C+++ I need help filling in missing/needed code to to do the following: Read two doubles as the forceApplied and the contactArea of a BallObject object. Declare and assign pointer myBallObject with a new BallObject object using the forceApplied and the contactArea as arguments in that order. Then call myBallObject's IncreaseForceAppliedAndContactArea() member function. Ex: If the input is 3.0 2.5, then the output is: #include #include using namespace std; class BallObject { public: BallObject(double forceAppliedValue, double contactAreaValue); void IncreaseForceAppliedAndContactArea(); void Print(); private: double forceApplied; double contactArea; }; BallObject::BallObject(double forceAppliedValue, double contactAreaValue) { forceApplied = forceAppliedValue; contactArea = contactAreaValue; } void BallObject::IncreaseForceAppliedAndContactArea() { forceApplied = forceApplied * 5.0; contactArea = contactArea * 5.0; cout << "BallObject's forceApplied and contactArea are increased." << endl; } void BallObject::Print() { cout << "BallObject's forceApplied: " << fixed << setprecision(1) << forceApplied << endl; cout << "BallObject's contactArea: " << fixed << setprecision(1) << contactArea << endl; } int main() { /* Additional variable declarations go here */ /* Your code goes here */ myBallObject->Print(); return 0; BallObject's forceApplied and contactArea are increased. BallObject's forceApplied: 15.0 BallObject's contactArea: 12.5
C+++
I need help filling in missing/needed code to to do the following:
Read two doubles as the forceApplied and the contactArea of a BallObject object. Declare and assign pointer myBallObject with a new BallObject object using the forceApplied and the contactArea as arguments in that order. Then call myBallObject's IncreaseForceAppliedAndContactArea() member function.
Ex: If the input is 3.0 2.5, then the output is:
#include <iostream>
#include <iomanip>
using namespace std;
class BallObject {
public:
BallObject(double forceAppliedValue, double contactAreaValue);
void IncreaseForceAppliedAndContactArea();
void Print();
private:
double forceApplied;
double contactArea;
};
BallObject::BallObject(double forceAppliedValue, double contactAreaValue) {
forceApplied = forceAppliedValue;
contactArea = contactAreaValue;
}
void BallObject::IncreaseForceAppliedAndContactArea() {
forceApplied = forceApplied * 5.0;
contactArea = contactArea * 5.0;
cout << "BallObject's forceApplied and contactArea are increased." << endl;
}
void BallObject::Print() {
cout << "BallObject's forceApplied: " << fixed << setprecision(1) << forceApplied << endl;
cout << "BallObject's contactArea: " << fixed << setprecision(1) << contactArea << endl;
}
int main() {
/* Additional variable declarations go here */
/* Your code goes here */
myBallObject->Print();
return 0;
BallObject's forceApplied and contactArea are increased. BallObject's forceApplied: 15.0 BallObject's contactArea: 12.5

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

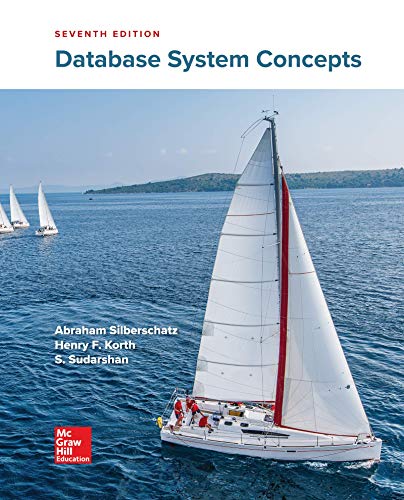
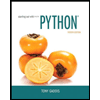
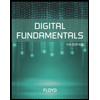
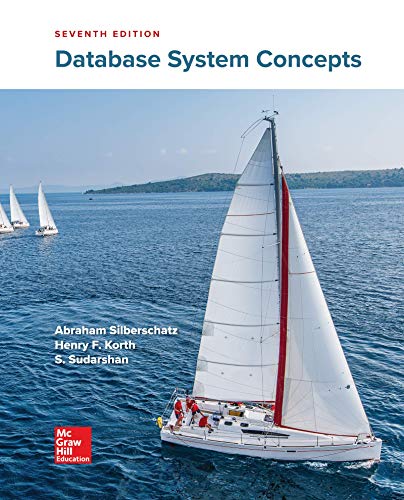
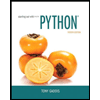
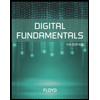
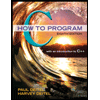
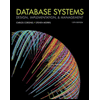
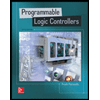