INSTRUCTIONS: In this assignment, you will make your own smart pointer type. You will write the following class to develop a referenced counted smart pointer. You will also implement a few other member functions to resemble the functionality of an ordinary raw pointer. Basically, this is a problem of designing a single, non-trivial class and overloading a few pointer related operators. You may not use any of the STLs except for stdexcept, to do this, i.e., please dont try and use a shared_ptr to implement the class. You can start with this code, and you may add other member functions, if you want. C++ LANGUAGE
INSTRUCTIONS: In this assignment, you will make your own smart pointer type. You will write the following class to develop a referenced counted smart pointer. You will also implement a few other member functions to resemble the functionality of an ordinary raw pointer. Basically, this is a problem of designing a single, non-trivial class and overloading a few pointer related operators. You may not use any of the STLs except for stdexcept, to do this, i.e., please dont try and use a shared_ptr to implement the class. You can start with this code, and you may add other member functions, if you want. C++ LANGUAGE
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
INSTRUCTIONS: In this assignment, you will make your own smart pointer type. You will write the following class to develop a referenced counted smart pointer. You will also implement a few other member functions to resemble the functionality of an ordinary raw pointer. Basically, this is a problem of designing a single, non-trivial class and overloading a few pointer related operators. You may not use any of the STLs except for stdexcept, to do this, i.e., please dont try and use a shared_ptr to implement the class. You can start with this code, and you may add other member functions, if you want.
C++ LANGUAGE
Only need smart_ptr.h file.

Transcribed Image Text:```cpp
template <typename T>
class smart_ptr {
public:
smart_ptr();
// Create a smart_ptr that is initialized to nullptr. The
// reference count should be initialized to nullptr.
explicit smart_ptr(T* raw_ptr);
// Create a smart_ptr that is initialized to raw_ptr. The
// reference count should be one. Make sure it points to
// the same pointer as the raw_ptr.
smart_ptr(const smart_ptr& rhs);
// Copy construct a pointer from rhs. The reference count
// should be incremented by one.
smart_ptr(smart_ptr&& rhs);
// Move construct a pointer from rhs.
smart_ptr& operator=(const smart_ptr& rhs);
// This assignment should make a shallow copy of the
// right-hand side's pointer data. The reference count
// should be incremented as appropriate.
smart_ptr& operator=(smart_ptr&& rhs);
// This move assignment should steal the right-hand side's
// pointer data.
bool clone();
// If the smart_ptr is either nullptr or has a reference
// count of one, this function will do nothing and return
// false. Otherwise, the referred to object's reference
// count will be decreased and a new deep copy of the
// object will be created. This new copy will be the
// object that this smart_ptr points and its reference
// count will be one.
int ref_count() const;
// Returns the reference count of the pointed to data.
T& operator*();
// The dereference operator shall return a reference to
// the referred object. Throws null_ptr_exception on
// invalid access.
T* operator->();
// The arrow operator shall return the pointer ptr_.
// Throws null_ptr_exception on invalid access.
~smart_ptr(); // deallocate all dynamic memory
private:
T* ptr_; // pointer to the referred object
int* ref_; // pointer to a reference count
};
```
This code defines a template class `smart_ptr` intended to manage memory safely using a reference counting mechanism. It includes constructors for initializing the smart pointer, operators for copying, moving, and dereferencing, and a function to clone the underlying object if needed. The class also manages the deallocation of the dynamic memory upon destruction.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
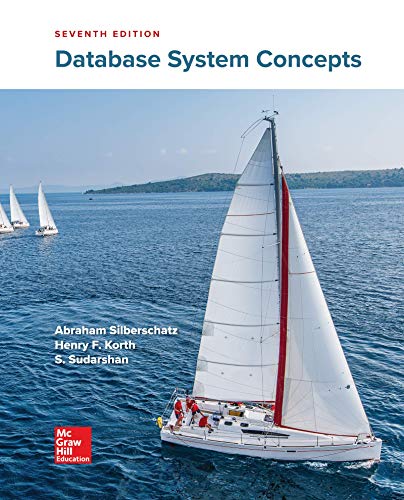
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
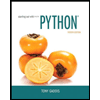
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
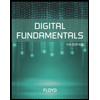
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
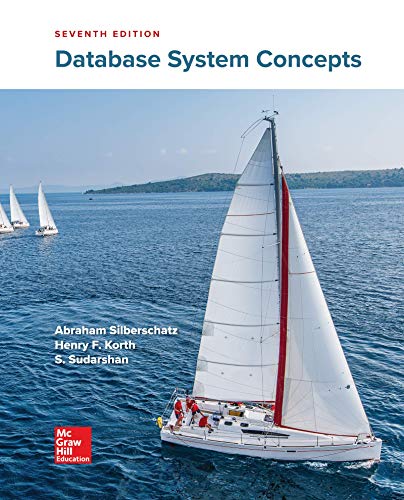
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
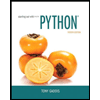
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
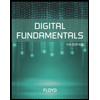
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
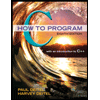
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
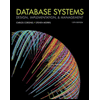
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
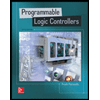
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education