Create a Java project called StockTask 1. Write an object class named Stock that contains: A private string data field named symbol for the stock's symbol. A private string data field named name for the stock's name. A private double data field named previousClosingPrice that stores the stock price for the previous day. A private double data field named currentPrice that stores the stock price for the current time. A constructor that creates a stock with the specified symbol and name. Accessor methods (get-methods) for the data fields. Mutator method (set-methods) for the data fields. A method named getChangePercent() that returns the percentage changed from previousClosingPrice to currentPrice. Formula: perc_change = (current price - previous closing price) / previous closing price x 100 2. Write a test class named testStock that creates a Stock object with the stock symbol SUNW, the name Sun Microsystems Inc., and the previous closing price of 100. Set a new current price to 90. Display the symbol and name of the company, the previous and current prices and price-change percentage. Example of output: Stock symbol: SUMW Stock name: Sun Microsystems Inc. Previous Closing Price: 100.0 Current price: 90.0 Price change: -10.0% Add code to create another Stock object with the stock symbol ORCL, the name Oracle Corporation/ Set the previous closing price to 34.5. Set a new current price to 38.75. Display the symbol and name of the company, the previous and current prices and price-change percentage. Example of output: Stock symbol: ORCL Stock name: Oracle Corporation Previous Closing Price: 34.5 Current Price: 38.75 Price Change: 12.32%
Create a Java project called StockTask
1. Write an object class named Stock that contains:
- A private string data field named symbol for the stock's symbol.
- A private string data field named name for the stock's name.
- A private double data field named previousClosingPrice that stores the stock price for the previous day.
- A private double data field named currentPrice that stores the stock price for the current time.
- A constructor that creates a stock with the specified symbol and name.
- Accessor methods (get-methods) for the data fields.
- Mutator method (set-methods) for the data fields.
- A method named getChangePercent() that returns the percentage changed from previousClosingPrice to currentPrice.
- Formula: perc_change = (current price - previous closing price) / previous closing price x 100
2. Write a test class named testStock that creates a Stock object with the stock symbol SUNW, the name Sun Microsystems Inc., and the previous closing price of 100.
Set a new current price to 90. Display the symbol and name of the company, the previous and current prices and price-change percentage.
Example of output:
Stock symbol: SUMW
Stock name: Sun Microsystems Inc.
Previous Closing Price: 100.0
Current price: 90.0
Price change: -10.0%
Add code to create another Stock object with the stock symbol ORCL, the name Oracle Corporation/
- Set the previous closing price to 34.5.
- Set a new current price to 38.75. Display the symbol and name of the company, the previous and current prices and price-change percentage.
- Example of output:
Stock symbol: ORCL
Stock name: Oracle Corporation
Previous Closing Price: 34.5
Current Price: 38.75
Price Change: 12.32%

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 8 images

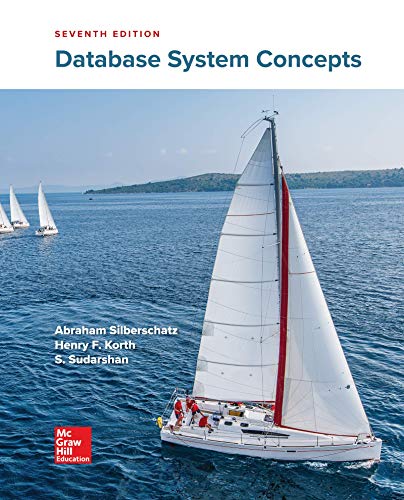
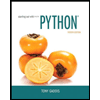
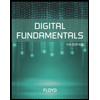
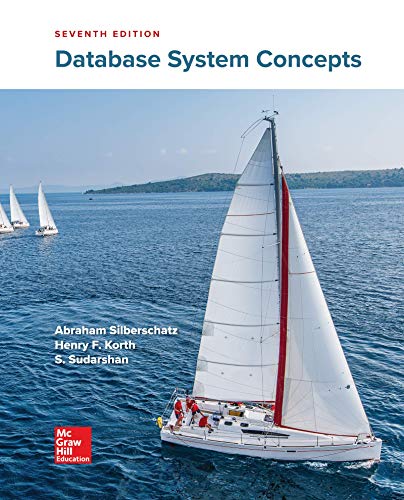
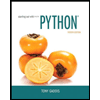
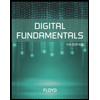
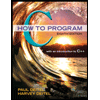
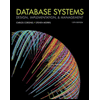
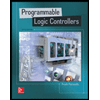