Please write a C++ program to check a password to see whether it is really secure. This program is like a password game. If the password is not secure, your program must report all its security violations. Please see the following test case #1 to design your application program. You must stop your program when the password from the user is "quit". In other words, “quit" cannot be a password in this game. You must write 7 functions to check the following 7 security rules for any given password. Rule 1: Length invalid – The length of the password must be 8 to 12 only. Rule 2: No Space – The password must not contain any space or blank character. Rule 3: At least 2 digits – The password must contain at least 2 digits. Rule 4: At least 1 upper-case letter – The password must contain at least one upper-case letter. Rule 5: At least 1 lower-case letter – The password must contain at least one lower-case letter. Rule 6: At least 1 special character – The password must contain at least one special character, which can be one of the following 7 choices: 'S', #', '@, '&', *', '?’, or '!'. Rule 7: No special numbers – The password must not contain any of the following 4 numbers: 2020, 2019, 2018, or 2017. Your test case #1 must look exactly as follows including the data. You must also do test case #2 and test case #3 with different sets of input data. Each test case must test at least 5 passwords with 2 secure and 3 non-secure. All those 7 violations must appear in those 3 non-secure passwords. Each test case or test run must begin with a welcome message, and must end with a thank-you message.
#include <string> // for string processing
#include <iostream> // Access input output stream: cin cout
using namespace std; // Access cout, endl, cin without using std:: as prefix
// 8 global variables and 7 prototypes of functions
string pw; // global pw for the password to be checked
bool r1, r2, r3, r4, r5, r6, r7; // global 7 boolean flags for violations
void s1(); void s2(); void s3(); void s4(); void s5();
void s6(); void s7(); // 7 prototypes of functions to be defined after main()
int main() // like a driver to call those 7 functions to check a password
{// begin of main // must return integer to the caller
cout << "Welcome to the PASSWORD game designed by Joel!" << endl;
cout << "Please enter a password:" << endl;
getline(cin, pw); // pw may have blanks anywhere, so must use getline( )
cout << "Your password \"" << pw << "\" " ;
while (pw != "quit") // the password is not “quit”
{ //begin of while loop
s1(); s2(); s3(); s4();
s5(); s6(); s7(); // call to check all 7 rules for satisfaction/violation
if (r1 && r2 && r3 && r4 && r5 && r6 && r7) // all 7 rules satisfied
cout << "is very secure! Congratulations!" << endl ;
else // insecure password
{ // begin print all the violated rules in detail ================.
cout << "violates the following rule(s):" << endl ; // You must complete all the following strings
if (!r1) cout << "Rule 1: Length invalid – The length of the password must ---
if (!r2) cout << "Rule 2: No Space – The password must not contain any space ---
if (!r3) cout << "Rule 3: At least 2 digits – The password must ---
if (!r4) cout << "Rule 4: At least 1 upper-case letter – The password must ---
if (!r5) cout << "Rule 5: At least 1 lower-case letter – The password must ---
if (!r6) cout << "Rule 6: At least 1 special character – The password must --
if (!r7) cout << "Rule 7: No special numbers – The password must not contain ---
} // end print all the violated rules in detail =====================.
cout << "Please enter a password:" << endl;
getline(cin, pw); // pw may have blanks anywhere, so must use getline( )
cout << "Your password \"" << pw << "\" ";
} // end of while loop ==============================================.
cout << "is to quit the game." << endl;
cout << "Thank you for playing the PASSWORD game designed by Joel!";
return 0 ; // return integer 0 to show the job is well done now.
} // end of main =========================================================.



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

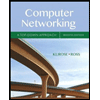
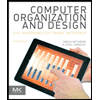
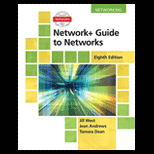
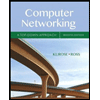
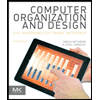
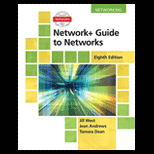
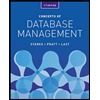
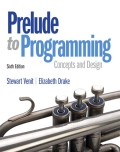
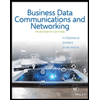