Please solve in C++ You are given the following files: main.cpp Classes.h Classes.cpp The current implementation outputs: Shape Colour: Red Shape Area: 0 Shape Colour: Blue Shape Area: 0 Change the code to use polymorphism and virtual functions to enable overriding of the Area function of the base class Shape to use the Area function of derived class Circle or Rectangle depending on the type of the object. The code should output: Shape Colour: Red Shape Area: 78.5397 Shape Colour: Blue Shape Area: 24 main.cpp #include #include #include "Classes.h" int main() { std::string red = "Red"; std::string blue = "Blue"; Circle circle(red, 5.0); Rectangle rectangle(blue, 4.0, 6.0); std::vector shapes; shapes.push_back(&circle); shapes.push_back(&rectangle); for (Shape* shape : shapes) { std::cout << "Shape Colour: " << shape->GetColour() << std::endl; std::cout << "Shape Area: " << shape->Area() << std::endl; } return EXIT_SUCCESS; } Classes.h #ifndef CLASSES_H #define CLASSES_H #include class Shape { protected: std::string colour; public: Shape(std::string &c); std::string GetColour(); double Area(); }; class Circle : public Shape { public: Circle(std::string &c, double r); double Area();\ private: double radius; }; class Rectangle : public Shape { private: double width; double height; public: Rectangle(std::string &c, double w, double h); double Area(); }; #endif Classes.cpp #include "Classes.h" Shape::Shape(std::string &c) : colour(c) {} double Shape::Area() { return 0.0; } std::string Shape::GetColour() { return colour; } Circle::Circle(std::string &c, double r) : Shape(c), radius(r) {} double Circle::Area() { return 3.14159 * radius * radius; } Rectangle::Rectangle(std::string &c, double w, double h) : Shape(c), width(w), height(h) {} double Rectangle::Area() { return width * height; }
Please solve in C++
You are given the following files:
- main.cpp
- Classes.h
- Classes.cpp
The current implementation outputs:
Shape Colour: Red Shape Area: 0 Shape Colour: Blue Shape Area: 0
Change the code to use polymorphism and virtual functions to enable overriding of the Area function of the base class Shape to use the Area function of derived class Circle or Rectangle depending on the type of the object. The code should output:
Shape Colour: Red Shape Area: 78.5397 Shape Colour: Blue Shape Area: 24
main.cpp
#include <iostream>
#include <
#include "Classes.h"
int main() {
std::string red = "Red";
std::string blue = "Blue";
Circle circle(red, 5.0);
Rectangle rectangle(blue, 4.0, 6.0);
std::vector<Shape*> shapes;
shapes.push_back(&circle);
shapes.push_back(&rectangle);
for (Shape* shape : shapes) {
std::cout << "Shape Colour: " << shape->GetColour() << std::endl;
std::cout << "Shape Area: " << shape->Area() << std::endl;
}
return EXIT_SUCCESS;
}
Classes.h
#ifndef CLASSES_H
#define CLASSES_H
#include <string>
class Shape
{
protected:
std::string colour;
public:
Shape(std::string &c);
std::string GetColour();
double Area();
};
class Circle : public Shape
{
public:
Circle(std::string &c, double r);
double Area();\
private:
double radius;
};
class Rectangle : public Shape
{
private:
double width;
double height;
public:
Rectangle(std::string &c, double w, double h);
double Area();
};
#endif
Classes.cpp
#include "Classes.h"
Shape::Shape(std::string &c) : colour(c) {}
double Shape::Area()
{
return 0.0;
}
std::string Shape::GetColour()
{
return colour;
}
Circle::Circle(std::string &c, double r) : Shape(c), radius(r) {}
double Circle::Area()
{
return 3.14159 * radius * radius;
}
Rectangle::Rectangle(std::string &c, double w, double h) : Shape(c), width(w), height(h) {}
double Rectangle::Area()
{
return width * height;
}

Step by step
Solved in 4 steps with 1 images

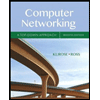
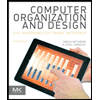
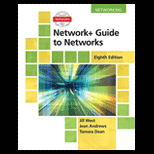
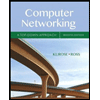
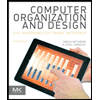
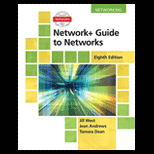
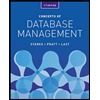
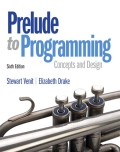
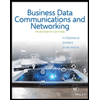