d a void instance method replaceAll(E oldE, E newE) in the MyLinkedList class to replace the occurrence of all oldE value with the specified newE value. Implement this method in O(n) time. import java.util.*; public class PExamCh24 { public static void main(String[] args) { new PExamCh24(); } public PExamCh24() { // Create a list for strings MyLinkedList list = new MyLinkedList<>(); // Add elements to the list list.add("America"); // Add America to the list System.out.println("(1) " + list); list.add(0, "Japan"); // Add Canada to the beginning of the list System.out.println("(2) " + list); list.add("Russia"); // Add Russia to the end of the list System.out.println("(3) " + list); list.addLast("Japan"); // Add France to the end of the list System.out.println("(4) " + list); list.add(2, "Japan"); // Add Germany to the list at index 2 System.out.println("(5) " + list); list.add(5, "Norway"); // Add Norway to the list at index 5 System.out.println("(6) " + list); list.add(0, "Japan"); // Same as list.addFirst("Poland") System.out.println("(7) " + list); // Remove elements from the list list.remove(0); // Same as list.remove("Australia") in this case System.out.println("(8) " + list); list.remove(2); // Remove the element at index 2 System.out.println("(9) " + list); list.remove(list.size() - 1); // Remove the last element System.out.print("(10) " + list + "\n(11) "); list.replaceAll("Japan", "England"); for (String s : list) { System.out.print(s.toUpperCase() + " "); } } class MyLinkedList implements MyList { public void replaceAll(E oldE, E newE) { // WRITE YOUR CODE HERE } protected Node head, tail; protected int size = 0; // Number of elements in the list /** * Create an empty list */ public MyLinkedList() { } /** * Create a list from an array of objects */ public MyLinkedList(E[] objects) { for (int i = 0; i < objects.length; i++) { add(objects[i]); } } /** * Return the head element in the list */ public E getFirst() { if (size == 0) { return null; } else { return head.element; } } /** * Return the last element in the list */ public E getLast() { if (size == 0) { return null; } else { return tail.element; } }
Add a void instance method replaceAll(E oldE, E newE) in the MyLinkedList class to replace the occurrence of all oldE value with the specified newE value. Implement this method in O(n) time.
import java.util.*;
public class PExamCh24 {
public static void main(String[] args) {
new PExamCh24();
}
public PExamCh24() {
// Create a list for strings
MyLinkedList<String> list = new MyLinkedList<>();
// Add elements to the list
list.add("America"); // Add America to the list
System.out.println("(1) " + list);
list.add(0, "Japan"); // Add Canada to the beginning of the list
System.out.println("(2) " + list);
list.add("Russia"); // Add Russia to the end of the list
System.out.println("(3) " + list);
list.addLast("Japan"); // Add France to the end of the list
System.out.println("(4) " + list);
list.add(2, "Japan"); // Add Germany to the list at index 2
System.out.println("(5) " + list);
list.add(5, "Norway"); // Add Norway to the list at index 5
System.out.println("(6) " + list);
list.add(0, "Japan"); // Same as list.addFirst("Poland")
System.out.println("(7) " + list);
// Remove elements from the list
list.remove(0); // Same as list.remove("Australia") in this case
System.out.println("(8) " + list);
list.remove(2); // Remove the element at index 2
System.out.println("(9) " + list);
list.remove(list.size() - 1); // Remove the last element
System.out.print("(10) " + list + "\n(11) ");
list.replaceAll("Japan", "England");
for (String s : list) {
System.out.print(s.toUpperCase() + " ");
}
}
class MyLinkedList<E> implements MyList<E> {
public void replaceAll(E oldE, E newE) {
// WRITE YOUR CODE HERE
}
protected Node<E> head, tail;
protected int size = 0; // Number of elements in the list
/**
* Create an empty list
*/
public MyLinkedList() {
}
/**
* Create a list from an array of objects
*/
public MyLinkedList(E[] objects) {
for (int i = 0; i < objects.length; i++) {
add(objects[i]);
}
}
/**
* Return the head element in the list
*/
public E getFirst() {
if (size == 0) {
return null;
} else {
return head.element;
}
}
/**
* Return the last element in the list
*/
public E getLast() {
if (size == 0) {
return null;
} else {
return tail.element;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

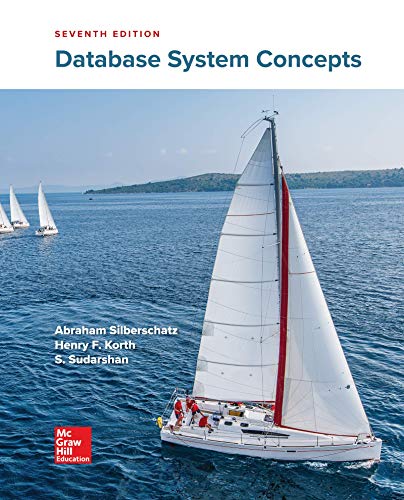
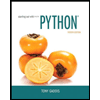
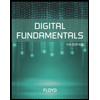
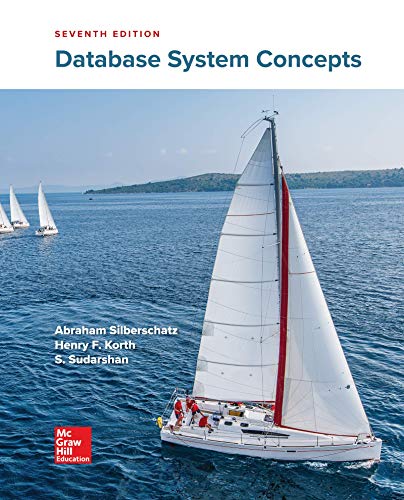
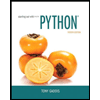
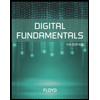
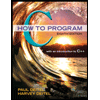
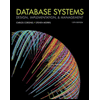
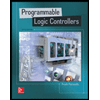