public class PhoneList { public static void main (String[] args) { Contact[] friends = new Contact [8]; friends [0] = new Contact ("John", "Smith", "610-555-7384"); friends [1] = new Contact ("Sarah", "Barnes", "215-555-3827"); friends [2] = new Contact ("Mark", "Riley", "733-555-2969"); friends [3] = new Contact ("Laura", "Getz", "663-555-3984"); friends [4] = new Contact ("Larry", "Smith", "464-555-3489"); friends [5] = new Contact ("Frank", "Phelps" "322-555-2284"); friends [6] = new Contact ("Mario", "Guzman", "804-555-9066"); friends [7] = new Contact ("Marsha", "Grant", "243-555-2837"); Sorting.selectionSort (friends); for (Contact friend friends) System.out.println (friend); I
public class PhoneList { public static void main (String[] args) { Contact[] friends = new Contact [8]; friends [0] = new Contact ("John", "Smith", "610-555-7384"); friends [1] = new Contact ("Sarah", "Barnes", "215-555-3827"); friends [2] = new Contact ("Mark", "Riley", "733-555-2969"); friends [3] = new Contact ("Laura", "Getz", "663-555-3984"); friends [4] = new Contact ("Larry", "Smith", "464-555-3489"); friends [5] = new Contact ("Frank", "Phelps" "322-555-2284"); friends [6] = new Contact ("Mario", "Guzman", "804-555-9066"); friends [7] = new Contact ("Marsha", "Grant", "243-555-2837"); Sorting.selectionSort (friends); for (Contact friend friends) System.out.println (friend); I
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Modify the Contact class in Chapter 10 so that the sorting methods invoked in PhoneList.java keeps the Contact objects sorted by phone number.
![```java
public class PhoneList {
public static void main (String[] args) {
Contact[] friends = new Contact[8];
friends[0] = new Contact("John", "Smith", "610-555-7384");
friends[1] = new Contact("Sarah", "Barnes", "215-555-3827");
friends[2] = new Contact("Mark", "Riley", "733-555-2969");
friends[3] = new Contact("Laura", "Getz", "663-555-3984");
friends[4] = new Contact("Larry", "Smith", "464-555-3489");
friends[5] = new Contact("Frank", "Phelps", "322-555-2284");
friends[6] = new Contact("Mario", "Guzman", "804-555-9066");
friends[7] = new Contact("Marsha", "Grant", "243-555-2837");
Sorting.selectionSort(friends);
for (Contact friend : friends)
System.out.println(friend);
}
}
```
**Explanation of Code:**
This Java program defines a `PhoneList` class, which contains a `main` method to execute the program. The program creates an array of `Contact` objects called `friends`, with a length of 8. Each element of this array is a new `Contact` object initialized with a first name, last name, and phone number.
After populating the `friends` array, the program calls a method `Sorting.selectionSort(friends)`, which presumably sorts the array using selection sort (the implementation of this method is not provided in the snippet).
Finally, the program iterates over each `Contact` object in the `friends` array and prints it to the system output. This likely involves calling a `toString` method on each `Contact` object, defined elsewhere in the code.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6a7e4603-16da-4579-84a9-ea3de183c343%2F3c237c4e-85e2-4acf-ba66-5b4257669e05%2F2kzsid2_processed.png&w=3840&q=75)
Transcribed Image Text:```java
public class PhoneList {
public static void main (String[] args) {
Contact[] friends = new Contact[8];
friends[0] = new Contact("John", "Smith", "610-555-7384");
friends[1] = new Contact("Sarah", "Barnes", "215-555-3827");
friends[2] = new Contact("Mark", "Riley", "733-555-2969");
friends[3] = new Contact("Laura", "Getz", "663-555-3984");
friends[4] = new Contact("Larry", "Smith", "464-555-3489");
friends[5] = new Contact("Frank", "Phelps", "322-555-2284");
friends[6] = new Contact("Mario", "Guzman", "804-555-9066");
friends[7] = new Contact("Marsha", "Grant", "243-555-2837");
Sorting.selectionSort(friends);
for (Contact friend : friends)
System.out.println(friend);
}
}
```
**Explanation of Code:**
This Java program defines a `PhoneList` class, which contains a `main` method to execute the program. The program creates an array of `Contact` objects called `friends`, with a length of 8. Each element of this array is a new `Contact` object initialized with a first name, last name, and phone number.
After populating the `friends` array, the program calls a method `Sorting.selectionSort(friends)`, which presumably sorts the array using selection sort (the implementation of this method is not provided in the snippet).
Finally, the program iterates over each `Contact` object in the `friends` array and prints it to the system output. This likely involves calling a `toString` method on each `Contact` object, defined elsewhere in the code.
Expert Solution

Step 1
To compare two String objects, use the compareTo method on a String object For example The function compareTo compares "apple" and "cat" alphabetically and outputs the result as an integer.
- If "apple" appears before "cat" in the alphabet, the compareTo method returns a negative number as a result.
- If "apple" and "cat" are the same in alphabetical order, the compareTo function returns zero.
- If "apple" follows "cat" alphabetically, the compareTo function gives a positive number.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
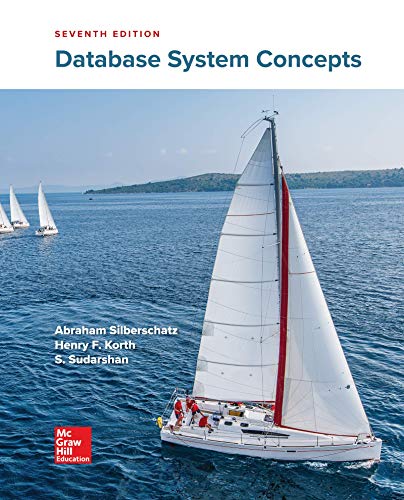
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
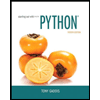
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
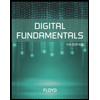
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
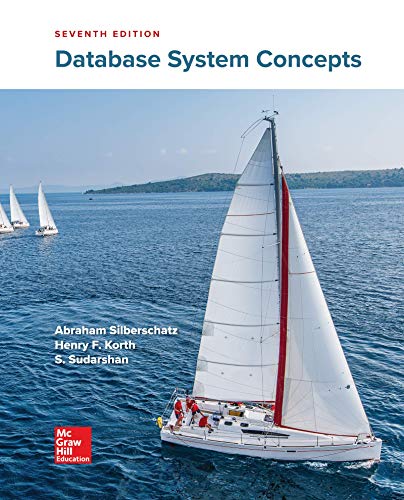
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
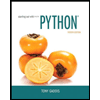
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
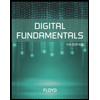
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
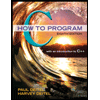
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
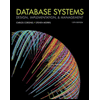
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
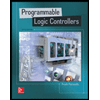
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education