A half-life is the amount of time it takes for a substance or entity to fall to half its original value. Caffeine has a half-life of about 6 hours in humans. Given caffeine amount (in mg) as input, output the caffeine level after 6, 12, and 24 hours. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: System.out.printf("After 6 hours: %.2f mg\n", yourValue);
A half-life is the amount of time it takes for a substance or entity to fall to half its original value. Caffeine has a half-life of about 6 hours in humans. Given caffeine amount (in mg) as input, output the caffeine level after 6, 12, and 24 hours. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: System.out.printf("After 6 hours: %.2f mg\n", yourValue);
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
Java output caffeine levels
![### Understanding Caffeine Half-Life: Educational Exercise
**Concept Explanation:**
A half-life is the amount of time it takes for a substance or entity to fall to half its original value. In the context of this exercise, we are examining the half-life of caffeine in humans. Caffeine has a half-life of about 6 hours in humans.
**Exercise Objective:**
Given an initial caffeine amount (measured in milligrams), the goal is to calculate and output the caffeine level at specific intervals: after 6, 12, and 24 hours.
**Example Calculation:**
If the initial input of caffeine is 100 mg, the calculation steps are as follows:
- **After 6 hours:** The caffeine level is halved.
```java
100 / 2 = 50.00 mg
```
- **After 12 hours:** The caffeine level is halved again.
```java
50 / 2 = 25.00 mg
```
- **After 24 hours:** The caffeine level is halved once more.
```java
25 / 2 = 6.25 mg
```
**Formatted Output:**
Each floating-point value should be output with two decimal places. This can be achieved using the following command in Java:
```java
System.out.printf("After 6 hours: %.2f mg\n", yourValue);
```
**Input and Output Example:**
If the input is:
```
100
```
The output will be:
```
After 6 hours: 50.00 mg
After 12 hours: 25.00 mg
After 24 hours: 6.25 mg
```
**Additional Information:**
- A cup of coffee contains approximately 100 mg of caffeine.
- A soda typically contains around 40 mg of caffeine.
- An energy drink (though a misnomer) can have between 100 mg and 200 mg of caffeine.
**Lab Activity:**
This is part of lab activity 2.19.1 - LAB: Caffeine levels.
**Java Sample Code:**
Here's a simple Java program that performs the described calculations:
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
double caffeineAmount;
caffeineAmount = scnr.nextDouble();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc8f23c41-b0a2-4b7f-b455-de9ffcd4a71a%2F08fca281-0704-46ce-8f1c-370cdb81bcf9%2F0ql1k6m_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Understanding Caffeine Half-Life: Educational Exercise
**Concept Explanation:**
A half-life is the amount of time it takes for a substance or entity to fall to half its original value. In the context of this exercise, we are examining the half-life of caffeine in humans. Caffeine has a half-life of about 6 hours in humans.
**Exercise Objective:**
Given an initial caffeine amount (measured in milligrams), the goal is to calculate and output the caffeine level at specific intervals: after 6, 12, and 24 hours.
**Example Calculation:**
If the initial input of caffeine is 100 mg, the calculation steps are as follows:
- **After 6 hours:** The caffeine level is halved.
```java
100 / 2 = 50.00 mg
```
- **After 12 hours:** The caffeine level is halved again.
```java
50 / 2 = 25.00 mg
```
- **After 24 hours:** The caffeine level is halved once more.
```java
25 / 2 = 6.25 mg
```
**Formatted Output:**
Each floating-point value should be output with two decimal places. This can be achieved using the following command in Java:
```java
System.out.printf("After 6 hours: %.2f mg\n", yourValue);
```
**Input and Output Example:**
If the input is:
```
100
```
The output will be:
```
After 6 hours: 50.00 mg
After 12 hours: 25.00 mg
After 24 hours: 6.25 mg
```
**Additional Information:**
- A cup of coffee contains approximately 100 mg of caffeine.
- A soda typically contains around 40 mg of caffeine.
- An energy drink (though a misnomer) can have between 100 mg and 200 mg of caffeine.
**Lab Activity:**
This is part of lab activity 2.19.1 - LAB: Caffeine levels.
**Java Sample Code:**
Here's a simple Java program that performs the described calculations:
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
double caffeineAmount;
caffeineAmount = scnr.nextDouble();
![### Introduction to Basic Java Input Handling
Below is an example of a simple Java program designed to accept user input and handle it appropriately using the `Scanner` class. Follow the instructions and comments in the code to understand how it works.
#### Code Example: LabProgram.java
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
// Declare a variable to store the caffeine measurement
double caffeineMg; // "double" supports floating-point numbers like 75.5, versus int for integers like 75.
// Read a double from the user
caffeineMg = scnr.nextDouble();
/* Type your code here. */
}
}
```
#### Understanding the Code:
1. **Importing the Scanner class**:
```java
import java.util.Scanner;
```
- This line imports the `Scanner` class, which is used to get user input.
2. **Main Class Definition**:
```java
public class LabProgram {
```
- This defines a public class named `LabProgram`.
3. **Main Method**:
```java
public static void main(String[] args) {
```
- The `main` method is the entry point of the program.
4. **Creating a Scanner object**:
```java
Scanner scnr = new Scanner(System.in);
```
- This line creates a new `Scanner` object named `scnr` to read input from the standard input stream (keyboard).
5. **Variable Declaration**:
```java
double caffeineMg; // "double" supports floating-point numbers like 75.5, versus int for integers like 75.
```
- Declares a double variable `caffeineMg` to store the caffeine amount in milligrams.
6. **Reading User Input**:
```java
caffeineMg = scnr.nextDouble();
```
- Reads a floating-point number from the user and assigns it to the variable `caffeineMg`.
7. **Placeholder for Additional Code**:
```java
/* Type your code here. */
```
- This comment indicates where additional code can be added to process or use the input gathered.
#### Additional Features:
This interface shows a development environment where students can write, test, and submit their code:
- **](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc8f23c41-b0a2-4b7f-b455-de9ffcd4a71a%2F08fca281-0704-46ce-8f1c-370cdb81bcf9%2Fenpu5nn_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Introduction to Basic Java Input Handling
Below is an example of a simple Java program designed to accept user input and handle it appropriately using the `Scanner` class. Follow the instructions and comments in the code to understand how it works.
#### Code Example: LabProgram.java
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
// Declare a variable to store the caffeine measurement
double caffeineMg; // "double" supports floating-point numbers like 75.5, versus int for integers like 75.
// Read a double from the user
caffeineMg = scnr.nextDouble();
/* Type your code here. */
}
}
```
#### Understanding the Code:
1. **Importing the Scanner class**:
```java
import java.util.Scanner;
```
- This line imports the `Scanner` class, which is used to get user input.
2. **Main Class Definition**:
```java
public class LabProgram {
```
- This defines a public class named `LabProgram`.
3. **Main Method**:
```java
public static void main(String[] args) {
```
- The `main` method is the entry point of the program.
4. **Creating a Scanner object**:
```java
Scanner scnr = new Scanner(System.in);
```
- This line creates a new `Scanner` object named `scnr` to read input from the standard input stream (keyboard).
5. **Variable Declaration**:
```java
double caffeineMg; // "double" supports floating-point numbers like 75.5, versus int for integers like 75.
```
- Declares a double variable `caffeineMg` to store the caffeine amount in milligrams.
6. **Reading User Input**:
```java
caffeineMg = scnr.nextDouble();
```
- Reads a floating-point number from the user and assigns it to the variable `caffeineMg`.
7. **Placeholder for Additional Code**:
```java
/* Type your code here. */
```
- This comment indicates where additional code can be added to process or use the input gathered.
#### Additional Features:
This interface shows a development environment where students can write, test, and submit their code:
- **
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
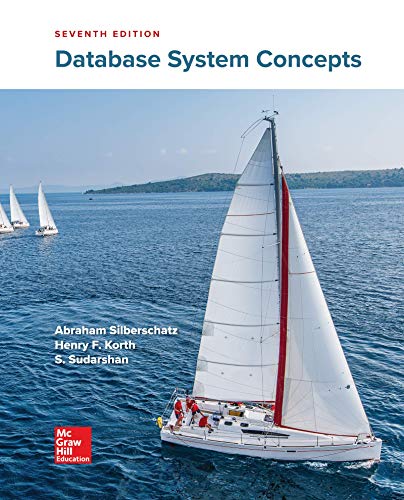
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
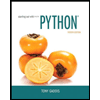
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
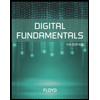
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
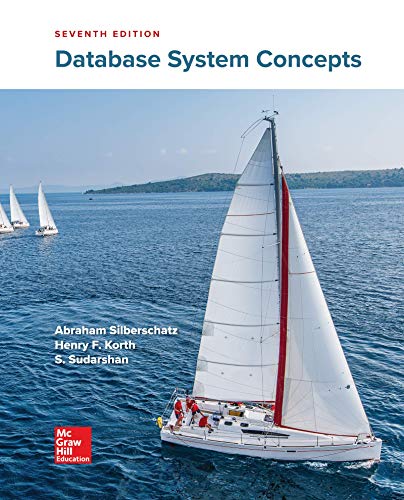
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
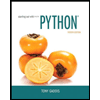
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
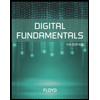
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
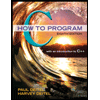
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
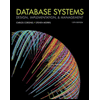
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
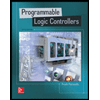
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education