Task 2: Working with Constants, Variables and Arithmetic Operators Exercise 1: Bring in the file circlearea.cpp from the Lab 2 folder. The code of circlearea.cpp is as follows: // This program will output the circumference and area // of the circle with a given radius. // PLACE YOUR NAME HERE #include using namespace std; const double PI = 3.14; const double RADIUS = 5.4; int main() { area // definition of area of circle float circumference; // definition of circumference circumference = 2 * PI * RADIUS; // computes circumference area = ; // computes area // Fill in the code for the cout statement that will output (with description) // the circumference // Fill in the code for the cout statement that will output (with description) // the area of the circle return 0; } Exercise 2: Fill in the blanks and the cout statements so that the output will produce the following: Exercise 3: Change the data type of circumference from float to int. Run the program and record the results. The circumference of the circle is . The area of the circle is . Explain what happened to get the above results.
Task 2: Working with Constants, Variables and Arithmetic Operators
Exercise 1: Bring in the file circlearea.cpp from the Lab 2 folder.
The code of circlearea.cpp is as follows:
// This program will output the circumference and area
// of the circle with a given radius.
// PLACE YOUR NAME HERE
#include <iostream>
using namespace std;
const double PI = 3.14;
const double RADIUS = 5.4;
int main()
{
area // definition of area of circle
float circumference; // definition of circumference
circumference = 2 * PI * RADIUS; // computes circumference
area = ; // computes area
// Fill in the code for the cout statement that will output (with description)
// the circumference
// Fill in the code for the cout statement that will output (with description)
// the area of the circle
return 0;
}
Exercise 2: Fill in the blanks and the cout statements so that the output will
produce the following:
Exercise 3: Change the data type of circumference from float to int. Run the
program and record the results.
The circumference of the circle is .
The area of the circle is .
Explain what happened to get the above results.

Step by step
Solved in 3 steps with 2 images

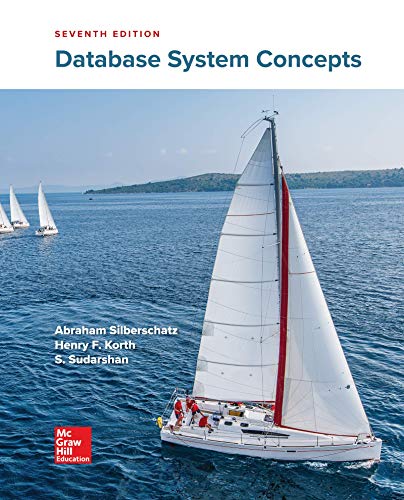
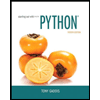
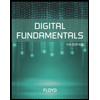
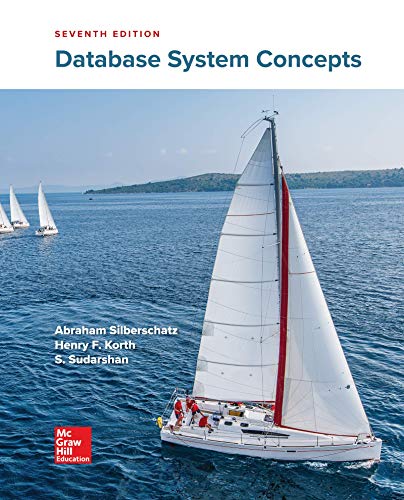
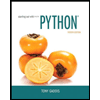
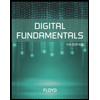
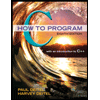
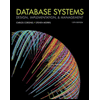
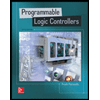