in c # i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book. The Corner’s Turn() method should display You turn corners to go around the block. The Pancake's Turn() method should display You turn a pancake when it's done on one side. The Leaf's Turn() method should display A leaf turns colors in the fall. i keep getting errors Unit TestIncomplete Page class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTest3c897a9a.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest3c897a9a.cs(23,7): error CS0246: The type or namespace name `Page' could not be found. Are you missing `TurningDemo' using directive? NtTest3c897a9a.cs(24,7): error CS0841: A local variable `page' cannot be used before it is declared Unit TestIncomplete Leaf class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTestedfb3216.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTestedfb3216.cs(23,7): error CS0246: The type or namespace name `Leaf' could not be found. Are you missing `TurningDemo' using directive? NtTestedfb3216.cs(24,7): error CS0841: A local variable `leaf' cannot be used before it is declared Unit TestIncomplete Pancake class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTestd679fa4e.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTestd679fa4e.cs(23,7): error CS0246: The type or namespace name `Pancake' could not be found. Are you missing `TurningDemo' using directive? NtTestd679fa4e.cs(24,7): error CS0841: A local variable `pancake' cannot be used before it is declared Unit TestIncomplete Corner class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTest0922cef6.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest0922cef6.cs(23,7): error CS0246: The type or namespace name `Corner' could not be found. Are you missing `TurningDemo' using directive? NtTest0922cef6.cs(24,7): error CS0841: A local variable `corner' cannot be used before it is declared my code is using System; namespace TurningDemo { public interface ITurnable { void Turn(); } public class Page : ITurnable { public void Turn() { Console.WriteLine("You turn a page in a book."); } } public class Leaf : ITurnable { public void Turn() { Console.WriteLine("A leaf turns colors in the fall."); } } public class Pancake : ITurnable { public void Turn() { Console.WriteLine("You turn a pancake when it's done on one side."); } } public class Corner : ITurnable { public void Turn() { Console.WriteLine("You turn corners to go around the block."); } } class Program { static void Main(string[] args) { ITurnable page = new Page(); page.Turn(); ITurnable leaf = new Leaf(); leaf.Turn(); ITurnable pancake = new Pancake(); pancake.Turn(); ITurnable corner = new Corner(); corner.Turn(); } } }
in c # i need to
Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf.
Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable.
Create each class’s Turn() method to display an appropriate message. For example:
- The Page’s Turn() method should display You turn a page in a book.
- The Corner’s Turn() method should display You turn corners to go around the block.
- The Pancake's Turn() method should display You turn a pancake when it's done on one side.
- The Leaf's Turn() method should display A leaf turns colors in the fall.
i keep getting errors
Unit TestIncomplete
Page class defined
Build Status
Build Failed
Build Output
Compilation failed: 3 error(s), 0 warnings
NtTest3c897a9a.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?
NtTest3c897a9a.cs(23,7): error CS0246: The type or namespace name `Page' could not be found. Are you missing `TurningDemo' using directive?
NtTest3c897a9a.cs(24,7): error CS0841: A local variable `page' cannot be used before it is declared
Unit TestIncomplete
Leaf class defined
Build Status
Build Failed
Build Output
Compilation failed: 3 error(s), 0 warnings
NtTestedfb3216.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?
NtTestedfb3216.cs(23,7): error CS0246: The type or namespace name `Leaf' could not be found. Are you missing `TurningDemo' using directive?
NtTestedfb3216.cs(24,7): error CS0841: A local variable `leaf' cannot be used before it is declared
Unit TestIncomplete
Pancake class defined
Build Status
Build Failed
Build Output
Compilation failed: 3 error(s), 0 warnings
NtTestd679fa4e.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?
NtTestd679fa4e.cs(23,7): error CS0246: The type or namespace name `Pancake' could not be found. Are you missing `TurningDemo' using directive?
NtTestd679fa4e.cs(24,7): error CS0841: A local variable `pancake' cannot be used before it is declared
Unit TestIncomplete
Corner class defined
Build Status
Build Failed
Build Output
Compilation failed: 3 error(s), 0 warnings
NtTest0922cef6.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?
NtTest0922cef6.cs(23,7): error CS0246: The type or namespace name `Corner' could not be found. Are you missing `TurningDemo' using directive?
NtTest0922cef6.cs(24,7): error CS0841: A local variable `corner' cannot be used before it is declared
my code is
using System;
namespace TurningDemo
{
public interface ITurnable
{
void Turn();
}
public class Page : ITurnable
{
public void Turn()
{
Console.WriteLine("You turn a page in a book.");
}
}
public class Leaf : ITurnable
{
public void Turn()
{
Console.WriteLine("A leaf turns colors in the fall.");
}
}
public class Pancake : ITurnable
{
public void Turn()
{
Console.WriteLine("You turn a pancake when it's done on one side.");
}
}
public class Corner : ITurnable
{
public void Turn()
{
Console.WriteLine("You turn corners to go around the block.");
}
}
class Program
{
static void Main(string[] args)
{
ITurnable page = new Page();
page.Turn();
ITurnable leaf = new Leaf();
leaf.Turn();
ITurnable pancake = new Pancake();
pancake.Turn();
ITurnable corner = new Corner();
corner.Turn();
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

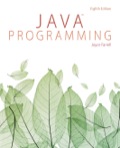
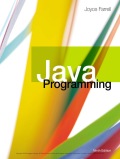
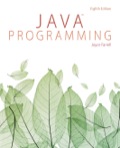
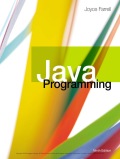
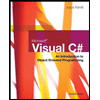
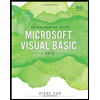