[12].weight = 5; // DON'T EDIT THIS LINE
graph->edges[13].source = 9; // DON'T EDIT THIS LINE
graph->edges[13].destination = 10; // DON'T EDIT THIS LINE
graph->edges[13].weight = 14; // DON'T EDIT THIS LINE
}
int is_cycle() {
return 0;
}
void Kruskals_MST(GRAPH *graph){


Step by step
Solved in 4 steps

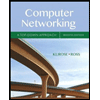
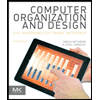
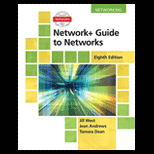
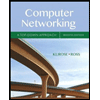
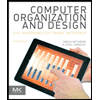
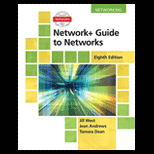
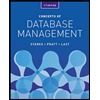
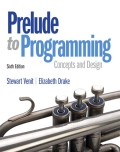
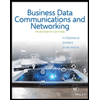