use Advanced C++ techniques, containers and features to refactor the C/C++ algorithm. The purpose is to replace appropriate declarations and code segments with Advanced C++ declarations and code. * Replace all arrays with appropriate STL containers. * Use STL algorithms to REPLACE existing logic where appropriate. * Use smart and move pointers where pointers are needed. * Use lambda expressions where appropriate. * Use C++ style casting when needed. * Look for opportunities where tuples could be used. * The code listing is in C and C++. If there are logic or syntax problems, please fix. # define ASCII 256 int algorithm1(int a, int b) { return (a > b) ? a : b; } void algorithm2(char* str, int size, int badchar[ASCII]) { int i; for (i = 0; i < ASCII; i++) badchar[i] = -1; for (i = 0; i < size; i++) badchar[(int) str[i]] = i; } void algorithm3(char *txt, char *pat) { int m = strlen(pat); int n = strlen(txt); int badchar[NO_OF_CHARS]; algorithm2(pat, m, badchar); int s = 0; while (s <= (n - m)) { int j = m - 1; while (j >= 0 && pat[j] == txt[s + j]) j--; if (j < 0) { s += (s + m < n) ? m - badchar[txt[s + m]] : 1; } else s += algorithm1(1, j - badchar[txt[s + j]]); } }
use Advanced C++ techniques, containers and features to refactor the C/C++
replace appropriate declarations and code segments with Advanced C++ declarations and code.
* Replace all arrays with appropriate STL containers.
* Use STL algorithms to REPLACE existing logic where appropriate.
* Use smart and move pointers where pointers are needed.
* Use lambda expressions where appropriate.
* Use C++ style casting when needed.
* Look for opportunities where tuples could be used.
* The code listing is in C and C++.
If there are logic or syntax problems, please fix.
# define ASCII 256
int algorithm1(int a, int b)
{
return (a > b) ? a : b;
}
void algorithm2(char* str, int size, int badchar[ASCII])
{
int i;
for (i = 0; i < ASCII; i++)
badchar[i] = -1;
for (i = 0; i < size; i++)
badchar[(int) str[i]] = i;
}
void algorithm3(char *txt, char *pat)
{
int m = strlen(pat);
int n = strlen(txt);
int badchar[NO_OF_CHARS];
algorithm2(pat, m, badchar);
int s = 0;
while (s <= (n - m))
{
int j = m - 1;
while (j >= 0 && pat[j] == txt[s + j])
j--;
if (j < 0)
{
s += (s + m < n) ? m - badchar[txt[s + m]] : 1;
}
else
s += algorithm1(1, j - badchar[txt[s + j]]);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

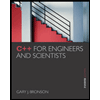
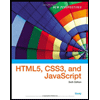
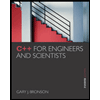
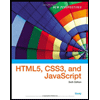