C++ use shared pointers to objects, create vectors of shared pointers to objects and pass these as parameters. You need to write one line of code. Declare circleTwoPtr. Watch for **** in comments.
m6 lb
Dont copy previous answers they are incorrect. Please provide a typed code and not pictures. The template was given below the code. we are just making changes to main cpp. We are writing one line of code based on the comments.
C++
use shared pointers to objects, create
You need to write one line of code. Declare circleTwoPtr. Watch for **** in comments.
Template code is given make changes and add stuff based on comments:
Template given:
main.cpp:
#include<iostream>
#include "Circle.h"
#include <fstream>
#include <vector>
#include <memory>
#include<cstdlib>
#include <fstream>
#include <sstream>
using namespace std;
const int SIZE = 10;
// pay attention to this parameter list
int inputData(vector<shared_ptr<Circle>> &circlePointerArray, string filename)
{
ifstream inputFile(filename);
istringstream instream;
string data;
int count =0;
int x,y,radius;
try{
if (inputFile){
while (!inputFile.eof() && count <SIZE){
getline(inputFile,data);
istringstream instream(data);
instream>>x;
instream>>y;
instream>>radius;
//create a new Circle object and push it into
//the vector
shared_ptr<Circle> circle = make_shared<Circle>(x,y,radius);
circlePointerArray.push_back(circle);
count++;
}
}
else throw string("File Not Found");
}
catch (string message){
cout<<message<<endl;
exit(0);
}
return count;
}
int main(){
shared_ptr<Circle> circleOnePtr = make_shared<Circle>(0,0,5);
// create a new shared pointer to a Circle Object
//*************** create another shared pointer called circleTwoPtr
//
if (circleOnePtr->greaterThan(circleTwoPtr))
cout<<" Circle One is bigger "<<endl;
else
cout<<" Circle Two is bigger "<<endl;
// declare a vector of shared pointers to Circle objects
std::vector<shared_ptr<Circle>>circlePointerVector;
int count = inputData(circlePointerVector, "dataLab4.txt");
cout<<"The total number of circles is "<<count<<endl;
cout<<"They are :"<<endl;
for (int i=0;i<count;i++)
cout<<circlePointerVector[i]->toString()<<endl;
double sumOfAreas=0;
for (int i=0;i<count;i++)
sumOfAreas+=circlePointerVector[i]->getArea();
cout<<"The total sum of the areas is "<<sumOfAreas<<endl;
shared_ptr <Circle>tmpPtr=make_shared<Circle>();
circlePointerVector[1]=circlePointerVector[3];
cout<<"The modified array is "<<endl;
for (int i=0;i<count;i++)
cout<<circlePointerVector[i]->toString()<<endl;
return 0;
}
Circle.cpp:
//#include<string>
//#include <cstdlib>// no need for this as is in header
#include "Circle.h"
#include <cmath>
#include <iostream>
Circle::Circle(){
//if you have regular constructor you must
//define default constructor
//C++ will not auto create for you
x=0;
y=0;
radius=1;
count++;
}
Circle::Circle(int xcoord,int ycoord, int r){
x=xcoord;
y=ycoord;
radius=r;
count++;
}
int Circle::getX(){
return x;
}
int Circle::getY(){
return y;
}
int Circle::getRadius(){
return radius;
}
int Circle::getCount(){
return count;
}
void Circle::setX(int xcoord){
x=xcoord;
}
void Circle::setY(int xcoord){
x=xcoord;
}
void Circle::setRadius(int r){
radius=r;
}
double Circle::getArea(){
return M_PI*pow(radius,2);
}
double Circle::getCircumference(){
return M_PI*radius/2;
}
double Circle::getDistance(Circle other){
return sqrt(pow(x-other.getX(),2)+pow((y-other.getY()),2));
}
bool Circle::intersects(Circle other){
return (this->getDistance(other)<=radius);
}
void Circle::resize(double scale){
radius = scale*radius;
}
Circle Circle::resize(int scale){
Circle c(x,y,radius*scale);
return c;
}
string Circle::toString(){
return "(" +to_string(x)+","+to_string(y)+"):"+to_string(radius);
}
// *****note the change to this parameter
// make suitable changes where need to other instance methods
bool Circle::greaterThan(shared_ptr<Circle> other){
return radius>other->getRadius();
}
Circle::~Circle(){
//std::cout<<"Inside Destructor "<<endl;
//if you have dynamically allocated memory
//you must release/delete it here
}
int Circle::count=0;
Circle.h:
#include<string>
#include <vector>
#include <memory>
using namespace std;
#ifndef CIRCLE_H// this is optional
#define CIRCLE_H // works with or without it
class Circle{
//private
static int count;
int radius;
int x,y;
public:
Circle();
Circle(int xcoord,int ycoord, int r);
int getY();
int getX();
int getRadius();
static int getCount();
double getArea();
double getCircumference();
double getDistance(Circle other);
bool intersects(Circle other);
Circle resize(int scale);//to copy self to other;
void resize(double scale);//for self
void setX(int xcoord);
void setY(int xcoord);
void setRadius(int r);
bool greaterThan(shared_ptr<Circle> other);
string toString();
~Circle();
//regular functions
//void inputData(vector<Circle>);
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

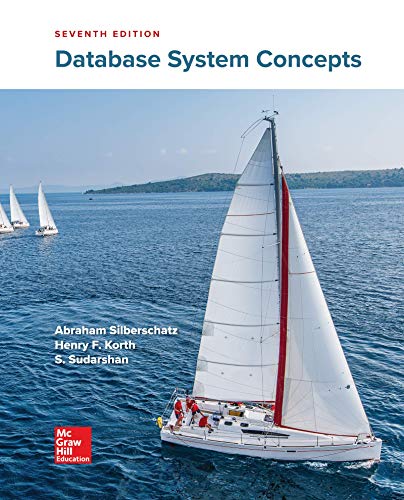
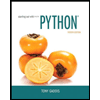
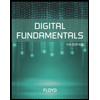
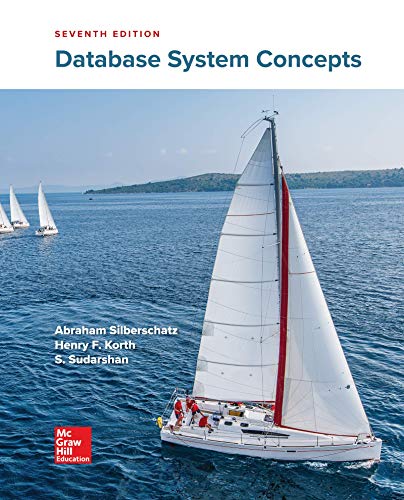
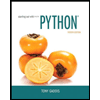
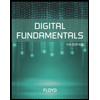
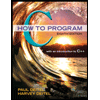
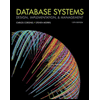
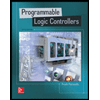