Consider the classes base and derived as follows. class base { protected: virtual void step_one() {std::cout << "base::step_one" << std::endl;} virtual void step_two() {std::cout << "base::step_two" << std::endl;} public: void run() { std::cout << "enter base::run" << std::endl; step_one(); step_two(); std::cout << "exit base::run" << std::endl; } }; class derived : public base { protected: void step_one() {std::cout << "derived::step_one" << std::endl;} void step_two() {std::cout << "derived::step_two" << std::endl;} public: void run() { std::cout << "enter derived::run" << std::endl; step_one(); step_two(); std::cout << "exit derived::run" << std::endl; } }; A. What’s the output of the following function test1? void test1() { derived d; derived *p = &d; p->run(); } B. What’s the output of the following function test2? void test2() { derived d; base *p = &d; p->run(); }
Consider the classes base and derived as follows.
class base {
protected:
virtual void step_one() {std::cout << "base::step_one" << std::endl;}
virtual void step_two() {std::cout << "base::step_two" << std::endl;}
public:
void run() {
std::cout << "enter base::run" << std::endl;
step_one();
step_two();
std::cout << "exit base::run" << std::endl;
}
};
class derived : public base {
protected:
void step_one() {std::cout << "derived::step_one" << std::endl;}
void step_two() {std::cout << "derived::step_two" << std::endl;}
public:
void run() {
std::cout << "enter derived::run" << std::endl;
step_one();
step_two();
std::cout << "exit derived::run" << std::endl;
}
};
A. What’s the output of the following function test1?
void test1() {
derived d;
derived *p = &d;
p->run();
}
B. What’s the output of the following function test2?
void test2() {
derived d;
base *p = &d;
p->run();
}

In the first question 1, first an object of class dervied is created and then a pointer of type derived is created.
This pointer is pointing to the location of the derived class object.
Using the above pointer, the function run() of the derived class is called. The Derived class's step_one() and step_two() functions are also called within the function.
Thus, the output will be:
enter derived::run
derived::step_one
derived::step_two
exit derived::run
Step by step
Solved in 2 steps

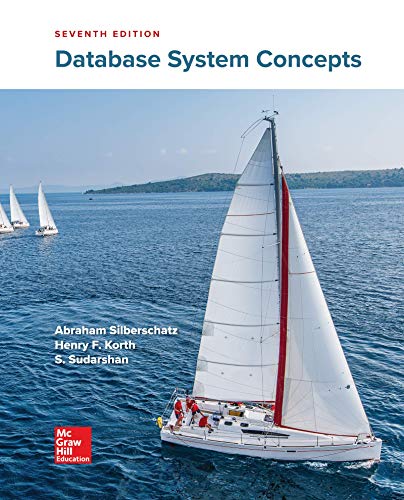
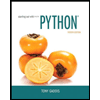
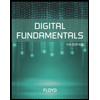
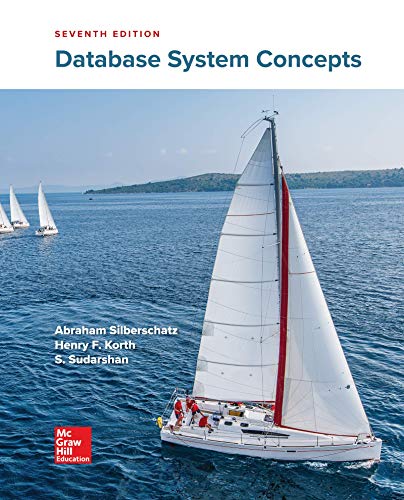
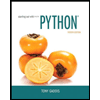
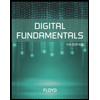
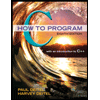
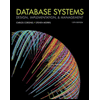
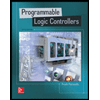