C PROGRAM!!!! How do I code the C code for the main.c file to accomplish the following tasks outlined in the assignment (see images) to produce a similar palindromes output? Given Code: dynarray.c file code #include "dynarray.h" const int DEFAULT_DYNARRAY_CAPACITY = 2; void dynarray_init(dynarray_t *a) { /* allocate space for data */ a->array = (data_t*)malloc(DEFAULT_DYNARRAY_CAPACITY * sizeof(data_t)); if (a->array == NULL) { fprintf(stderr, "ERROR: Cannot allocate memory for dynamic array."); exit(-1); } /* initialize other metadata */ a->capacity = DEFAULT_DYNARRAY_CAPACITY; a->size = 0; a->front = 0; } void dynarray_expand(dynarray_t *a, size_t new_capacity) { size_t i; /* allocate new array */ data_t *new_array = (data_t*)malloc(new_capacity * sizeof(data_t)); if (!new_array) { fprintf(stderr, "ERROR: Cannot allocate memory for dynamic array."); exit(-1); } /* copy items to new array */ for (i = 0; i < a->size; i++) { new_array[i] = a->array[(a->front + i) % a->capacity]; } /* delete old array and clean up */ free(a->array); a->array = new_array; a->capacity = new_capacity; a->front = 0; } size_t dynarray_size(dynarray_t *a) { return a->size; } size_t dynarray_is_empty(dynarray_t *a) { return dynarray.h file code typedef int data_t; typedef struct { data_t *array; size_t capacity; size_t size; size_t front; } dynarray_t; /* dynamic array operations */ void dynarray_init(dynarray_t *a); void dynarray_expand(dynarray_t *a, size_t capacity); size_t dynarray_size(dynarray_t *a); size_t dynarray_is_empty(dynarray_t *a); void dynarray_free(dynarray_t *a); /* stack operations */ void dynarray_push(dynarray_t *a, data_t item); data_t dynarray_pop(dynarray_t *a); data_t dynarray_top(dynarray_t *a); /* queue operations */ void dynarray_enqueue(dynarray_t *a, data_t item); data_t dynarray_dequeue(dynarray_t *a); data_t dynarray_front(dynarray_t *a); #endif lab5.c file code #include "lab5.h" slnode_t* malloc_slnode(data_t data) { slnode_t *node = (slnode_t*)malloc(sizeof(slnode_t)); if (node == NULL) { fprintf(stderr, "ERROR: Cannot allocate memory for link list node."); exit(EXIT_FAILURE); } node->data = data; node->next = NULL; return node; } void sllist_init(sllist_t *list) { list->head = NULL; list->size = 0; } void sllist_addfirst(sllist_t *list, data_t item) { slnode_t *new_node = malloc_slnode(item); if (list->head != NULL) { new_node->next = list->head;
C PROGRAM!!!!
How do I code the C code for the main.c file to accomplish the following tasks outlined in the assignment (see images) to produce a similar palindromes output?
Given Code:
dynarray.c file code
#include "dynarray.h"
const int DEFAULT_DYNARRAY_CAPACITY = 2;
void dynarray_init(dynarray_t *a) {
/* allocate space for data */
a->array = (data_t*)malloc(DEFAULT_DYNARRAY_CAPACITY * sizeof(data_t));
if (a->array == NULL) {
fprintf(stderr, "ERROR: Cannot allocate memory for dynamic array.");
exit(-1);
}
/* initialize other metadata */
a->capacity = DEFAULT_DYNARRAY_CAPACITY;
a->size = 0;
a->front = 0;
}
void dynarray_expand(dynarray_t *a, size_t new_capacity) {
size_t i;
/* allocate new array */
data_t *new_array = (data_t*)malloc(new_capacity * sizeof(data_t));
if (!new_array) {
fprintf(stderr, "ERROR: Cannot allocate memory for dynamic array.");
exit(-1);
}
/* copy items to new array */
for (i = 0; i < a->size; i++) {
new_array[i] = a->array[(a->front + i) % a->capacity];
}
/* delete old array and clean up */
free(a->array);
a->array = new_array;
a->capacity = new_capacity;
a->front = 0;
}
size_t dynarray_size(dynarray_t *a) {
return a->size;
}
size_t dynarray_is_empty(dynarray_t *a) {
return
dynarray.h file code
typedef int data_t;
typedef struct {
data_t *array;
size_t capacity;
size_t size;
size_t front;
} dynarray_t;
/* dynamic array operations */
void dynarray_init(dynarray_t *a);
void dynarray_expand(dynarray_t *a, size_t capacity);
size_t dynarray_size(dynarray_t *a);
size_t dynarray_is_empty(dynarray_t *a);
void dynarray_free(dynarray_t *a);
/* stack operations */
void dynarray_push(dynarray_t *a, data_t item);
data_t dynarray_pop(dynarray_t *a);
data_t dynarray_top(dynarray_t *a);
/* queue operations */
void dynarray_enqueue(dynarray_t *a, data_t item);
data_t dynarray_dequeue(dynarray_t *a);
data_t dynarray_front(dynarray_t *a);
#endif
lab5.c file code
#include "lab5.h"
slnode_t* malloc_slnode(data_t data) {
slnode_t *node = (slnode_t*)malloc(sizeof(slnode_t));
if (node == NULL) {
fprintf(stderr, "ERROR: Cannot allocate memory for link list node.");
exit(EXIT_FAILURE);
}
node->data = data;
node->next = NULL;
return node;
}
void sllist_init(sllist_t *list) {
list->head = NULL;
list->size = 0;
}
void sllist_addfirst(sllist_t *list, data_t item) {
slnode_t *new_node = malloc_slnode(item);
if (list->head != NULL) {
new_node->next = list->head;
}
list->head = new_node;
list->size++;
}
bool sllist_contains (sllist_t *list, data_t item) {
slnode_t *n;
// iterate over the linked list, looking for 'item'
for (n = list->head; n != NULL; n = n->next) {
if (n->data == item) {
return true;
}
}
return false;
}
size_t sllist_size(sllist_t *list) {
return list->size;
}
bool is_palindrome(char *text) {
return false;
}
size_t sllist_count (sllist_t *list, data_t item) {
return 0;
}
int sllist_find (sllist_t *list, data_t item) {
return -1;
}
void sllist_free (sllist_t *list) {
}
void sllist_addlast (sllist_t *list, data_t item) {
}
bool sllist_is_equal (sllist_t *list1, sllist_t *list2) {
return false;
}
void sllist_insert (sllist_t *list, data_t item, size_t index) {
}
void sllist_remove (sllist_t *list, data_t item) {}
lab5.h file code:
typedef struct slnode {
data_t data;
struct slnode *next;
} slnode_t;
/* * Singly-linked list definition.
typedef struct {
slnode_t *head;
size_t size;
} sllist_t;
PROVIDED FUNCTIONS
//Allocate a new singly-linked list node with the given data value.
slnode_t* malloc_slnode (data_t data);
//Initialize a singly-linked list
void sllist_init (sllist_t *list);
//Add an item at the head of the list.
void sllist_addfirst (sllist_t *list, data_t item);
//Returns true if there is an item equal to the given one in the list.
bool sllist_contains (sllist_t *list, data_t item);
//Returns the number of items currently in the list.
size_t sllist_size (sllist_t *list);
//Return true if the given string is a palindrome; false otherwise.
bool is_palindrome(char *text);
//Returns the number of items equal to the given one in the list.
size_t sllist_count (sllist_t *list, data_t item);
// Returns the index of the first item equal to the given item. If no such item is found, returns -1.
int sllist_find (sllist_t *list, data_t item);
//Clean up the list, freeing all of the list nodes.
void sllist_free (sllist_t *list);
//Add an item at the tail of the list.
void sllist_addlast (sllist_t *list, data_t item);
//Returns true if the two lists have the same number of items, and each pair of corresponding items are also equal; false otherwise.
bool sllist_is_equal (sllist_t *list1, sllist_t *list2);
//Adds an item at the given index in the list.
void sllist_insert (sllist_t *list, data_t item, size_t index);
//Removed the first item in the list that is equal to the given item. If no such item is found, no changes are made.
void sllist_remove (sllist_t *list, data_t item);
#endif
![## Palindromes
A palindrome is a string that is identical to itself when reversed. For example, "madam", "dad", and "abba" are palindromes. Note: the empty string is a palindrome, as is every string of length one.
### Instructions
Write some code in the `main()` function to accomplish the following tasks:
1. **Prompt for User Input**:
- Reuse code from Lab 3 to prompt the user to enter a string between 0 and 255 characters in length.
- Ignore whitespace and punctuation, and make all comparisons case-insensitive.
2. **Process the Input**:
- Store the entries from the keyboard in the queue ADT provided in the lab 5 code package.
3. **Palindrome Verification**:
- Use the `is_palindrome()` function ADT to implement a simple palindrome verification of the data entered. Here is the signature and documentation for the function:
```cpp
bool is_palindrome(char *text)
```
- Return true if the text is a palindrome, false otherwise.
### Submission
Turn in your commented program and a screenshot (script) of the execution run of the program. Include some sample tests in your captured code run. Examples of valid palindromes:
- ""
- "a"
- "aa"
- "aaa"
- "aba"
- "abba"
- "Taco cat"
- "Madam, I'm Adam"
- "A man, a plan, a canal: Panama"
- "Doc, note: I dissent. A fast never prevents a fatness. I diet on cod."
These examples highlight the variety of forms that palindromes can take, including those with punctuation and spaces which should be ignored when determining if a string is a palindrome.
### Notes
Ensure your code includes comments to explain key points and logic processes used. This helps in understanding the flow of the program and in maintaining the code in the future.
---
### Example Code
An example of how you might structure your `main()` function:
```cpp
#include <iostream>
#include <cstring>
#include <cctype>
bool is_palindrome(char *text);
int main() {
char input[256];
std::cout << "Enter a string (0-255 characters): ";
std::cin.getline(input, 256);
// Preprocess the input
int len = strlen(input);
char processed[](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8cb58778-70ba-4f7e-90ce-7267bd79a013%2F65c69035-89ce-4abc-893e-d324e1b944c4%2Fuea2c6t_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

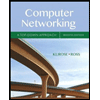
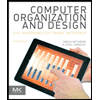
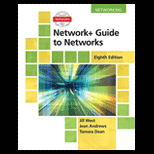
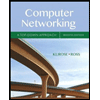
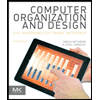
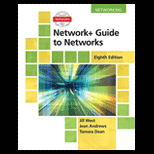
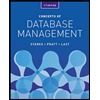
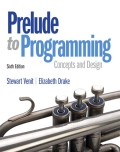
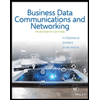