Exercise 1 - What is wrong in the code below: public class Main { public static void main(String[] args) { int x = 5 int y = 10; int z = x + y; System.out.println(z); } } Exercise 2 - What is wrong in the code below: public class Main { public static void main(String[] args) { int x = 5; int y = 0; int z = x / y; System.out.println(z); } } Exercise 3 - What is wrong in the code below: public class Main { public static void main(String[] args) { int x = 5; int y = 10; int z = x - y; System.out.println("The difference between " + x + " and " + y + " is " + z); } } Exercise 4 - What is wrong in the code below: public class Main { public static void main(String[] args) { int 1stNumber = 5; int second_number = 10; int sum = 1stNumber + second_number; System.out.println(sum); } } Exercise 5 - What is wrong in the code below: public class Main { public static void main(String[] args) { int x = 5; double y = 10.5; int sum = x + y; System.out.println(sum); } }
Exercise 1 - What is wrong in the code below:
public class Main {
public static void main(String[] args) {
int x = 5
int y = 10;
int z = x + y;
System.out.println(z);
}
}
Exercise 2 - What is wrong in the code below:
public class Main {
public static void main(String[] args) {
int x = 5;
int y = 0;
int z = x / y;
System.out.println(z);
}
}
Exercise 3 - What is wrong in the code below:
public class Main {
public static void main(String[] args) {
int x = 5;
int y = 10;
int z = x - y;
System.out.println("The difference between " + x + " and " + y + " is " + z);
}
}
Exercise 4 - What is wrong in the code below:
public class Main {
public static void main(String[] args) {
int 1stNumber = 5;
int second_number = 10;
int sum = 1stNumber + second_number;
System.out.println(sum);
}
}
Exercise 5 - What is wrong in the code below:
public class Main {
public static void main(String[] args) {
int x = 5;
double y = 10.5;
int sum = x + y;
System.out.println(sum);
}
}

Step by step
Solved in 5 steps with 1 images

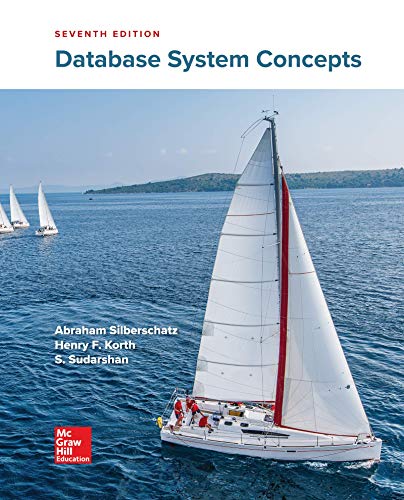
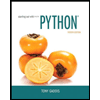
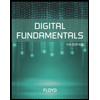
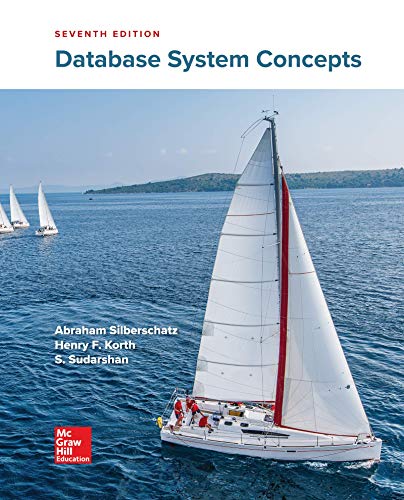
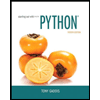
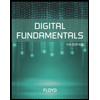
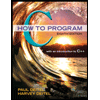
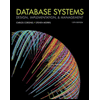
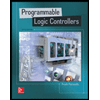