The program should have a main method and another method called passwordChecker. The main method will send the two new passwords to passwordChecker.
//Assignment 06
*/
public static void main[](String[] args) {
String pass= "ICS 111";
System.out.printIn(valPassword(pass));
}
/*
public static boolean valPassword(String password)
{
if(password.length() > 6)
{
if(checkPass(password)
{
return true;
}
else
{
return false;
}
}
else
System.out.print("Too small");
return false;
}
public static boolean checkPass (String password)
{
boolean hasNum=false; boolean hasCap = false; boolean hasLow = false; char c;
for(int i = 0; i < password.length(); i++)
{
c = password.charAt(1);
if(Character.isDigit(c));
{
hasNum = true;
}
else if(Character.isUpperCase(c))
{
hasCap = true;
}
else if(Character.isLowerCase(c))
{
hasLow = true;
}
} return true;
{
return false;
}
}



This is very simple.
Here is the complete and correct Java code for the given problem.
Note: The errors are indentation and syntax errors.
--------------------------------------------- Java Code -------------------------------------------------
public class printPassword{
public static void main(String args[]){
String pass= "ICS 111";
System.out.println(valPassword(pass));
}
}
public class valPassword{
public static boolean valPassword(String password){
if(password.length() > 6){
if(checkPass(password)){
return true;
}
else
{
return false;
}
}
else{
System.out.println("Too small");
return false;
}
}
public static boolean checkPass (String password){
boolean hasNum=false; boolean hasCap = false;
boolean hasLow = false; char c;
for(int i = 0; i < password.length(); i++){
c = password.charAt(1);
if(Character.isDigit(c)){
hasNum = true;
}
else if(Character.isUpperCase(c)){
hasCap = true;
}
else if(Character.isLowerCase(c)){
hasLow = true;
}
} return true;
return false;
}
Step by step
Solved in 2 steps

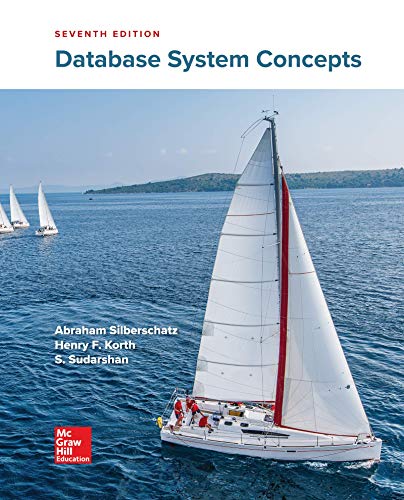
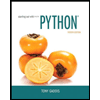
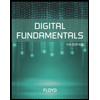
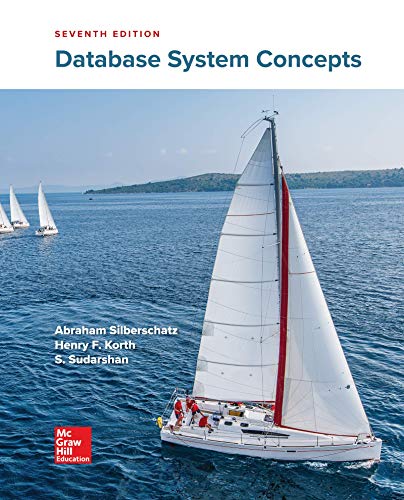
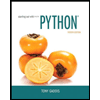
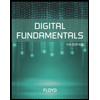
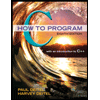
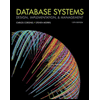
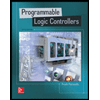