HELP IN JAVA CODE PLEASE Create code using DrawingPanel to generate values for a magic square based on a random magicValue and execute as an example is shown below Make sure the old magic square is erased when the new magic square is executed.
HELP IN JAVA CODE PLEASE Create code using DrawingPanel to generate values for a magic square based on a random magicValue and execute as an example is shown below Make sure the old magic square is erased when the new magic square is executed.
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 2GZ
Related questions
Question
HELP IN JAVA CODE PLEASE
Create code using DrawingPanel to generate values for a magic square based on a random magicValue and execute as an example is shown below
Make sure the old magic square is erased when the new magic square is executed.
![### CSC 142 Magic Square
The following is an illustration of a 4x4 magic square, where the sum of the numbers in each row, each column, and both main diagonals are the same.
#### Magic Square Grid:
```
| 8 | 11 | 14 | 1 |
| 13 | 2 | 7 | 12 |
| 3 | 16 | 9 | 6 |
| 10 | 5 | 4 | 15 |
```
To represent the above grid in a program, you can store the values using a 2-dimensional array as shown below:
```java
int [][] squareData = {
{8, 11, 14, 1},
{13, 2, 7, 12},
{3, 16, 9, 6},
{10, 5, 4, 15}
};
```
### Testing the Magic Square
To ensure that a square is a magic square, various tests can be conducted. Below are the types of tests you can perform:
1. **Row Sum Test**: Verify that the sum of each row equals the magic constant.
2. **Column Sum Test**: Verify that the sum of each column equals the magic constant.
3. **Main Diagonal Sum Test**: Verify that the sum of the main diagonal (from top-left to bottom-right) equals the magic constant.
4. **Secondary Diagonal Sum Test**: Verify that the sum of the secondary diagonal (from top-right to bottom-left) equals the magic constant.
Using the values of the example magic square provided, you can create test cases with invalid data to ensure your testing is robust. When creating these test sets, make sure to align each with the specific type of case you aim to test, whether it is row-based, column-based, or diagonal-based.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa49e8544-22cd-49e6-b20c-ed062c173676%2F478393c0-c8dd-4f1c-a137-4fcb1f2ebc28%2Fehmnlnm_processed.png&w=3840&q=75)
Transcribed Image Text:### CSC 142 Magic Square
The following is an illustration of a 4x4 magic square, where the sum of the numbers in each row, each column, and both main diagonals are the same.
#### Magic Square Grid:
```
| 8 | 11 | 14 | 1 |
| 13 | 2 | 7 | 12 |
| 3 | 16 | 9 | 6 |
| 10 | 5 | 4 | 15 |
```
To represent the above grid in a program, you can store the values using a 2-dimensional array as shown below:
```java
int [][] squareData = {
{8, 11, 14, 1},
{13, 2, 7, 12},
{3, 16, 9, 6},
{10, 5, 4, 15}
};
```
### Testing the Magic Square
To ensure that a square is a magic square, various tests can be conducted. Below are the types of tests you can perform:
1. **Row Sum Test**: Verify that the sum of each row equals the magic constant.
2. **Column Sum Test**: Verify that the sum of each column equals the magic constant.
3. **Main Diagonal Sum Test**: Verify that the sum of the main diagonal (from top-left to bottom-right) equals the magic constant.
4. **Secondary Diagonal Sum Test**: Verify that the sum of the secondary diagonal (from top-right to bottom-left) equals the magic constant.
Using the values of the example magic square provided, you can create test cases with invalid data to ensure your testing is robust. When creating these test sets, make sure to align each with the specific type of case you aim to test, whether it is row-based, column-based, or diagonal-based.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
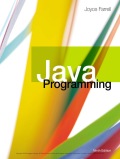
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
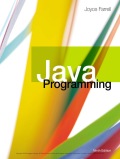
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage