BST.Java import java.util.NoSuchElementException; /** * Your implementation of a BST. */ public class BST> { /* * Do not add new instance variables or modify existing ones. */ private BSTNode root; private int size; /* * Do not add a constructor. */ /** * Adds the data to the tree. * * This must be done recursively. * * The new data should become a leaf in the tree. * * Traverse the tree to find the appropriate location. If the data is * already in the tree, then nothing should be done (the duplicate * shouldn't get added, and size should not be incremented). * * Should be O(log n) for best and average cases and O(n) for worst case. * * @param data The data to add to the tree. * @throws java.lang.IllegalArgumentException If data is null. */ public void add(T data) { // WRITE YOUR CODE HERE (DO NOT MODIFY METHOD HEADER)! } /** * Removes and returns the data from the tree matching the given parameter. * * This must be done recursively. * * There are 3 cases to consider: * 1: The node containing the data is a leaf (no children). In this case, * simply remove it. * 2: The node containing the data has one child. In this case, simply * replace it with its child. * 3: The node containing the data has 2 children. Use the SUCCESSOR to * replace the data. You should use recursion to find and remove the * successor (you will likely need an additional helper method to * handle this case efficiently). * * Do NOT return the same data that was passed in. Return the data that * was stored in the tree. * * Hint: Should you use value equality or reference equality? * * Must be O(log n) for best and average cases and O(n) for worst case. * * @param data The data to remove. * @return The data that was removed. * @throws java.lang.IllegalArgumentException If data is null. * @throws java.util.NoSuchElementException If the data is not in the tree. */ public T remove(T data) { // WRITE YOUR CODE HERE (DO NOT MODIFY METHOD HEADER)! } /** * Returns the root of the tree. * * For grading purposes only. You shouldn't need to use this method since * you have direct access to the variable. * * @return The root of the tree */ public BSTNode getRoot() { // DO NOT MODIFY THIS METHOD! return root; } /** * Returns the size of the tree. * * For grading purposes only. You shouldn't need to use this method since * you have direct access to the variable. * * @return The size of the tree */ public int size() { // DO NOT MODIFY THIS METHOD! return size; } }
Provided Code:
BST.Java
import java.util.NoSuchElementException;
/**
* Your implementation of a BST.
*/
public class BST<T extends Comparable<? super T>> {
/*
* Do not add new instance variables or modify existing ones.
*/
private BSTNode<T> root;
private int size;
/*
* Do not add a constructor.
*/
/**
* Adds the data to the tree.
*
* This must be done recursively.
*
* The new data should become a leaf in the tree.
*
* Traverse the tree to find the appropriate location. If the data is
* already in the tree, then nothing should be done (the duplicate
* shouldn't get added, and size should not be incremented).
*
* Should be O(log n) for best and average cases and O(n) for worst case.
*
* @param data The data to add to the tree.
* @throws java.lang.IllegalArgumentException If data is null.
*/
public void add(T data) {
// WRITE YOUR CODE HERE (DO NOT MODIFY METHOD HEADER)!
}
/**
* Removes and returns the data from the tree matching the given parameter.
*
* This must be done recursively.
*
* There are 3 cases to consider:
* 1: The node containing the data is a leaf (no children). In this case,
* simply remove it.
* 2: The node containing the data has one child. In this case, simply
* replace it with its child.
* 3: The node containing the data has 2 children. Use the SUCCESSOR to
* replace the data. You should use recursion to find and remove the
* successor (you will likely need an additional helper method to
* handle this case efficiently).
*
* Do NOT return the same data that was passed in. Return the data that
* was stored in the tree.
*
* Hint: Should you use value equality or reference equality?
*
* Must be O(log n) for best and average cases and O(n) for worst case.
*
* @param data The data to remove.
* @return The data that was removed.
* @throws java.lang.IllegalArgumentException If data is null.
* @throws java.util.NoSuchElementException If the data is not in the tree.
*/
public T remove(T data) {
// WRITE YOUR CODE HERE (DO NOT MODIFY METHOD HEADER)!
}
/**
* Returns the root of the tree.
*
* For grading purposes only. You shouldn't need to use this method since
* you have direct access to the variable.
*
* @return The root of the tree
*/
public BSTNode<T> getRoot() {
// DO NOT MODIFY THIS METHOD!
return root;
}
/**
* Returns the size of the tree.
*
* For grading purposes only. You shouldn't need to use this method since
* you have direct access to the variable.
*
* @return The size of the tree
*/
public int size() {
// DO NOT MODIFY THIS METHOD!
return size;
}
}
BSTNode.Java
/**
* Node class used for implementing the BST.
*
* DO NOT MODIFY THIS FILE!!
*
* @author CS 1332 TAs
* @version 1.0
*/
public class BSTNode<T extends Comparable<? super T>> {
private T data;
private BSTNode<T> left;
private BSTNode<T> right;
/**
* Constructs a BSTNode with the given data.
*
* @param data the data stored in the new node
*/
BSTNode(T data) {
this.data = data;
}
/**
* Gets the data.
*
* @return the data
*/
T getData() {
return data;
}
/**
* Gets the left child.
*
* @return the left child
*/
BSTNode<T> getLeft() {
return left;
}
/**
* Gets the right child.
*
* @return the right child
*/
BSTNode<T> getRight() {
return right;
}
/**
* Sets the data.
*
* @param data the new data
*/
void setData(T data) {
this.data = data;
}
/**
* Sets the left child.
*
* @param left the new left child
*/
void setLeft(BSTNode<T> left) {
this.left = left;
}
/**
* Sets the right child.
*
* @param right the new right child
*/
void setRight(BSTNode<T> right) {
this.right = right;
}
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

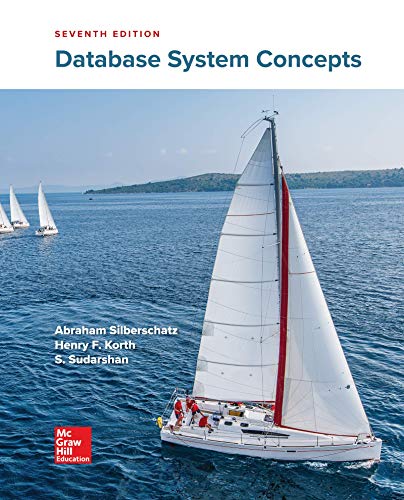
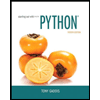
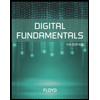
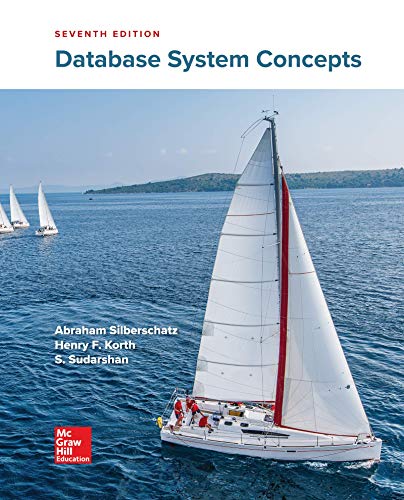
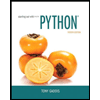
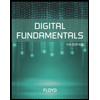
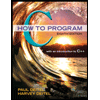
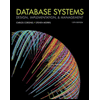
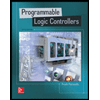