Define a new "Exam" class that manages the exam name (string) and its score (integer). For example, an exam can have - "Midterm Exam", 100 - "Final Exam", 50 The class must not provide the default constructor. It must require the exam name and score in order to initialize the Exam object. The class must provide only the following methods (no more and no less): - isPerfect method that returns true if the score is exactly 100 and false otherwise. - isPassing method that returns true if the score is equal or greater than 70 and false otherwise. - toString method must return all the exam information including the result of the exam as a string in the following format: EXAM() SCORE() RESULT(Pass/Fail) such as EXAM(Midterm Exam) SCORE(100) RESULT(Pass) EXAM(Final Exam) SCORE(50) RESULT(Fail) "Pass" means the score is greater or equal 70. "Fail" is whenever the score is below 70. - isGreater method that compares with another Exam object and return true if the score of the current Exam object is greater than the score of the other Exam object. - increment method that accepts the increment score amount (int) and increase the current score of that exam by that given amount Important notes: - Please do not provide any other method including a method to return the score. - The class must not provide the default constructor. Show how this Exam class being used to create objects and how these methods are being called and return proper values.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Define a new "Exam" class that manages the exam name (string) and
its score (integer). For example, an exam can have
- "Midterm Exam", 100
- "Final Exam", 50
The class must not provide the default constructor. It must require the
exam name and score in order to initialize the Exam object.
The class must provide only the following methods (no more and no
less):
- isPerfect method that returns true if the score is exactly 100
and false otherwise.
- isPassing method that returns true if the score is equal or
greater than 70 and false otherwise.
- toString method must return all the exam information including
the result of the exam as a string in the following format:
EXAM(<name>) SCORE(<score>) RESULT(Pass/Fail)
such as
EXAM(Midterm Exam) SCORE(100) RESULT(Pass)
EXAM(Final Exam) SCORE(50) RESULT(Fail)
"Pass" means the score is greater or equal 70.
"Fail" is whenever the score is below 70.
- isGreater method that compares with another Exam object and
return true if the score of the current Exam object is greater than
the score of the other Exam object.
- increment method that accepts the increment score amount
(int) and increase the current score of that exam by that given
amount
Important notes:
- Please do not provide any other method including a method
to return the score.
- The class must not provide the default constructor.
Show how this Exam class being used to create objects and how these
methods are being called and return proper values.

Step by step
Solved in 4 steps with 2 images

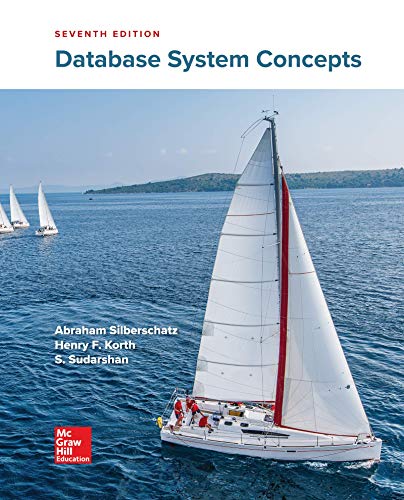
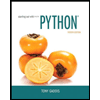
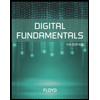
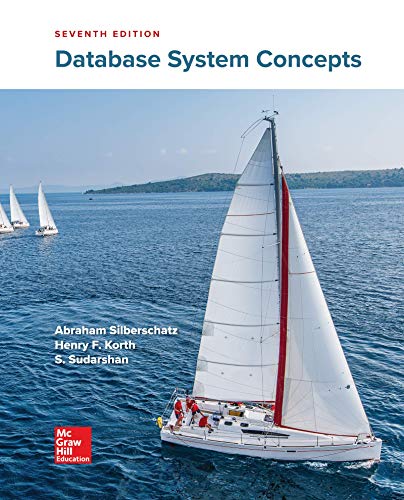
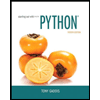
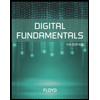
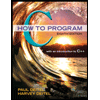
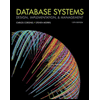
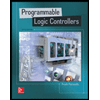