em.out.println("\nTest1 Score:" + Score1.get(i) + "\nTest2 Score:" + Score2.get(i) + "\nTest3 Score:" + Score3.get(i)); // calculate average average = (Score1.get(i) + Score2.get(i) + Score3.get(i)) / 3.0; // display average System.out.printf("Average:%.2f", average); System.out.println();
// JAVA program to calculate the average score
// import utility class for list collection
import java.util.*;
// class definition
public class Main
{
// main method
public static void main(String[] args) {
// Variable declaration
char middle_initial;
int id;
double score1, score2, score3, average;
String first_name, last_name, choice;
// Scanner to read user inputs
Scanner sc = new Scanner(System.in);
// integer counter to count number of students
int counter = 0;
// run a do-while loop until the user enters N or No
do
{
// prompt user to enter first name, middle initial, and last name
System.out.print("Enter student's First Name:");
first_name = sc.next();
System.out.print("Enter student's Middle Initial:");
middle_initial = sc.next().charAt(0);
System.out.print("Enter student's Last Name:");
last_name = sc.next();
// Add fullName of student in the Name list
Name.add(first_name + "." + middle_initial + "." + last_name);
// enter id
System.out.print("Enter student's Id:");
id = sc.nextInt();
// add Id of student in Id list.
Id.add(id);
// input validation for score 1
do
{
// enter score 1
System.out.print("Enter Test1 Score:");
score1 = sc.nextDouble();
} while (score1 <= 0 || score1 > 100);
// Add valid score1 of student in Score1 list
Score1.add(score1);
// input validation for score 2
do
{
// enter score 2
System.out.print("Enter Test2 Score:");
score2 = sc.nextDouble();
} while (score2 <= 0 || score2 > 100);
// Add valid score2 of student in Score2 list
Score2.add(score2);
// input validation for score 3
do
{
// enter score 3
System.out.print("Enter Test3 Score:");
score3 = sc.nextDouble();
} while (score3 <= 0 || score3 >= 100);
// Add valid score3 of student in Score3 list
Score3.add(score3);
// increases counter by 1
counter++;
// prompt user for choice
System.out.print("\nWant to add more students?(Y/N):");
choice = sc.next();
System.out.println();
} while (choice.equalsIgnoreCase("Y") || choice.equalsIgnoreCase("Yes"));
// once loop terminates, display information of all added students
for (int i = 0; i < counter; i++)
{
// display full name, id, and test scores
System.out.println("\nStudent Name:" + Name.get(i));
System.out.println("Id:" + Id.get(i));
System.out.println("\nTest1 Score:" + Score1.get(i) +
"\nTest2 Score:" + Score2.get(i) +
"\nTest3 Score:" + Score3.get(i));
// calculate average
average = (Score1.get(i) + Score2.get(i) + Score3.get(i)) / 3.0;
// display average
System.out.printf("Average:%.2f", average);
System.out.println();
}
}
}
Hi guys can someone debug for me this program, I need the arrays and the method removed out of the program completely

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

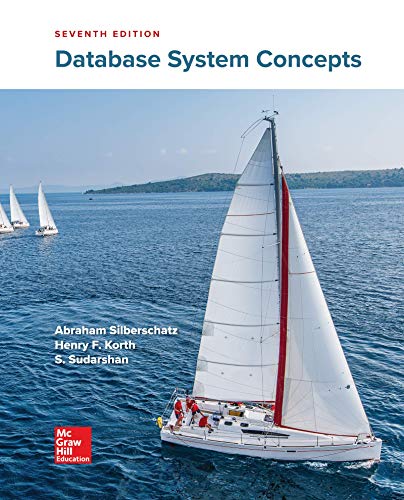
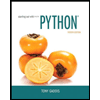
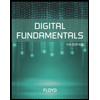
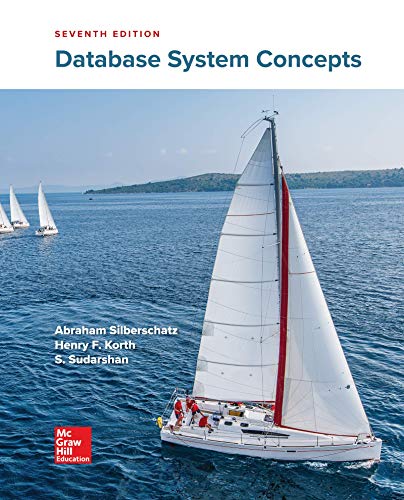
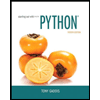
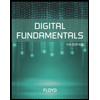
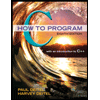
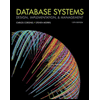
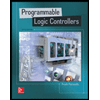