can you help me to draw the flow chart for the java code: import java.util.Scanner; class BinoNode { int data; int numNodes; BinoNode arr[]; public BinoNode(int k) { data = -1; numNodes = k; arr = new BinoNode[numNodes]; } } class BinomialTree { private BinoNode root; private int order, size; public BinomialTree(int k) { size = 0; order = k; root = new BinoNode(order); createTree(root); } private void createTree(BinoNode r) { int n = r.numNodes; if (n == 0) { return; } for (int i = 0; i < n; i++) { r.arr[i] = new BinoNode(i); createTree(r.arr[i]); } } public void clear() { size = 0; root = new BinoNode(order); createTree(root); } public void insert(int val) { try { insert(root, val); } catch (Exception e) { } } private void insert(BinoNode r, int val) throws Exception { if (r.data == -1) { r.data = val; size++; throw new Exception("inserted !"); } int n = r.numNodes; for (int i = 0; i < n; i++) { insert(r.arr[i], val); } } public void printTree() { System.out.print("\nBinomial Tree = "); printTree(root); System.out.println(); } private void printTree(BinoNode r) { if (r.data != -1) { System.out.print(r.data + " "); } int n = r.numNodes; if (n == 0) { return; } for (int i = 0; i < n; i++) { printTree(r.arr[i]); } } } public class BinomialTreeProject { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("##----Binomial Tree----##"); System.out.println("\nEnter order of binomial tree:"); System.out.print("= "); BinomialTree binomialTree = new BinomialTree(scan.nextInt()); boolean quit = false; do { System.out.println("\n#---Binomial Tree Operations---#"); System.out.println("1. Insert "); System.out.println("2. Delete"); System.out.println("3. Search"); System.out.println("4. Print"); System.out.println("5. Quit"); System.out.print("Enter your option = "); int choice = scan.nextInt(); switch (choice) { case 1: System.out.println("Enter integer element to insert"); System.out.print("= "); binomialTree.insert(scan.nextInt()); break; case 2: binomialTree.clear(); System.out.println("\nTree Cleared\n"); break; case 3: System.out.println("TODO: Search"); break; case 4: System.out.print("\n-------------------->"); binomialTree.printTree(); System.out.println("-------------------->"); break; case 5: quit = true; System.out.println("\nThanks for using --Binomial Tree--\n"); break; default: System.out.println("Wrong Entry \n "); break; } } while (quit == false); } }
can you help me to draw the flow chart for the java code:
import java.util.Scanner;
class BinoNode {
int data;
int numNodes;
BinoNode arr[];
public BinoNode(int k) {
data = -1;
numNodes = k;
arr = new BinoNode[numNodes];
}
}
class BinomialTree {
private BinoNode root;
private int order, size;
public BinomialTree(int k) {
size = 0;
order = k;
root = new BinoNode(order);
createTree(root);
}
private void createTree(BinoNode r) {
int n = r.numNodes;
if (n == 0) {
return;
}
for (int i = 0; i < n; i++) {
r.arr[i] = new BinoNode(i);
createTree(r.arr[i]);
}
}
public void clear() {
size = 0;
root = new BinoNode(order);
createTree(root);
}
public void insert(int val) {
try {
insert(root, val);
} catch (Exception e) {
}
}
private void insert(BinoNode r, int val) throws Exception {
if (r.data == -1) {
r.data = val;
size++;
throw new Exception("inserted !");
}
int n = r.numNodes;
for (int i = 0; i < n; i++) {
insert(r.arr[i], val);
}
}
public void printTree() {
System.out.print("\nBinomial Tree = ");
printTree(root);
System.out.println();
}
private void printTree(BinoNode r) {
if (r.data != -1) {
System.out.print(r.data + " ");
}
int n = r.numNodes;
if (n == 0) {
return;
}
for (int i = 0; i < n; i++) {
printTree(r.arr[i]);
}
}
}
public class BinomialTreeProject {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.println("##----Binomial Tree----##");
System.out.println("\nEnter order of binomial tree:");
System.out.print("= ");
BinomialTree binomialTree = new BinomialTree(scan.nextInt());
boolean quit = false;
do {
System.out.println("\n#---Binomial Tree Operations---#");
System.out.println("1. Insert ");
System.out.println("2. Delete");
System.out.println("3. Search");
System.out.println("4. Print");
System.out.println("5. Quit");
System.out.print("Enter your option = ");
int choice = scan.nextInt();
switch (choice) {
case 1:
System.out.println("Enter integer element to insert");
System.out.print("= ");
binomialTree.insert(scan.nextInt());
break;
case 2:
binomialTree.clear();
System.out.println("\nTree Cleared\n");
break;
case 3:
System.out.println("TODO: Search");
break;
case 4:
System.out.print("\n-------------------->");
binomialTree.printTree();
System.out.println("-------------------->");
break;
case 5:
quit = true;
System.out.println("\nThanks for using --Binomial
Tree--\n");
break;
default:
System.out.println("Wrong Entry \n ");
break;
}
} while (quit == false);
}
}

Step by step
Solved in 2 steps with 2 images

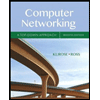
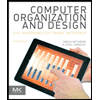
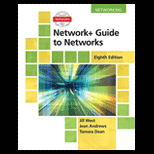
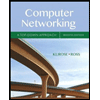
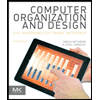
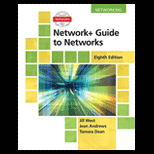
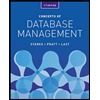
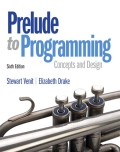
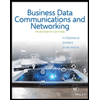