Change the given source code for Home Automation application into Command Pattern. I have listed the following source code 1. Bathroom.java public class Bathroom extends Room { private String hotWater; } 2. Bedroom.java public class Bedroom extends Room { private String bed; } 3. Client.java public class Client { public static void main(String[] args) { House house = new House(); house.addRoom(new LivingRoom()); house.addRoom(new Bedroom()); house.addRoom(new Bedroom()); house.addRoom(new Bedroom()); house.addRoom(new Kitchen()); house.addRoom(new Bathroom()); house.rooms.forEach(Room::switchLights); } } 4.FloorLamp.java public class FloorLamp { private Light light; public FloorLamp(){ this.light = new Light(); } public void switchLights(){ light.switchLights(); System.out.println(this.getClass()+"'s light is "+this.light.isSwitchedon()); } } 5.House.java import java.util.ArrayList; import java.util.List; public class House { List rooms; public House(){ rooms = new ArrayList<>(); } public void addRoom(Room room) { rooms.add(room); } } 6.Kitchen.java public class Kitchen extends Room{ private String oven; } 7.Light.java public class Light { private boolean switchedon = false; public boolean isSwitchedon() { return switchedon; } /*public void setSwitchedon(boolean switchedon) { this.switchedon = switchedon; }*/ public void switchLights() { switchedon = !switchedon; //System.out.println(this.getClass()+"'s light is "+this.switchedon); } } 8. LivingRoom.java public class LivingRoom extends Room{ private String windows; private String slidingDoors; private FloorLamp floorLamp; public LivingRoom(){ floorLamp = new FloorLamp(); floorLamp.switchLights(); } } 9. Room.java public class Room { private Light light; public Room() { this.light = new Light(); } public void switchLights() { light.switchLights(); //light.setSwitchedon(!light.isSwitchedon());//if on turns off, if off turns on System.out.println(this.getClass()+"'s light is "+this.light.isSwitchedon()); } }
Change the given source code for Home Automation application into Command Pattern. I have listed the following source code
1. Bathroom.java
public class Bathroom extends Room {
private String hotWater;
}
2. Bedroom.java
public class Bedroom extends Room {
private String bed;
}
3. Client.java
public class Client {
public static void main(String[] args) {
House house = new House();
house.addRoom(new LivingRoom());
house.addRoom(new Bedroom());
house.addRoom(new Bedroom());
house.addRoom(new Bedroom());
house.addRoom(new Kitchen());
house.addRoom(new Bathroom());
house.rooms.forEach(Room::switchLights);
}
}
4.FloorLamp.java
public class FloorLamp {
private Light light;
public FloorLamp(){
this.light = new Light();
}
public void switchLights(){
light.switchLights();
System.out.println(this.getClass()+"'s light is "+this.light.isSwitchedon());
}
}
5.House.java
import java.util.ArrayList;
import java.util.List;
public class House {
List<Room> rooms;
public House(){
rooms = new ArrayList<>();
}
public void addRoom(Room room)
{
rooms.add(room);
}
}
6.Kitchen.java
public class Kitchen extends Room{
private String oven;
}
7.Light.java
public class Light {
private boolean switchedon = false;
public boolean isSwitchedon()
{
return switchedon;
}
/*public void setSwitchedon(boolean switchedon)
{
this.switchedon = switchedon;
}*/
public void switchLights()
{
switchedon = !switchedon;
//System.out.println(this.getClass()+"'s light is "+this.switchedon);
}
}
8. LivingRoom.java
public class LivingRoom extends Room{
private String windows;
private String slidingDoors;
private FloorLamp floorLamp;
public LivingRoom(){
floorLamp = new FloorLamp();
floorLamp.switchLights();
}
}
9. Room.java
public class Room {
private Light light;
public Room() {
this.light = new Light();
}
public void switchLights()
{
light.switchLights();
//light.setSwitchedon(!light.isSwitchedon());//if on turns off, if off turns on
System.out.println(this.getClass()+"'s light is "+this.light.isSwitchedon());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

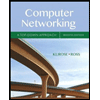
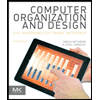
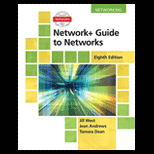
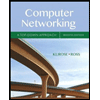
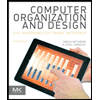
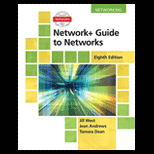
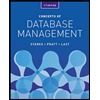
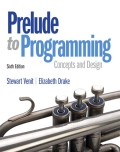
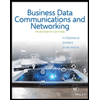