Java programming I have to do the "Resize method" which I believe if the array is full, it should resize so there is space.
Java programming
I have to do the "Resize method" which I believe if the array is full, it should resize so there is space.
Ill paste the code below:
package circularQueue;
/*
* Design the class that would implement the interfaceiQueue.
*/
public class cirQueue <T> implements iQueue <T> {
//Array of T objects
private T[] Ar; //reference to array of objects
//int size keeps track of how many elements in the queue
int size = 0;
//first the index to the head of the queue
int first = -1;
//last the index the last element of the queue
int last = -1;
public cirQueue() {
Ar = (T[]) new Object[5];
size = 0;
first = last = -1;
}
public int size() {
return size;
}
public boolean empty() {
return (size == 0);
}
private void resize() {
//HW TODO
}
//inserts element e at the back of the queue
public void insert(T e) {
if(this.empty()) {
first = last = 0;
//insert e at indx 0;
Ar[first] = e;
size = 1;
return;
}
if(size == Ar.length) {
//resize HW complete this one.
this.resize();
}
//increase last by one, make sure it circulate back to 0 if it reaches capacity-1
last = (last + 1) % Ar.length;
//insert e at index last
Ar[last] = e;
//increase the size
size++;
}
public T remove() {
if(this.empty()) {
System.out.println("Error empty queue");
return null;
}
T save = Ar[first];
first = (first + 1) % Ar.length;
size--;
return save;
}
public String toString() {
if(this.empty())
return "[]";
String st = "[";
//build your string [-1, -3, -9]
int temp = first;
for(int i = 0; i < size-1; i++) {
//in the loop your are always dealing with element i
//v element i is Ar[i]
//append Ar[i], to the string
st += Ar[temp].toString() + ", ";
temp = (temp + 1) % Ar.length;
}
//close the bracket
st += Ar[temp].toString() + "]";
return st;
}
}
![import java.util.ArrayList;I
public class Tester {
public static void main(String [] args) {
Stack<String> st = new Stack<String>();
st.push("st");
st.push ("sfsf");
st.push("hi");
System.out.println("size:
System.out.println("removing:
System.out.println("size:
System.out.println("the top is: " + st.peek ());
System.out.println("size: " + st.size()+
+ st.size() +
+ st.pop());
+ st.size()+
St:
+ st.toString());
%3D
" St: " + st.toString());
St:
+ st.toString());
Queue<Integer> Q = new LinkedList<Integer>();
Q.add(-4);
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F33eba9e5-eb7d-44a1-93e3-ed0ee897e17f%2F3473cca1-3b98-4f9d-a978-c23da3b9a0de%2Fkjk14xh_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

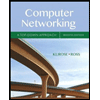
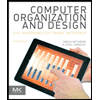
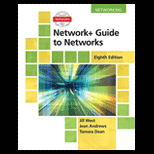
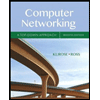
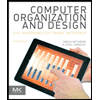
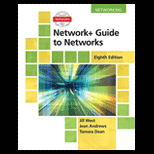
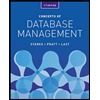
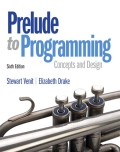
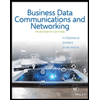