Given the main class Dog.java and the following subclass Poodle.java: Instructions for Poodle.java: Variables: Double whiskerLength – the length of the dog’s longest whisker in inches An invalid value should default this field to 8.0. A value is invalid if it is not a number, infinite, nonpositive, or null. Constructors: A constructor that takes in name, length, weight, and whiskerLength. A constructor that takes in no parameters and sets name to “Bluu”, length to 52.0, weight to 720.0, and whiskerLength to 5.0. A copy constructor that deep copies all necessary instance fields. Methods: isHairy Returns a boolean reflecting if the dog is hairy. A dog is said to be hairy if its longest whisker is longer than its end-to-end length. toString Overrides the corresponding method in its super class, such that a String with the following format is returned without trailing or leading whitespace. “I’m a talking dog named . My length is ft in and my weight is lbs oz. I’m a poodle whose longest whisker is , so I {am/am not} shaggy.” Setter for whiskerLength. If the input is invalid, set the field to its default value 1. How do I go about creating the setter to check for invalid inputs, and incorportating it into Constructor A. 2. Is there a way to call the toString in Dog.java and combining with the "I'm a Poodle whos longest whisker..." part of the toString in Poodle.java 3. Overall is there anything else that should be improved/cleaned up?
Given the main class Dog.java and the following subclass Poodle.java: Instructions for Poodle.java: Variables: Double whiskerLength – the length of the dog’s longest whisker in inches An invalid value should default this field to 8.0. A value is invalid if it is not a number, infinite, nonpositive, or null. Constructors: A constructor that takes in name, length, weight, and whiskerLength. A constructor that takes in no parameters and sets name to “Bluu”, length to 52.0, weight to 720.0, and whiskerLength to 5.0. A copy constructor that deep copies all necessary instance fields. Methods: isHairy Returns a boolean reflecting if the dog is hairy. A dog is said to be hairy if its longest whisker is longer than its end-to-end length. toString Overrides the corresponding method in its super class, such that a String with the following format is returned without trailing or leading whitespace. “I’m a talking dog named . My length is ft in and my weight is lbs oz. I’m a poodle whose longest whisker is , so I {am/am not} shaggy.” Setter for whiskerLength. If the input is invalid, set the field to its default value 1. How do I go about creating the setter to check for invalid inputs, and incorportating it into Constructor A. 2. Is there a way to call the toString in Dog.java and combining with the "I'm a Poodle whos longest whisker..." part of the toString in Poodle.java 3. Overall is there anything else that should be improved/cleaned up?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Given the main class Dog.java and the following subclass Poodle.java:
Instructions for Poodle.java:
Variables:
- Double whiskerLength – the length of the dog’s longest whisker in inches
- An invalid value should default this field to 8.0.
- A value is invalid if it is not a number, infinite, nonpositive, or null.
Constructors:
- A constructor that takes in name, length, weight, and whiskerLength.
- A constructor that takes in no parameters and sets name to “Bluu”, length to 52.0, weight to 720.0, and whiskerLength to 5.0.
- A copy constructor that deep copies all necessary instance fields.
Methods:
- isHairy
- Returns a boolean reflecting if the dog is hairy.
- A dog is said to be hairy if its longest whisker is longer than its end-to-end length.
- toString
- Overrides the corresponding method in its super class, such that a String with the following format is returned without trailing or leading whitespace.
- “I’m a talking dog named <name>. My length is <feet> ft <inches> in and my weight is <pounds> lbs <ounces> oz. I’m a poodle whose longest whisker is <whiskerLength>, so I {am/am not} shaggy.”
- Setter for whiskerLength.
If the input is invalid, set the field to its default value
1. How do I go about creating the setter to check for invalid inputs, and incorportating it into Constructor A.
2. Is there a way to call the toString in Dog.java and combining with the "I'm a Poodle whos longest whisker..." part of the toString in Poodle.java
3. Overall is there anything else that should be improved/cleaned up?

Transcribed Image Text:public class Poodle extends Dog{
private Double whiskerLength;
public Poodle(String name, double length, double weight, double whiskerLength) {
this.name = name;
}
this.length = length;
this.weight = weight;
this.whiskerLength = whiskerLength;
totalFish++;
}
public Poodle () {
this("Bluu", 52.0, 720.0,5.0);
totalFish++;
}
public Catfish (Catfish other) {
this.name = other.name;
this.length
=
other.length;
this.weight = other.weight;
totalFish++;
}
public Boolean isShaggy () {
}
return whiskerLength > length;
}
public String toString () {
String shaggy = isShaggy()? "am" : "am not";
return String.format("I'm a talking fish named %s. My length is %s ft %s in and my weight is %s lbs %s oz." +
"I'm a catfish whose longest whisker is %d, so I am shaggy", name, formatLength(), formatWeight(),whiskerLength, shaggy);

Transcribed Image Text:public class Dog
// Variables
protected String name;
protected Double length;
protected Double weight;
protected static int totalDogs = 0;
// Constructors
public Dog (String name, Double length, Double weight) {
if(name == null || name.trim().isEmpty()) {
this.name = "Clifford";
} else {
}
this.name = name;
if(length == null || length.isNaN() || length.isInfinite() || length < 0) {
this.length
= 8.0;
} else {
this.length = length;
}
if(weight == null || weight.isNaN() || weight.isInfinite() || weight < 0) {
this.weight
= 2.0;
} else {
}
this.weight = weight;
totalFish++;
}
public Dog() {
this("Clifford", 5.0, 12.0);
//Methods
}
public Fish (Fish other) {
this.name = other.name;
this.length
this.weight
totalFish++;
other.length;
= other.weight;
public String formatLength() {
int feet = (int) (length / 12);
double inches = length % 12;
inches = (int) (inches * 100.0) / 100.0;
return String.format("%d ft %.2f in", feet, inches);
}
public String formatWeight() {
int pounds = (int) (weight / 16);
double ounces = weight % 16;
ounces = (int) (ounces * 100.0) / 100.0;
if (pounds
1) {
return String.format("%d lb %.2f oz", pounds, ounces);
} else {
return String.format("%d lbs %.2f oz", pounds, ounces);
}
}
public String toString() {
return String.format("I'm a talking Dog named %s. My length is %s and my weight is %s.".
', name, format Length(), formatWeight());
==
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
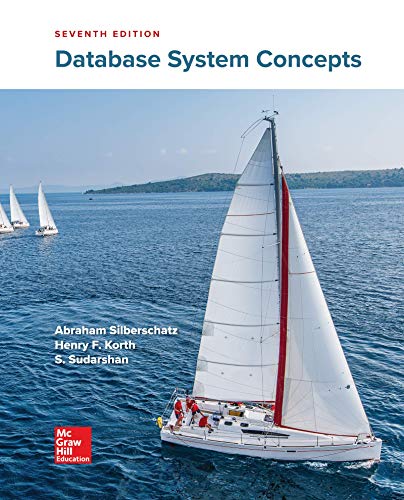
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
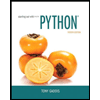
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
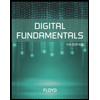
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
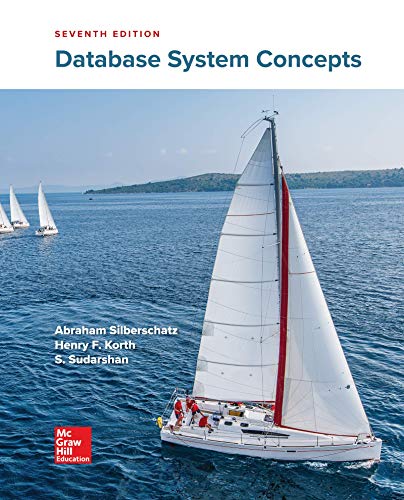
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
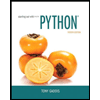
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
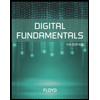
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
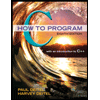
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
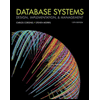
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
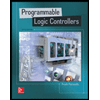
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education